1、初识面向对象
1)面向对象(Object-Oriented Programing,OOP)
2)本质
-
3)三大特性
-
4)认识类和对象
认识角度:先有对象后有类,对象是具体的事物,类,是抽象的,是对对象的抽象
-
2、方法加固和加深
1)方法的定义
修饰符
- 返回类型
- break和return
- 方法名
- 参数列表
- 异常抛出 ```java package com.oop;
import java.io.IOException;
public class Demo01 { public static void main(String[] args) { / 修饰符 返回值类型 方法名(…){ 方法体 return } / int [] arrays = {1,2,3,4,5}; printArrays(34,3,3,4,5,90,54); }
public static void printArrays(int... arrays){
for (int item:
arrays) {
System.out.print(item + "\t");
}
}
// 异常
public static void readFile(String file) throws IOException{
}
}
<a name="tRVir"></a>
#### 2) 方法的调用
<a name="jsPtD"></a>
#### 2.1)静态方法
- 可以直接通过类名调用方法
- 类创建后立即存在
<a name="jTl3g"></a>
#### 2.2)非静态方法
- 实例化这个类 new,实例调用
- 实例化后才会存在
```java
//可以调用
public static void a(){
b();
}
public static void b(){
}
-------------------
public static void a(){
b();
}
public static void b(){
}
------------------
public void a(){
b();
}
public static void b(){
}
// 不可用调用
public static void a(){
b();
}
public void b(){
}
- 形参和实参
- 值传递和引用传递 ```java package com.oop;
// 值传递 public class Demo03 { public static void main(String[] args) { int a = 1; System.out.println(“origin a:” + a);//1 Demo03 demo03 = new Demo03(); demo03.change(a);//1 } public void change(int a){ a = 10; } }
#
// 引用传递 package com.oop;
public class Demo04 { public static void main(String[] args) { Person person = new Person(); System.out.println(“orgin name: “ + person.name);//null
Demo04 demo04 = new Demo04();
demo04.change(person);
System.out.println("call after: " + person.name);//秦将
}
public void change(Person person){
person.name = "秦将";
}
}
class Person{ String name; }
- this关键字
<a name="YVOBH"></a>
### 3、对象的创建分析
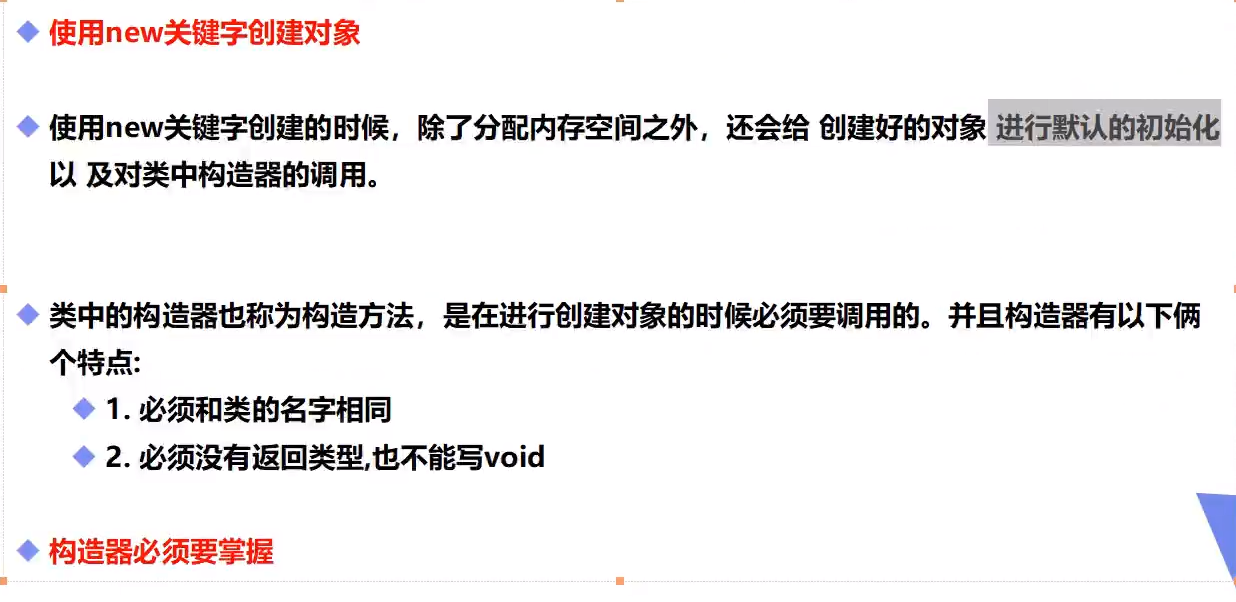<br />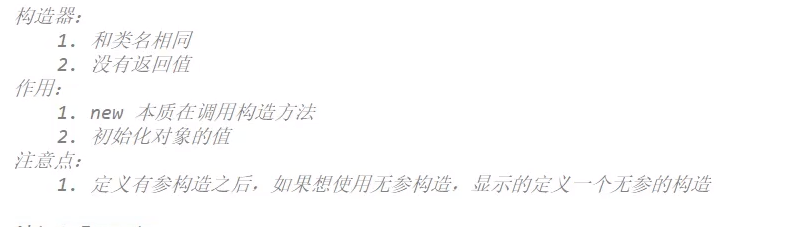
<a name="hUxjP"></a>
#### 1)示例代码
```java
// Person
package com.oop;
public class Person {
//1、 一个类什么也不用写,也会存在一个构造方法
// 实例化初始值
// 2、使用new关键字,本质在调用构造器
// 有参构造,一旦定义有参函数,无参必须显示定义
String name;
public Person(String name) {
this.name = name;
}
}
// Student
package com.oop;
public class Student {
public static void main(String[] args) {
Person person = new Person("请讲");
System.out.println(person.name);
}
}
2) 创建对象内存分析
// application
package com.oop;
public class Application {
public static void main(String[] args) {
Pet dog = new Pet();
dog.name = "旺财";
dog.age = 11;
dog.shout();
Pet cat = new Pet();
}
}
//pet
package com.oop;
public class Pet {
public String name;
public int age;
public Pet() {
}
public void shout(){
System.out.println("交了一声");
}
}
3) 总结
4、面向对象三大特性
1)封装
1.1)作用
- 快捷键 构建构造函数 alt+insert+shfit
- 提高程序的安全性
- 隐藏代码的实现细节
- 统一接口
- 系统可维护增加 ```java //app package com.oop;
public class Application { public static void main(String[] args) { Pet dog = new Pet(); dog.setAge(300); int age = dog.getAge(); System.out.println(age);
}
} //pet package com.oop;
public class Pet { private String name; private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (age < 0 || age >130){
this.age = 1;
}else {
this.age = age;
}
}
}
<a name="RcMwy"></a>
#### 2)继承
- **定义**
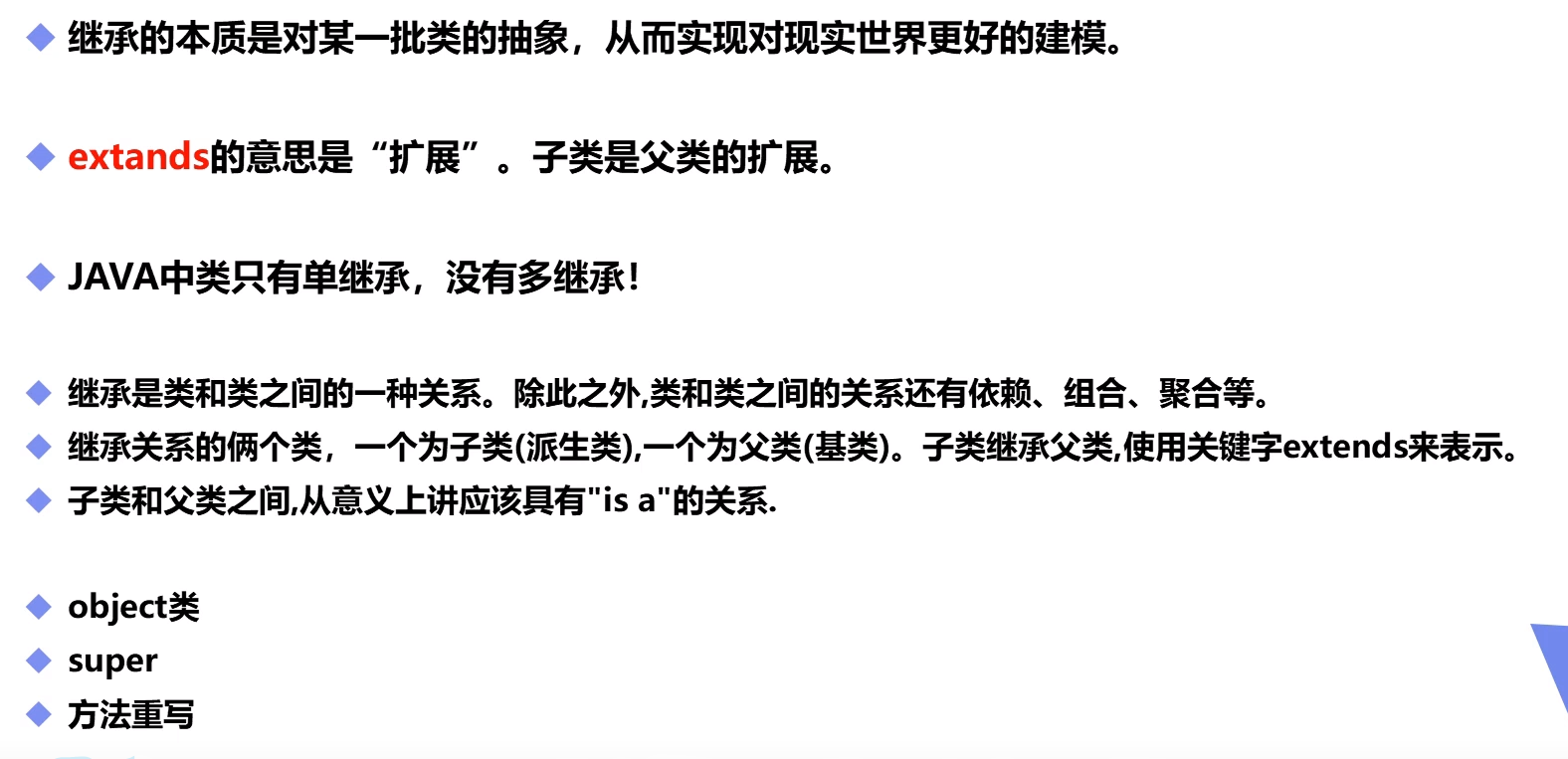
- 在java中默认继承object类
- 查看继承关系,快捷键 ctrl + H
```java
//app
package com.oop;
public class Application {
public static void main(String[] args) {
Student student = new Student();
student.say();
}
}
//person
package com.oop;
public class Person {
//1、 一个类什么也不用写,也会存在一个构造方法
// 实例化初始值
// 2、使用new关键字,本质在调用构造器
// 有参构造,一旦定义有参函数,无参必须显示定义
public Person() {
}
String name;
public Person(String name) {
this.name = name;
}
public void say(){
System.out.println("我开始讲了一句话");
}
}
// student
package com.oop;
public class Student extends Person{
public static void main(String[] args) {
}
}
2.1)super()
- 特别注意点
- 调用子类构造器,会默认调用父类构造器,先执行子类然后父类
- 子类构造器中显示写super().必须放在构造函数代码中的第一行
- 如果父类是有参,子类要调用父类构造函数,必须显示调用且有参
- super和this不能同时调用构造方法!
- VS this
- 代表的对象不同
- this 本身调用这个对象
- super 代表父类对象的引用
- 前提
- this 没继承也能使用
- super只有在继承条件中使用
- 构造方法
- this 本类的构造
- super 父类的构造
- 代表的对象不同
2.2)方法重写
- 重写是对方法的重写,与属性无关
- 要求
- 需要有继承关系
- 方法名必须相同
- 参数列表必须相同
- 修饰符:范围可以扩大 public > protected > default >private
- 抛出异常:范围可以缩小,不能被扩大 ClassNotFoundException -> Exception
- 必须都是非静态方法,因为静态方法在类创建后便会存在
- 为什么要重写
- 父类的功能,子类不一定需要,或者不一定满足
- ALT+Insert: Override
- 代码示例 ```java // Student package com.oop;
public class Student extends Person{ public static void main(String[] args) { }
@Override // 注解
public void say() {
System.out.println("我只是个学生而已");
}
public Student() {
// 隐式调用 super()
this.say();
super.say();
System.out.println("我现在还是个学生");
}
} // Person package com.oop;
public class Person { //1、 一个类什么也不用写,也会存在一个构造方法 // 实例化初始值 // 2、使用new关键字,本质在调用构造器 // 有参构造,一旦定义有参函数,无参必须显示定义 public Person() { System.out.println(“我是你爸爸”); }
String name;
public Person(String name) {
this.name = name;
}
public void say(){
System.out.println("你爸爸开始讲了一句话");
}
} // app package com.oop;
public class Application { public static void main(String[] args) { Student student = new Student(); student.say(); } }
<a name="swFWj"></a>
#### 3)多态
<a name="QtzsD"></a>
#### 3.1)定义
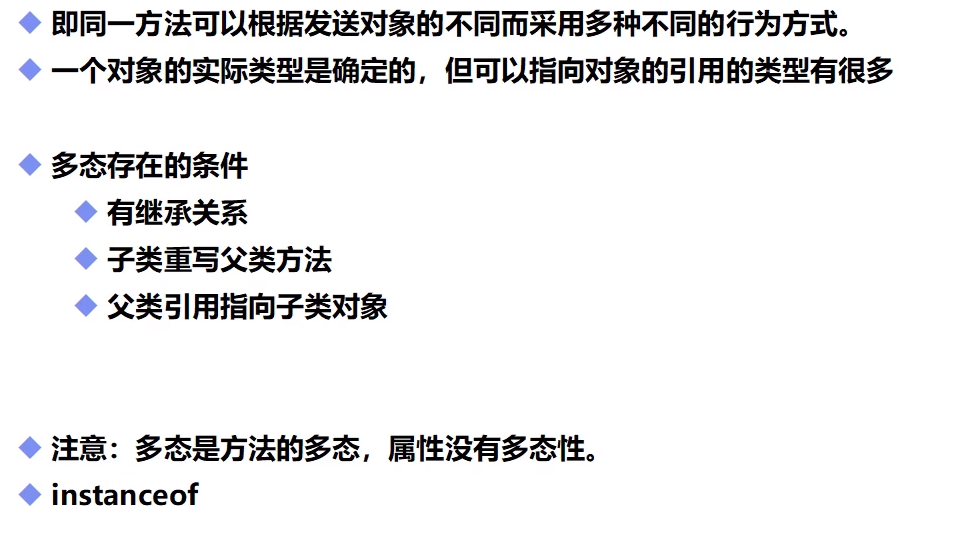
<a name="JWDV7"></a>
#### 3.2)多态注意事项
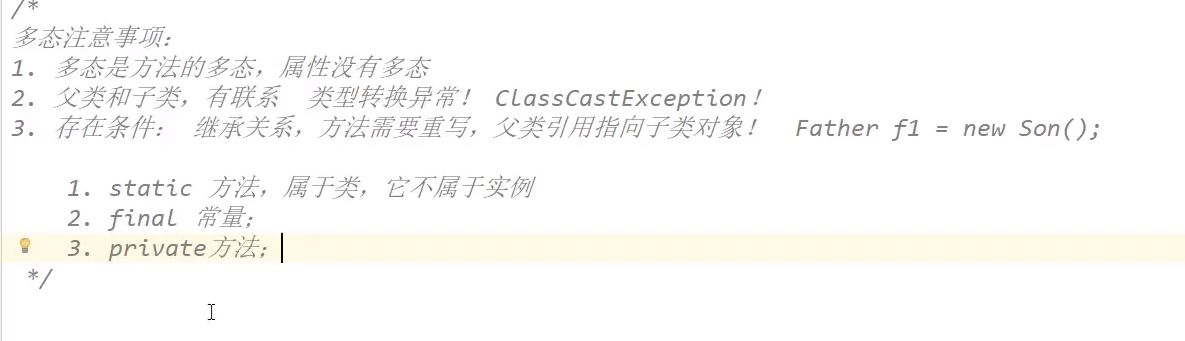
- 父类型指向子类,但不能调用子类方法
- 实例可以执行的方法,与左边类型有关
- 重写子类方法,执行子类方法
<a name="l8hoX"></a>
#### 3.3)代码示例
```java
//student
package com.oop;
public class Student extends Person{
@Override
public void run() {
System.out.println("son");
}
public void read(){
System.out.println("read");
}
}
// person
package com.oop;
public class Person {
public void run(){
System.out.println("run");
}
public void say(){
System.out.println("say");
}
}
//appication
package com.oop;
public class Application {
public static void main(String[] args) {
Student student = new Student();
Person student1 = new Student();// 父类型指向子类,但不能调用子类方法
student.run();// 实例可以执行的方法,与左边类型有关
student1.run();// 重写子类方法,执行子类方法
((Student)student1).read();
}
}
4)instanceof
- 编译是否通过取决于 两个类型间是否有直接关系,而非间接关系 ```java package com.oop;
public class Application { public static void main(String[] args) {
//Object > Person > Student
//Object > Person > Teacher
//Object > String
Student student = new Student();
System.out.println(student instanceof Person);//true
System.out.println(student instanceof Object);//true
System.out.println(student instanceof Student);//true
//System.out.println(student instanceof Teacher); 编译报错 非继承关系
//System.out.println(student instanceof String); 编译报错,非继承关系
System.out.println("#####################################");
Person p = new Student();
System.out.println(p instanceof Person);//true
System.out.println(p instanceof Object);//true
System.out.println(p instanceof Student);//true
System.out.println(p instanceof Teacher);//false
// System.out.println(p instanceof String); 编译报错,非继承关系
System.out.println("#####################################");
Object o = new Student();
System.out.println(o instanceof Person);//true
System.out.println(o instanceof Object);//true
System.out.println(o instanceof Student);//true
System.out.println(o instanceof Teacher);//false
System.out.println(o instanceof String); //false
}
}
<a name="Wik1e"></a>
#### 5)类型转化
- 调用结果 以右边类型方法为主
```java
package com.oop;
public class Application {
public static void main(String[] args) {
// 高类型调用低类型方法 需要强制转换
Person p1 = new Student();
((Student)p1).read();
// 低类型调用高类型方法 可能会丢失自己的一些方法
Student student = new Student();
Person p2 = student;// son run
//p2.read();//编译报错
p2.run();
}
}
5.1)总结
6)static 关键字
public class Person { public static int age; public double name;
{
System.out.println("匿名代码块");
}
static {
System.out.println("静态代码块");
}
public Person() {
System.out.println("构造方法");
}
public static void main(String[] args) {
// System.out.println(age); // System.out.println(name); 编译报错,不能调用非静态方法 Person person = new Person(); System.out.println(“################################”); Person person1 = new Person();
}
}
#
静态代码块 匿名代码块 构造方法
#
匿名代码块 构造方法
<a name="CiPqZ"></a>
#### 6.2)导入静态包
```java
package com.oop;
import static java.lang.Math.random;
import static java.lang.Math.PI;
public class Person {
public static void main(String[] args) {
System.out.println(random());
System.out.println(PI);
}
}
5、抽象类和接口
5.1 定义
- 接口可以多继承,插座可以插什么头
- 思考题?
- 抽象类不能new对象,那么存在构造器吗? ```java //Student package com.oop.Demo07;
public abstract class Student {
public abstract void doSomething();
public void run(){
System.out.println("Student run!");
}
} //application package com.oop.Demo07;
public class Application extends Student{ @Override public void doSomething() { System.out.println(“该做些什么呢?”); }
public static void main(String[] args) {
Application application = new Application();
application.doSomething();
application.run();
}
}
<a name="TBRxQ"></a>
#### 5.2 接口
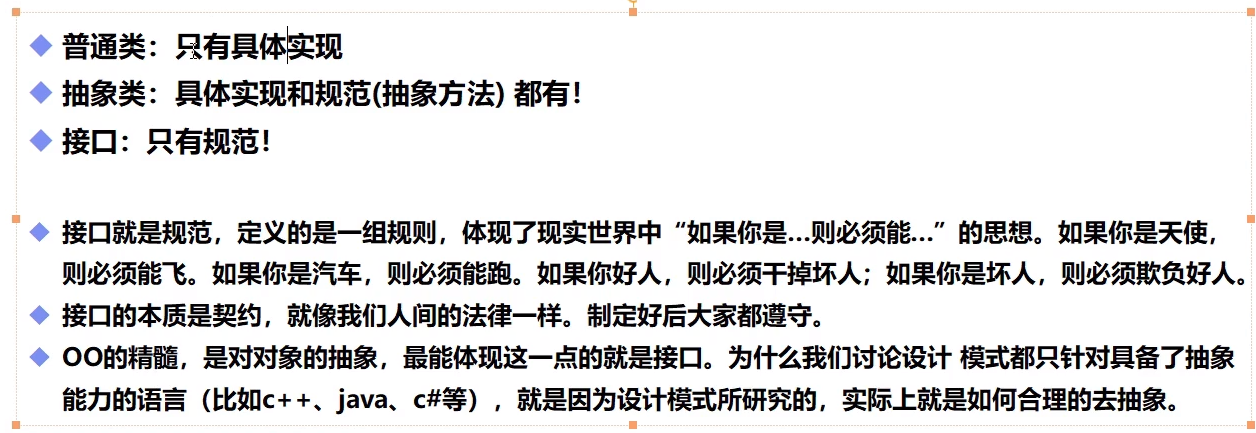
- **接口的作用**
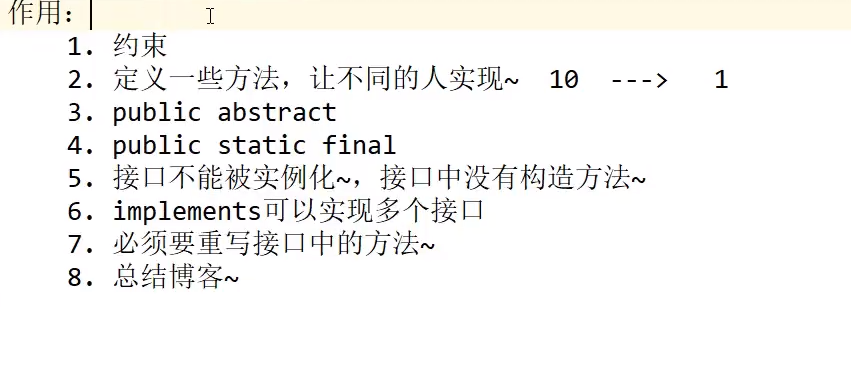
```java
// UserService
package com.oop.Demo08;
public interface UserService {
void addUser();
void deleteUser();
void editUser();
void queryUser();
}
// UserImpl
package com.oop.Demo08;
public class UserImpl implements UserService{
@Override
public void addUser() {
System.out.println("添加用户");
}
@Override
public void deleteUser() {
System.out.println("删除用户");
}
@Override
public void editUser() {
System.out.println("编辑用户");
}
@Override
public void queryUser() {
System.out.println("查询用户");
}
}
6、内部类和OOP实战
6.1 内部类
- 一个java文件可以有多个class文件,但只能有一个public class类 ```java //成员内部类 package com.oop.Demo09;
public class Outer { private int id = 10; public void out(){ System.out.println(“这是外部类的方法”); }
class Inner{
public void in(){
System.out.println("这是内部类的方法: "+id);
}
}
}
package com.oop.Demo09;
public class Appication { public static void main(String[] args) { Outer outer = new Outer(); // 1.通过外部类实例化内部类 Outer.Inner inner = outer.new Inner(); inner.in(); // 2.访问内部类 私有属性
}
}
//静态内部类 内部类加上static即可
//局部内部类 package com.oop.Demo09;
public class Outer { public void method(){ // 局部内部类 class Inner{ public void in(){ System.out.println(“局部内部类”);
}
}
}
}
class A{
} //匿名内部类
没有名字初始化类,不用将实例保存到变量中
package com.oop.Demo09;
public class Outer { public static void main(String[] args) { new UserService() { @Override public void eat() {
}
}
}
}
interface UserService{ void eat(); } ```