题目链接
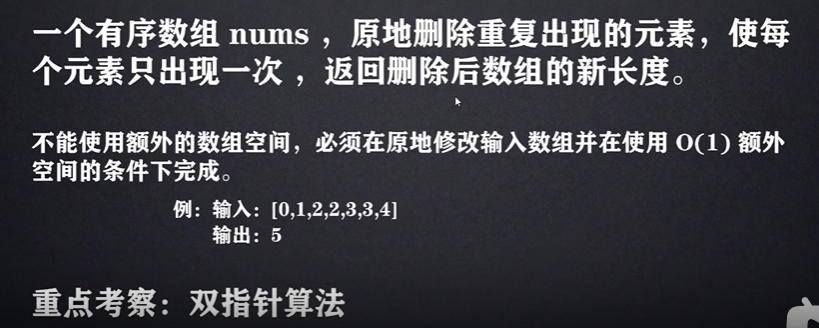
class Solution {
// 1.我的方法
public int removeDuplicates1(int[] nums) {
int left=0,right=0;
int len = nums.length;
while(left < len) {
if(left > 0 && nums[left] == nums[left-1]) {
left++;
} else {
if(left != right) {
nums[right] = nums[left];
right++;
left++;
} else {
left++;
right++;
}
}
}
return right;
}
// 2.老师的
public int removeDuplicates2(int[] nums) {
if(nums.length == 0) {
return 0;
}
int i = 0;
for(int j = 1; j < nums.length; j++) {
if(nums[j]!= nums[i]) {
i++; // 快指针往后移动
nums[i] = nums[j];
}
}
return i+1;
}
// 3.我的方法,调整
public int removeDuplicates(int[] nums) {
int right=1,left=0;
int len = nums.length;
while(right < len) {
if(nums[left] == nums[right]) { // 相等的时候右边的指针进行移动
right++;
} else { // 不相等的时候,将左指针向右移动一位,再进行赋值。如果是相同位置的话,等于重复赋值,不用关心。
left++;
nums[left] = nums[right];
right++;
}
}
return left+1;
}
}