描述
内置生成透明背景图逻辑
脚本
// 启发语录列表
const textList = [
'选我所爱,爱我所选。',
'让改变适合你现在的生活和需要,而不是让你的生活和需要围绕着改变去进行。',
'此生理想、近期规划、今日功课。',
'流水不争先,争的是滔滔不绝。',
'既往不恋,当下不杂,未来不迎。',
];
/*-------上面是配置区域-------*/
const fmLocal = FileManager.local();
const basePath = fmLocal.documentsDirectory();
const bgImgPath = basePath + "bgImg";
const fixedIndexPath = basePath + "fixedIndex";
// 创建小组件
const widget = new ListWidget();
if (config.runsInWidget) {
createWidget();
return;
}
let selectResult = await confirm("???", ["设置", "换一句", "忽略"]);
if (selectResult === 0) {
let selectResult = await confirm("设置什么?", [
"背景图",
"固定文本",
"忽略",
]);
if (selectResult === 0) {
let selectResult = await confirm("请选择", [
"选择背景图",
"默认背景图",
"透明背景图",
"忽略",
]);
if (selectResult === 0) {
let img = await Photos.fromLibrary();
fmLocal.writeImage(bgImgPath, img);
widget.backgroundImage = img;
}
if (selectResult === 1) {
const isExistBg = fmLocal.fileExists(bgImgPath);
isExistBg && fmLocal.remove(bgImgPath);
}
if (selectResult === 2) {
let message =
"以下是【透明背景】生成步骤,如果你没有屏幕截图请退出,并返回主屏幕长按进入编辑模式。滑动到最右边的空白页截图。然后重新运行!";
let exitOptions = ["继续(已有截图)", "退出(没有截图)"];
let shouldExit = await confirm(message, exitOptions);
if (shouldExit) return;
let img = await Photos.fromLibrary();
let height = img.size.height;
let phone = phoneSizes()[height];
if (!phone) {
message =
"您似乎选择了非iPhone屏幕截图的图像,或者不支持您的iPhone。请使用其他图像再试一次!";
await confirm(message, ["好的!我现在去截图"]);
return;
}
let crop = { w: phone.中号, h: phone.小号, x: phone.左边, y: phone.顶部 };
// Crop image and finalize the widget.
let imgCrop = cropImage(img, new Rect(crop.x, crop.y, crop.w, crop.h));
message = "您的小部件背景已准备就绪,退出到桌面预览。";
const resultOptions = ["好的"];
await confirm(message, resultOptions);
fmLocal.writeImage(bgImgPath, imgCrop);
}
}
if (selectResult === 1) {
let index = await confirm(
"请选择",
textList.map((item) => item.replace(/\s/gi, ""))
);
fmLocal.writeString(fixedIndexPath, String(index));
}
}
if (selectResult === 1) {
const isExistFixedIndex = fmLocal.fileExists(fixedIndexPath);
isExistFixedIndex && fmLocal.remove(fixedIndexPath);
}
createWidget();
// widget.presentMedium();
// 创建桌面小组件
function createWidget() {
const isExistFixedIndex = fmLocal.fileExists(fixedIndexPath);
let textItem;
if (isExistFixedIndex) {
const index = fmLocal.readString(fixedIndexPath);
textItem = textList[Number(index)];
} else {
const index = Math.floor(Math.random() * textList.length);
textItem = textList[index];
}
const bgImg = fmLocal.readImage(bgImgPath);
if (!bgImg) {
const gradient = new LinearGradient();
gradient.locations = [0, 1];
gradient.colors = [new Color("#333333"), new Color("#111111")];
widget.backgroundGradient = gradient;
} else {
const bgImg = fmLocal.readImage(bgImgPath);
widget.backgroundImage = bgImg;
}
let text;
// 如果文本内容过长,通过给前后加换行实现文本自适应缩小的目的
if (textItem.length >= 30) {
textItem = `\n${textItem}\n`;
}
// 设置语录样式
text = widget.addText(textItem);
text.textColor = new Color("#ffffff");
text.font = new Font("Georgia-BoldItalic", 26);
text.minimumScaleFactor = 0.5;
textItem.length > 12 ? text.leftAlignText() : text.centerAlignText();
Script.setWidget(widget);
}
// 选择框
async function confirm(message, options) {
let alert = new Alert();
alert.message = message;
for (const option of options) {
alert.addAction(option);
}
return await alert.presentAlert();
}
// Pixel sizes and positions for widgets on all supported phones.
function phoneSizes() {
let phones = {
"2340": {
// 12mini
小号: 436,
中号: 936,
大号: 980,
左边: 72,
右边: 570,
顶部: 212,
中间: 756,
底部: 1300,
},
"2532": {
// 12/12 Pro
小号: 472,
中号: 1012,
大号: 1058,
左边: 78,
右边: 618,
顶部: 230,
中间: 818,
底部: 1408,
},
"2778": {
// 12 Pro Max
小号: 518,
中号: 1114,
大号: 1162,
左边: 86,
右边: 678,
顶部: 252,
中间: 898,
底部: 1544,
},
"2688": {
小号: 507,
中号: 1080,
大号: 1137,
左边: 81,
右边: 654,
顶部: 228,
中间: 858,
底部: 1488,
},
"1792": {
小号: 338,
中号: 720,
大号: 758,
左边: 54,
右边: 436,
顶部: 160,
中间: 580,
底部: 1000,
},
"2436": {
小号: 465,
中号: 987,
大号: 1035,
左边: 69,
右边: 591,
顶部: 213,
中间: 783,
底部: 1353,
},
"2208": {
小号: 471,
中号: 1044,
大号: 1071,
左边: 99,
右边: 672,
顶部: 114,
中间: 696,
底部: 1278,
},
"1334": {
小号: 296,
中号: 642,
大号: 648,
左边: 54,
右边: 400,
顶部: 60,
中间: 412,
底部: 764,
},
"1136": {
小号: 282,
中号: 584,
大号: 622,
左边: 30,
右边: 332,
顶部: 59,
中间: 399,
底部: 399,
},
};
return phones;
}
// Crop an image into the specified rect.
function cropImage(img, rect) {
let draw = new DrawContext();
draw.size = new Size(rect.width, rect.height);
draw.drawImageAtPoint(img, new Point(-rect.x, -rect.y));
return draw.getImage();
}
效果图
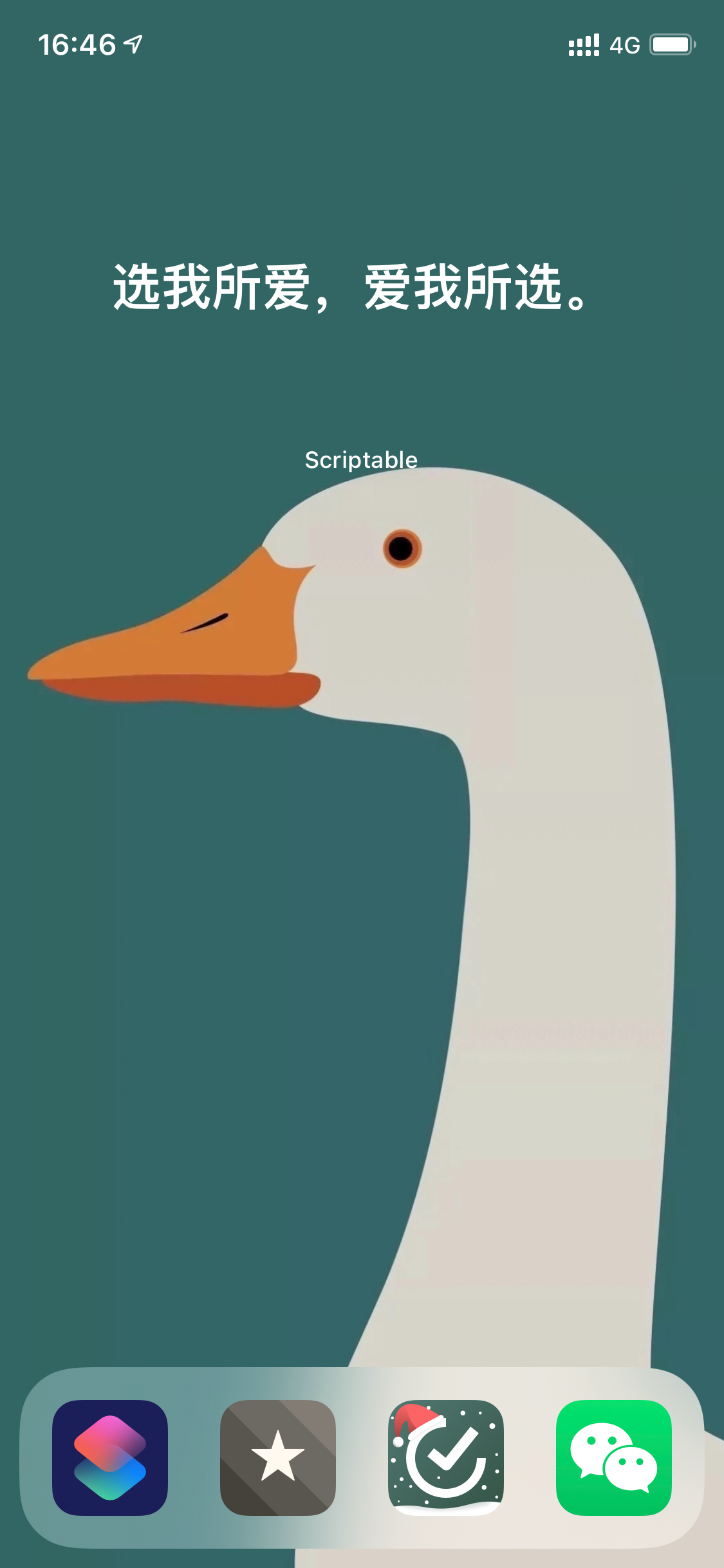