maven依赖
<!--jar包依赖-->
<dependencies>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-all</artifactId>
</dependency>
</dependencies>
<!--protobuf-maven-plugin插件-->
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<!--suppress UnresolvedMavenProperty -->
<protocArtifact>com.google.protobuf:protoc:${protoc.version}:exe:${os.detected.classifier}
</protocArtifact>
<pluginId>grpc-java</pluginId>
<!--suppress UnresolvedMavenProperty -->
<pluginArtifact>io.grpc:protoc-gen-grpc-java:${grpc.version}:exe:${os.detected.classifier}
</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
proto文件定义
syntax = "proto3"; // 协议版本
// 选项配置
option java_package = "com.example";
option java_outer_classname = "RPCDateServiceApi";
option java_multiple_files = true;
// 定义包名
package com.example;
// 服务接口.定义请求参数和相应结果 会生成RPCDateServiceGrpc接口
service RPCDateService {
rpc getDate (RPCDateRequest) returns (RPCDateResponse) {
}
}
// 定义请求体
message RPCDateRequest {
string userName = 1;
}
// 定义相应内容
message RPCDateResponse {
string serverDate = 1;
}
生成Bean和接口
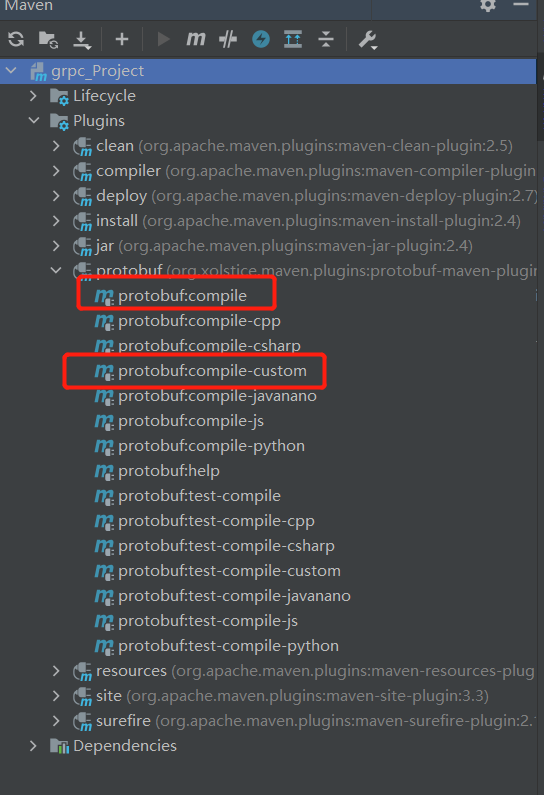
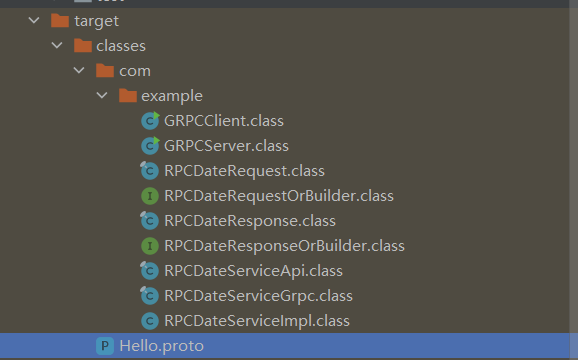
定义接口实现类 (RPCDateServiceGrpc的实现类)
public class RPCDateServiceImpl extends RPCDateServiceGrpc.RPCDateServiceImplBase {
@Override
public void getDate(RPCDateRequest request, StreamObserver<RPCDateResponse> responseObserver) {
// 请求结果,我们定义的
RPCDateResponse rpcDateResponse = null;
String userName = request.getUserName();
String response = String.format("你好: %s, 今天是%s.", userName,
new SimpleDateFormat("yyyy-MM-dd HH:mm:ss: a").format(new Date()));
try {
// 定义响应,是一个builder构造器.
rpcDateResponse = RPCDateResponse
.newBuilder()
.setServerDate(response)
.build();
} catch (Exception e) {
responseObserver.onError(e);
} finally {
// 这种写法是observer,异步写法,老外喜欢用这个框架.
responseObserver.onNext(rpcDateResponse);
}
responseObserver.onCompleted();
}
}
服务端
public class GRPCServer {
private static final int port = 9999;
public static void main(String[] args) throws Exception {
// 设置service接口.
Server server = ServerBuilder.
forPort(port)
.addService(new RPCDateServiceImpl())
.build().start();
System.out.println(String.format("GRpc服务端启动成功, 端口号: %d.", port));
server.awaitTermination();
}
}
客户端
public class GRPCClient {
private static final String host = "127.0.0.1";
private static final int serverPort = 9999;
public static void main(String[] args) throws Exception {
// 1. 拿到一个通信的channel
ManagedChannel managedChannel = ManagedChannelBuilder.forAddress(host, serverPort).usePlaintext().build();
try {
// 2.拿到道理对象
RPCDateServiceGrpc.RPCDateServiceBlockingStub rpcDateService = RPCDateServiceGrpc.newBlockingStub(managedChannel);
for (int i = 0; i < 5; i++) {
RPCDateRequest rpcDateRequest = RPCDateRequest
.newBuilder()
.setUserName("anthony"+i)
.build();
// 3. 请求
RPCDateResponse rpcDateResponse = rpcDateService.getDate(rpcDateRequest);
// 4. 输出结果
System.out.println(rpcDateResponse.getServerDate());
Thread.sleep(2000);
}
} finally {
// 5.关闭channel, 释放资源.
managedChannel.shutdown();
}
}
}
结果
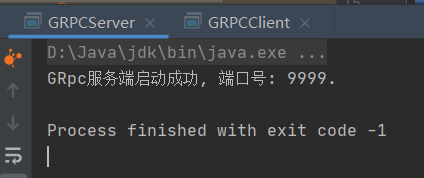
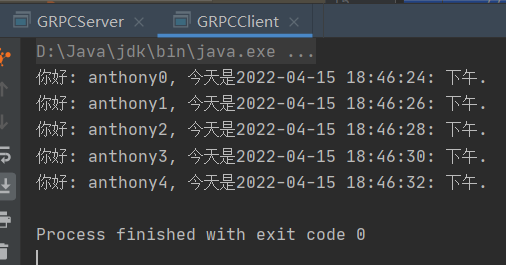