声明新类型
- 关键字 type 可以用来声明新类型:
- type celsius float64
- var temperature celsius = 20
- 虽然 Celsius 是一种全新的类型,但是由于它和 float64 具有相同的行为和表示,所以赋值操作能顺利执行。
- 例如加法等运算,也可以像 float64 那样使用。
package main
func main() {
type celsius float64
const degrees = 20
var temperature celsius = degrees
temperature += 10
}
- 为什么要声明新类型:极大的提高代码可读性和可靠性
- 不同的类型是无法混用的
通过方法添加行为
- 在 C#、Java 里,方法属于类
- 在 Go 里,它提供了方法,但是没提供类和对象
- Go 比其他语言的方法要灵活
- 可以将方法与同包中声明的任何类型
相关联,但不可以是 int、float64 等预
声明的类型进行关联。
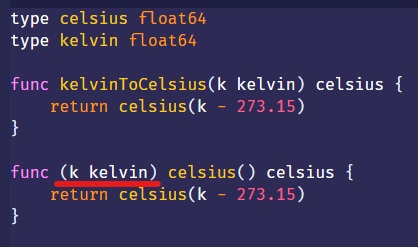
- 上例中,celsius 方法虽然没有参数。但它前面却有一个类型参数的接收者。
- 每个方法可以有多个参数,但只能有一个接收者。
- 在方法体中,接收者的行为和其它参数一样。
- 方法声明图解:
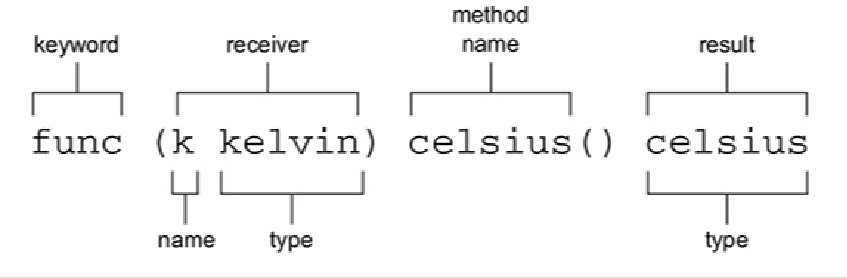
方法调用
作业题
- 编写一个程序:
- 它包含三种类型:celsius、fahrenheit、kelvin
- 3 种温度类型之间转换的方法
package main
import "fmt"
type celsius float64
func (c celsius) fahrenheit() fahrenheit {
return fahrenheit((c * 9.0 / 5.0) + 32.0)
}
func (c celsius) kelvin() kelvin {
return kelvin(c + 273.15)
}
type fahrenheit float64
func (f fahrenheit) celsius() celsius {
return celsius((f - 32.0) * 5.0 / 9.0)
}
func (f fahrenheit) kelvin() kelvin {
return f.celsius().kelvin()
}
type kelvin float64
func (k kelvin) celsius() celsius {
return celsius(k - 273.15)
}
func (k kelvin) fahrenheit() fahrenheit {
return k.celsius().fahrenheit()
}
func main() {
var k kelvin = 200.0
c := k.celsius()
fmt.Print(k, "º K is ", c, "º C")
}