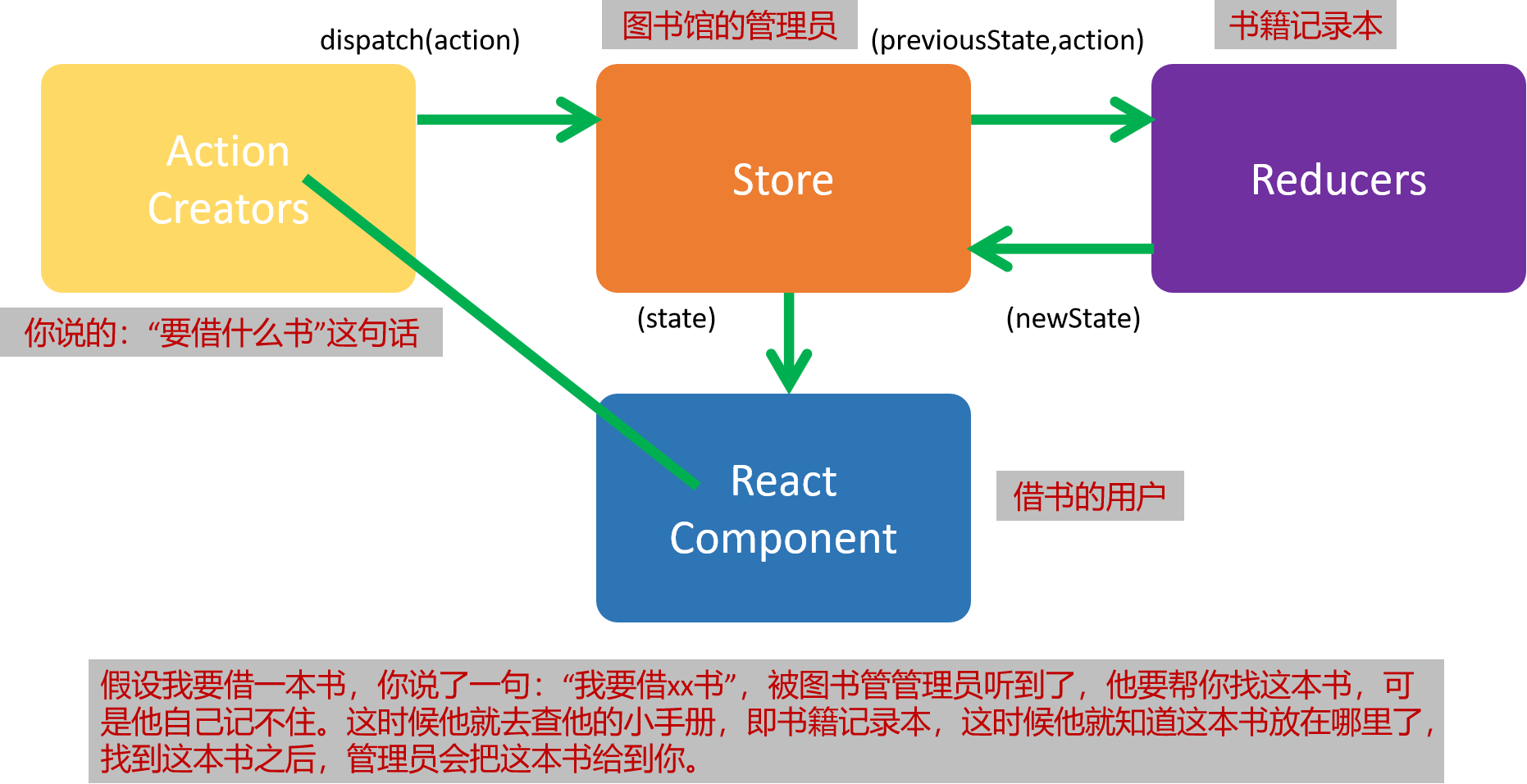
0、基本概念
store 就是保存数据的地方,将store看成一个容器,一个应用只能有一个store
//如何生成一个store createStore
//store--index.js
import { createStore } from 'redux'
const defaultState = {
msg:"react很不好用"
}
const reducer = (state=defaultState,action)=>{
return state;
}
// createStore只能接收函数
let store = createStore(reducer);
export default store;
reducer 就是对state重新计算的过程就是reducer,reducer必须是函数
1、安装依赖
yarn add redux
2、项目配置
store
--index.js
--reducer.js
2-1、创建store容器,管理数据
//index.js
import {createStore} from 'redux';
import reducer from './reducer';
const store = createStore(reducer);
export default store;
2-2、创建reducer,接收action,重新计算state
//reducer.js
let defaultState = {
msg:"react"
}
export default (state=defaultState,action)=>{
return state
}
3-1、在Home.js中导入store(需要在哪里使用就在哪里导入)
import store from '../store'js
class Home extends Component {
constructor(props) {
super(props);
this.state = getState();
}
....
}
3-2、action(View发出的一个通知,要改变state)
//通过一个事件派发action
1.创建一个ation,同时派发
class Home extends Component {
...
render() {
return (
<div>
<button onClick={this.handleClick}>改变store</button>
</div>
);
}
handleClick=()=>{
const action = {
type:"btn_change",
value:"复习redux"
}
store.dispatch(action)
}
}
export default Home;
//2.store自动接收action,之后将action传递给reducer
//3.在reducer改变state
let defaultState = {
msg:"react"
}
//reducer可以接收state,但是不能直接修改state,所以这里做一个深拷贝
export default (state=defaultState,action)=>{
//看数据派发过来没有
console.log(action)
switch(action.type){
case "btn_change":
var newState = {...state};
newState.msg = action.value;
return newState;
default:
return state;
}
}
//4.订阅store的状态的改变,只要store改变,store.subscribe(fn),fn函数必然会执行
class Home extends Component {
constructor(props) {
...
store.subscribe(this.handleStoreChange);
}
...
handleStoreChange=()=>{
console.log(1)
this.setState(store.getState())
}
}