基本介绍
- 反射可以在运行时动态的获取变量的各种信息,比如变量的类型(type)、类别(kind)
- 如果是结构体变量,还可以获取到结构体本身的信息,包括结构体的字段、方法
- 通过反射,可以修改变量的值,可以调用关联的方法
- 使用反射,需要import(”reflect”)
反射
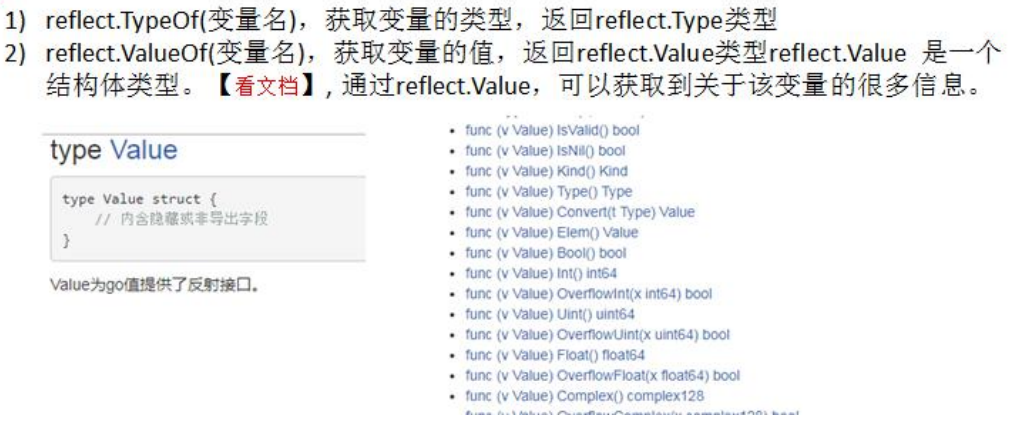
变量、interface{}、reflect.Value是可以相互转换的,这点在实际开发中,会经常使用到。
细节说明
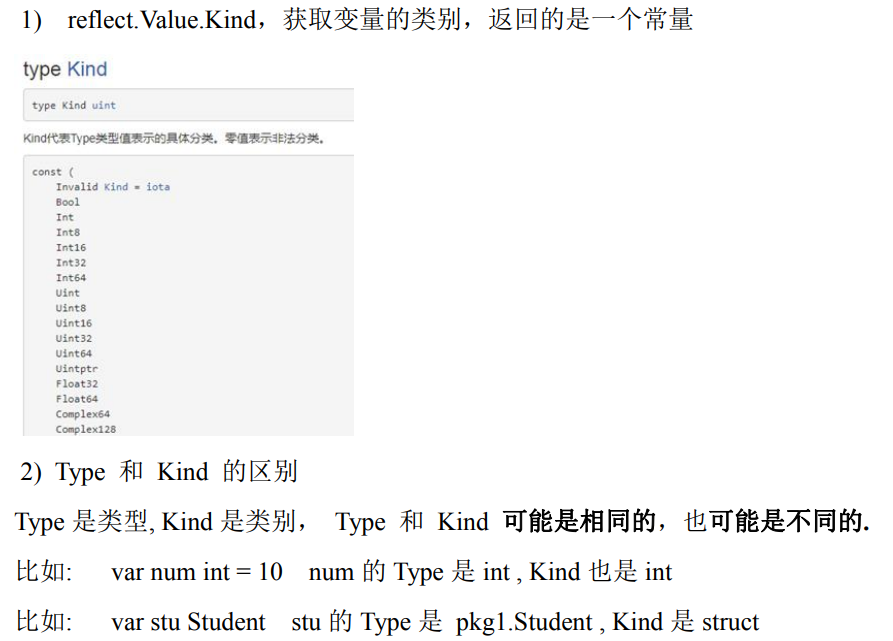
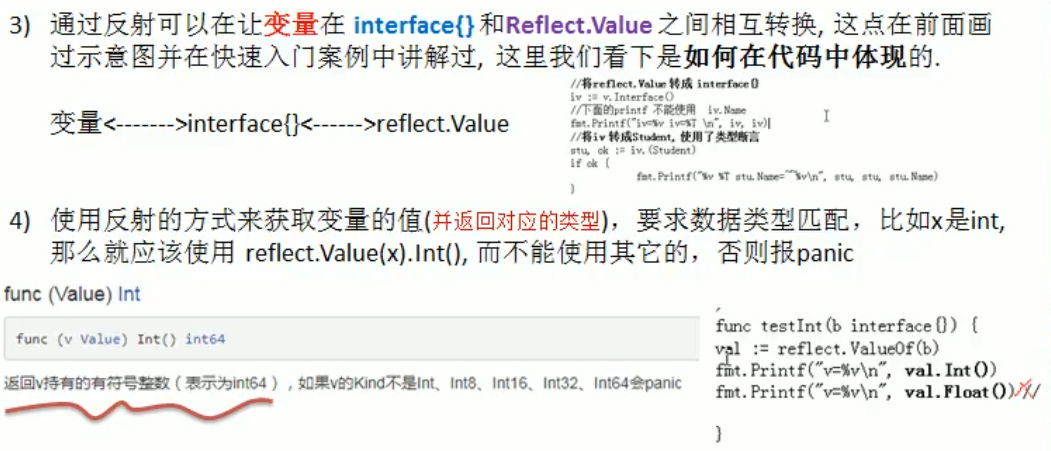
代码案例
package AdvancedLearn
import (
"fmt"
"reflect"
)
type Student struct{
Name string
Age int
}
// 专门演示反射
func reflectDemoInt(b interface{}){
// 通过反射 获取传入变量的 type , kind , value
rTyp := reflect.TypeOf(b)
fmt.Println(rTyp)
rVal := reflect.ValueOf(b) //不能直接参与计算
fmt.Println(2+rVal.Int()) //转值计算
fmt.Println(rVal)
//将rVal转成 interface{} => 再转化为对应类型
iV := rVal.Interface()
num2 := iV.(int)
fmt.Println(num2)
}
func reflectDemoStruct(b interface{}){
// 通过反射 获取传入变量的 type , kind , value
rTyp := reflect.TypeOf(b)
fmt.Println("rType =",rTyp) // rType = AdvancedLearn.Student
rVal := reflect.ValueOf(b) //不能直接参与计算
fmt.Println("rValue =",rVal) // rValue = {BigHead 25}
//将rVal转成 interface{} => 再转化为对应类型
iV := rVal.Interface()
fmt.Println(iV)
// iV.Name 此时不可行
stu2 , ok := iV.(Student)
if ok{
fmt.Println(stu2.Name,stu2.Age)
}
}
func TestReflect() {
// 基本数据类型 num的反射操作
//var num int = 100
//reflectDemoInt(num)
// type = int(打印值,实际为reflect.type)
// value = 100(打印值,实际为reflect.value)
stu := Student{"BigHead",25}
reflectDemoStruct(stu)
}