1.PyTest 测试用例默认执行顺序
pytest默认执行用例是根据项目下的文件夹名称按Ascii码去收集的, module里面的用例是从上<br /> 往下执行。
** 例子 9-1 **<br />** **<br />** 目录:**<br />** **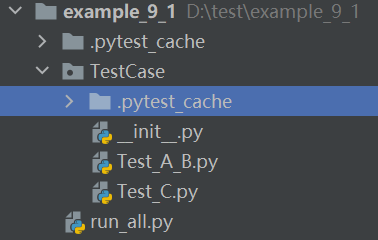<br />** 代码: **<br />** Test_A_B.py**
# -*- coding:utf-8 -*-
# Author: tang_ren_li
# 2021-1-26 22:45
class Test_B():
def test_4(self):
print("Test_B test4 run ")
assert 1
def test_3(self):
print("Test_B test3 run ")
assert 1
def test_b(self):
print("Test_B test3 run ")
assert 1
def test_a(self):
print("Test_B test3 run ")
assert 1
class Test_A():
def test_4(self):
print("Test_C test4 run ")
assert 1
def test_3(self):
print("Test_C test3 run ")
assert 1
def test_b(self):
print("Test_C test3 run ")
assert 1
def test_a(self):
print("Test_C test3 run ")
assert 1
Test_C.py
# -*- coding:utf-8 -*-
# Author: tang_ren_li
# 2021-1-26 22:45
class Test_C():
def test_4(self):
print("Test_C test4 run ")
assert 1
def test_3(self):
print("Test_C test3 run ")
assert 1
def test_b(self):
print("Test_C test3 run ")
assert 1
def test_a(self):
print("Test_C test3 run ")
assert 1
模块里测试用例的顺序从上到下 按ASC码是打乱的
run_all.py
# -*- coding:utf-8 -*-
# Author: tang_ren_li
# 2021-1-26 22:45
import pytest
if __name__ == '__main__':
pytest.main(["-s"])
执行结果:
TestCase\Test_A_B.py Test_B test4 run
.Test_B test3 run
.Test_B test_b run
.Test_B test_a run
.Test_A test4 run
.Test_A test3 run
.Test_A test_b run
.Test_A test_a run
.
TestCase\Test_C.py Test_C test4 run
.Test_C test3 run
.Test_C test_b run
.Test_C test_a run
.
说明:
1.模块里的测试用例并不会像unittest测试用例一样 会按照ASC码进行排序,是按照从上往下,即代码编写的顺序进行执行了, 这样当然更方便,在编写代码时就可以排序。 改变顺序只需要改变测试用例在模块中的先后顺序就可以了。
2.对于测试模块的执行顺序还是按照ASC码命名进行排序, 由于测试模块数量一般是可控的,所以在命名上进行排序也是很方便的
2.PyTest 控制测试用例执行顺序
2.1 前言
在软件测试测试用例设计的理论中, 一般认为测试用例的独立性越强越好, 即测试颗粒度越高越好。 所以不应该因为测试用例的互相依赖执行,来对测试用例进行排序, 应该尽量解耦。
对于模块文件执行的顺序, 通过文件按照ASC码命名对测试执行的顺序进行控制是最方便的。<br /> <br /> 对于模块里方法执行顺序控制则通过插件 pytest-ordering 来控制
2.2 pytest-ordering 插件安装
** 安装命令:**pip install pytest-ordering
**查看安装结果:**pip show pytest-ordering<br /> <br /> 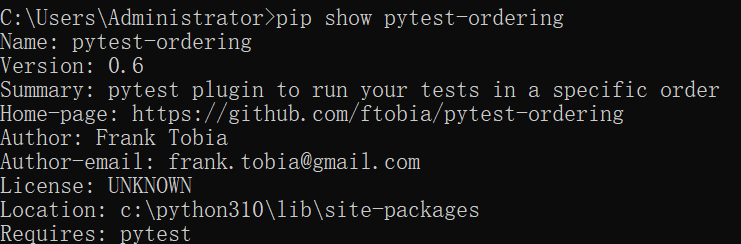
2.3 pytest-ordering 插件使用
通过装饰器@pytest.mark.run来控制case的执行顺序
方式一:
第一个执行: @ pytest.mark.run(‘first’)
第二个执行: @ pytest.mark.run(‘second’)
倒数第二个执行: @ pytest.mark.run(‘second_to_last’)
最后一个执行: @ pytest.mark.run(‘last’)
方式二:
第一个执行: @ pytest.mark.first
第二个执行: @ pytest.mark.second
倒数第二个执行: @ pytest.mark.second_to_last
第四个执行: @pytest.mark.last
方式三:
第一个执行: @ pytest.mark.run(order=1)
第二个执行: @ pytest.mark.run(order=2)
倒数第二个执行: @ pytest.mark.run(order=-2)
最后一个执行: @ pytest.mark.run(order=-1)
执行优先级:
0>较小的正数>较大的正数>无标记>较小的负数>较大的负数
通过order 插件则不需要更改测试用例在模块中的上下顺序,只需要对测试用例加上<br /> 装饰器就可以控制测试用例的顺序
例子 9-2
**基于9-1 Test_A_B 代码进行修改 **
# -*- coding:utf-8 -*-
# Author: tang_ren_li
# 2021-1-26 22:45
import pytest
class Test_B():
@pytest.mark.last #最后一个运行
def test_4(self):
print("Test_B test4 run ")
assert 1
def test_3(self):
print("Test_B test3 run ")
assert 1
@pytest.mark.run(order=-2)#到数第二个运行
def test_b(self):
print("Test_B test_b run ")
assert 1
@pytest.mark.run(order=2)#第三个运行
def test_a(self):
print("Test_B test_a run ")
assert 1
class Test_A():
def test_4(self):
print("Test_A test4 run ")
assert 1
def test_3(self):
print("Test_A test3 run ")
assert 1
@pytest.mark.run(order=1)#第二个运行
def test_b(self):
print("Test_A test_b run ")
assert 1
@pytest.mark.first#第一个运行
def test_a(self):
print("Test_A test_a run ")
assert 1
运行结果
TestCase\Test_A_B.py
Test_A test_a run
.Test_A test_b run
.Test_B test_a run
.Test_B test3 run
.Test_A test4 run
.Test_A test3 run
.
TestCase\Test_C.py Test_C test4 run
.Test_C test3 run
.Test_C test_b run
.Test_C test_a run
.
TestCase\Test_A_B.py Test_B test_b run
.Test_B test4 run
说明:
可以发现,是按照插件pytest-ordering 装饰规则的顺序进行运行的