温度表
- 编写一个温度转换表格程序:
- 画两个表格:
- 第一个表格有两列,第一列是摄氏度,第二列是华氏度。
- 从 -40℃ 打印到 100℃,间隔为 5 ℃,并将摄氏度转化为华氏度。
- 第二个表格就是第一个表格的两列互换一下,从华氏度转化为摄氏度。
- 负责画线和填充值的代码都应该是可复用的。画表格和计算温度应该用不同的函数分别来实现。
- 实现一个 drawTable 函数,它接受一个一等函数作为参数,调用该函数就可以绘制每一行的温度。传入不同的函数就可以产生不同的输出数据。
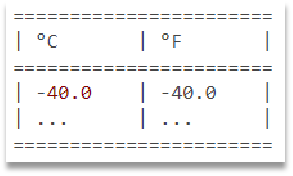
package main
import "fmt"
type celsius float64
func (c celsius) fahrenheit() fahrenheit {
return fahrenheit((c * 9.0 / 5.0) + 32.0)
}
type fahrenheit float64
func (f fahrenheit) celsius() celsius {
return celsius((f - 32.0) * 5.0 / 9.0)
}
const (
line = "======================="
rowFormat = "| %8s | %8s |\n"
numberFormat = "%.1f"
)
type getRowFn func(row int) (string, string)
func drawTable(hdr1, hdr2 string, rows int, getRow getRowFn) {
fmt.Println(line)
fmt.Printf(rowFormat, hdr1, hdr2)
fmt.Println(line)
for row := 0; row < rows; row++ {
cell1, cell2 := getRow(row)
fmt.Printf(rowFormat, cell1, cell2)
}
fmt.Println(line)
}
func ctof(row int) (string, string) {
c := celsius(row*5 - 40)
f := c.fahrenheit()
cell1 := fmt.Sprintf(numberFormat, c)
cell2 := fmt.Sprintf(numberFormat, f)
return cell1, cell2
}
func ftoc(row int) (string, string) {
f := fahrenheit(row*5 - 40)
c := f.celsius()
cell1 := fmt.Sprintf(numberFormat, f)
cell2 := fmt.Sprintf(numberFormat, c)
return cell1, cell2
}
func main() {
drawTable("ºC", "ºF", 29, ctof)
fmt.Println()
drawTable("ºF", "ºC", 29, ftoc)
}