一、实例要求
- Http 协议是无状态的,浏览器和服务器间的请求响应一次,下一次会重新创建连接。
- 要求:实现基于 WebSocket 的长连接的全双工的交互。
- 改变 Http 协议多次请求的约束,实现长连接了,服务器可以发送消息给浏览器。
- 客户端浏览器和服务器端会相互感知,比如服务器关闭了,浏览器会感知,同样浏览器关闭了,服务器会感知。
-
二、代码演示
MyServer
public class MyServer {
public static void main(String[] args) throws InterruptedException {
// 创建两个线程
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
NioEventLoopGroup workerGroup = new NioEventLoopGroup(3);
try {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.handler(new LoggingHandler(LogLevel.INFO))// 在 bossGroup 增加一个日志处理器
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 因为基于 http 协议,使用 http 的编码和解码器
pipeline.addLast(new HttpServerCodec());
// 是以块方式写,添加 ChunkedWriteHandler 处理器
pipeline.addLast(new ChunkedWriteHandler());
/**
* 说明
* 1、http 数据在传输过程中是分段,HttpObjectAggregator,就是可以将多个段聚合起来
* 2、这就是为什么,当浏览器发送大量数据时,就会发出多次http请求
*/
pipeline.addLast(new HttpObjectAggregator(8192));
/**
* 说明
* 1、对应 websocket,它的数据是以 帧(frame)形式传递
* 2、可以看到 WebSocketFrame,下面有六个子类
* 3、浏览器请求时 ws://localhost:6699/hello 表示请求的url
* 4、WebSocketServerProtocolHandler 核心功能是将 Http 协议 升级成 ws 协议,保持长连接
* 5、是通过一个状态码 101
*/
pipeline.addLast(new WebSocketServerProtocolHandler("/hello"));
// 自定义handler,处理业务逻辑
pipeline.addLast(new MyTextWebsocketFrameHandler());
}
});
// 启动服务器
ChannelFuture channelFuture = serverBootstrap.bind(6699).sync();
channelFuture.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
MyTextWebsocketFrameHandler
public class MyTextWebsocketFrameHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
System.out.println("服务器端收到消息 " + msg.text());
// 回复
ctx.channel().writeAndFlush(new TextWebSocketFrame("服务器时间 " + LocalDateTime.now() + " " + msg.text()));
}
/**
* 当 web 客户端连接后,触发方法
*
* @param ctx
* @throws Exception
*/
@Override
public void handlerAdded(ChannelHandlerContext ctx) throws Exception {
// id 表示唯一的值,LongText 是唯一的 ShortText 不是唯一
System.out.println("handlerAdded 被调用" + ctx.channel().id().asLongText());
System.out.println("handlerAdded 被调用" + ctx.channel().id().asShortText());
}
@Override
public void handlerRemoved(ChannelHandlerContext ctx) throws Exception {
System.out.println("handlerRemoved 被调用" + ctx.channel().id().asLongText());
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
System.out.println("异常发生 " + cause.getMessage());
ctx.close(); // 关闭
}
}
hellp.html
```java <!DOCTYPE html>
<a name="T1HaP"></a>
# 三、演示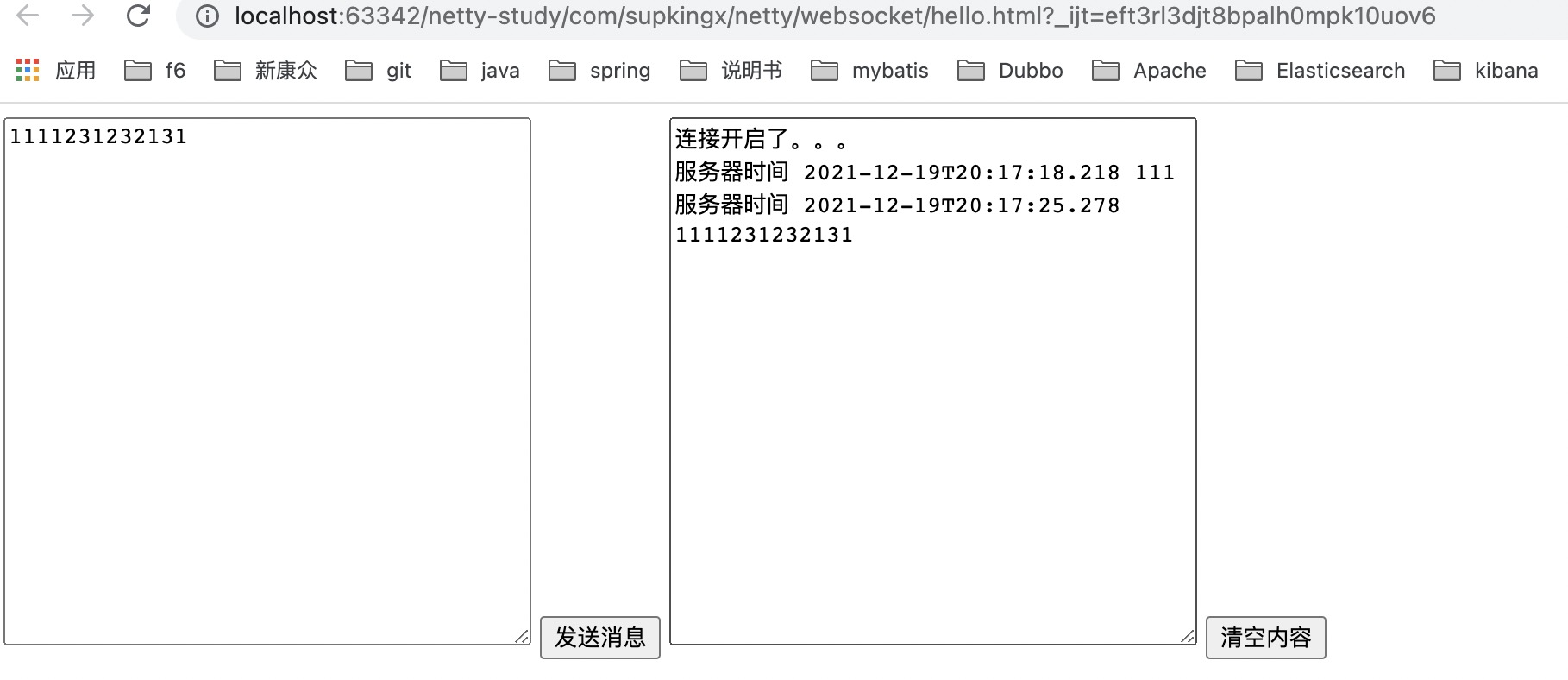
<a name="s4RYl"></a>
# 四、分析
关注以下代码段,netty 通过 WebSocketServerProtocolHandler 将 http 协议连接升级到 Websocket 协议。
```java
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 因为基于 http 协议,使用 http 的编码和解码器
pipeline.addLast(new HttpServerCodec());
// 是以块方式写,添加 ChunkedWriteHandler 处理器
pipeline.addLast(new ChunkedWriteHandler());
/**
* 说明
* 1、http 数据在传输过程中是分段,HttpObjectAggregator,就是可以将多个段聚合起来
* 2、这就是为什么,当浏览器发送大量数据时,就会发出多次http请求
*/
pipeline.addLast(new HttpObjectAggregator(8192));
/**
* 说明
* 1、对应 websocket,它的数据是以 帧(frame)形式传递
* 2、可以看到 WebSocketFrame,下面有六个子类
* 3、浏览器请求时 ws://localhost:6699/hello 表示请求的url
* 4、WebSocketServerProtocolHandler 核心功能是将 Http 协议 升级成 ws 协议,保持长连接
* 5、是通过一个状态码 101
*/
pipeline.addLast(new WebSocketServerProtocolHandler("/hello"));
// 自定义handler,处理业务逻辑
pipeline.addLast(new MyTextWebsocketFrameHandler());
}
});