什么是文件?
文件是保存数据的地方,比如大家经常使用的word文档,txt文件,excel文件...都是文件。
它既可以保存一张图片,也可以保存视频、声音...
文件流 ``` 文件在程序中是以流的形式来操作的
流:数据在数据源(文件)和程序(内存)之间经历的路径 输入流:数据从数据源(文件)到程序(内存)的路径 输出流:数据从程序(内存)到数据源(文件)的路径
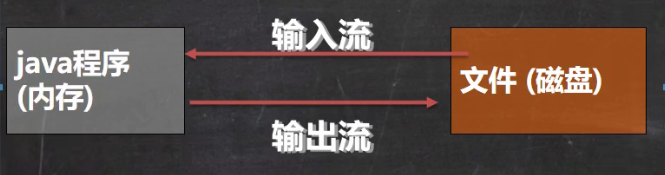
- 创建文件对象相关构造器和方法
new File(String pathname) // 根据路径构造一个File对象 new File(File parent, String child) // 根据父目录文件 + 子路径构建 new File(String parent, String child) //根据父目录 + 字路径构建
createNewFile 创建新文件
```java
// 方式一 new File(String pathname)
@Test
public void create01() {
String filePath = "e:\\news1.txt";
File file = new File(filePath);
try {
file.createNewFile();
System.out.println("文件创建成功");
} catch (IOException e) {
e.printStackTrace();
}
}
// 方式二 new File(File parent, String child) // 根据父目录文件 + 子路径构建
@Test
public void create02(){
File parentFile = new File("e:\\");
String fileName = "new2.txt";
// 这里的file对象,在java程序中,只是一个对象
File file = new File(parentFile, fileName);
try {
file.createNewFile();
System.out.println("创建成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
// 方式三 new File(String parent, String child) //根据父目录 + 子路径构建
@Test
public void create03() {
String parent = "e:\\";
String fileName = "news3.txt";
File file = new File(parent, fileName);
try {
file.createNewFile();
System.out.println("创建成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
目录的操作和文件删除
mkdir:创建一级目录
mkdirs:创建多级目录
delete:删除空目录或文件
IO流原理
1.I/O是Input/Output的缩写,I/O技术是非常实用的技术,用于处理数据的传输,如读写文件,网络通讯等
2.Java程序中,对于数据的输入.输出操作以“流(Stream)”的方式进行
3.java.io包下提供了何种“流”类和接口,用以获取不同类的数据,并通过方法输入或输出数据
4.输入input:读取外部数据(磁盘、光盘等存储设备的数据)到程序(内存)中
5.输出output:将程序(内存)数据输出到磁盘,光盘等存储设备中
流的分类
按操作数据单位不同分为:字节流(8 bit)二进制文件,字符流(按字符)文本文件
按数据流的流向不同分为:输入流、输出流
按流的角色的不同分为:字节流、处理流/包装流
| (抽象基类) | 字节流 | 字符流 | | —- | —- | —- | | 输入流 | InputStream | Reader | | 输出流 | OutputStream | Writer |
1.Java的IO流共涉及40多个类,实际上非常规则,都是从如上4个抽象基类派生的
2.由这四个类派生出来的子类名称都是以其父类名作为子类名后缀