一、单线程模式
1.1 模型图
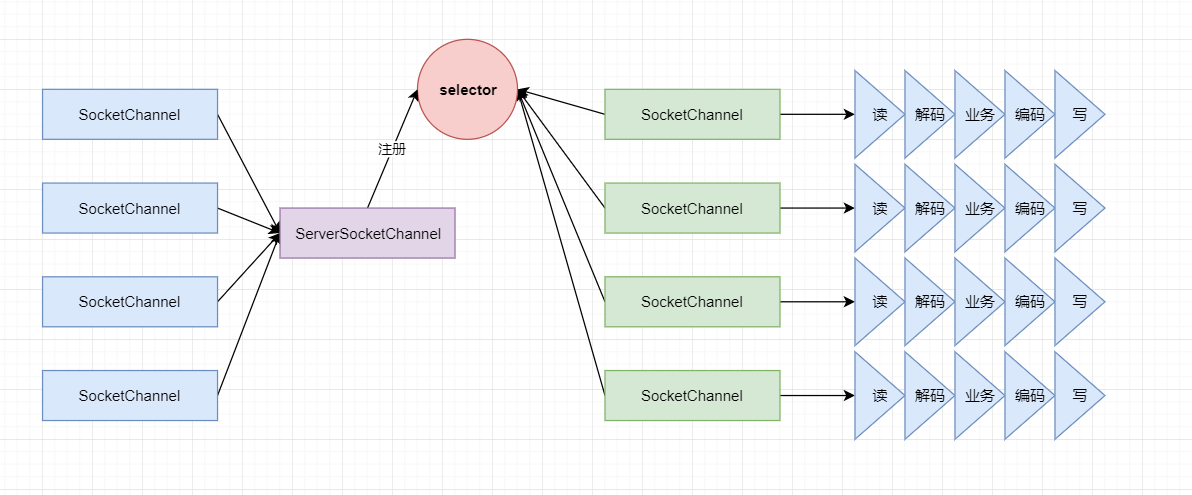
1.2 代码实现
public class Main {
public static void main(String[] args) throws IOException {
TCPReactor tcpReactor = new TCPReactor(9090);
tcpReactor.run();
}
}
public class TCPReactor implements Runnable {
private ServerSocketChannel serverSocketChannel;
private Selector selector;
public TCPReactor(int port) throws IOException {
selector = Selector.open();
serverSocketChannel = ServerSocketChannel.open();
ServerSocket socket = serverSocketChannel.socket();
socket.bind(new InetSocketAddress(port)); // 绑定端口
serverSocketChannel.configureBlocking(false); // 设置非阻塞
SelectionKey selectionKey = serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT); // 处理 accept 事件
selectionKey.attach(new Acceptor(selector, serverSocketChannel)); // 附件一个对象
}
@Override
public void run() {
while (!Thread.interrupted()) {
System.out.println("Waiting for new event on port: " + serverSocketChannel.socket().getLocalPort());
try {
if (selector.select() == 0) {
continue;
}
} catch (IOException e) {
e.printStackTrace();
}
Set<SelectionKey> selectionKeys = selector.selectedKeys();
Iterator<SelectionKey> iterator = selectionKeys.iterator();
while (iterator.hasNext()) {
SelectionKey selectionKey = iterator.next();
dispatch(selectionKey);
iterator.remove();
}
}
}
private void dispatch(SelectionKey selectionKey) {
// 第一次是 accept 事件(Acceptor)
// 后面就有可能是 read 事件(TCPHandler),根据需要进行 dispatch
Runnable r = (Runnable) selectionKey.attachment();
if (r != null) {
r.run();
}
}
}
public class Acceptor implements Runnable {
Selector selector;
ServerSocketChannel serverSocketChannel;
public Acceptor(Selector selector, ServerSocketChannel serverSocketChannel) {
this.selector = selector;
this.serverSocketChannel = serverSocketChannel;
}
@Override
public void run() {
try {
SocketChannel socketChannel = serverSocketChannel.accept(); // 接收客户端请求
System.out.println(socketChannel.socket().getRemoteSocketAddress().toString() + " is connected");
if (socketChannel != null) {
socketChannel.configureBlocking(false); // 设置非阻塞
SelectionKey selectionKey = socketChannel.register(selector, SelectionKey.OP_READ);
selectionKey.attach(new TCPHandler(selectionKey, socketChannel));
selector.wakeup(); // 让一个阻塞的 selector 操作立即返回
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class TCPHandler implements Runnable {
SelectionKey selectionKey;
SocketChannel socketChannel;
int state = 0;
public TCPHandler(SelectionKey selectionKey, SocketChannel socketChannel) {
this.selectionKey = selectionKey;
this.socketChannel = socketChannel;
state = 0; // 初始状态设置未 READING
}
@Override
public void run() {
try {
if (state == 0) {
read(); // 读取数据
} else {
send(); // 发送数据
}
} catch (Exception ex) {
System.out.println("[warning] A client has been closed");
closeChannel();
}
}
private void closeChannel() {
try {
socketChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private synchronized void read() throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(1024);
int len = socketChannel.read(buffer);
if (len == -1) {
System.out.println("[warning] A client has been closed");
closeChannel();
return;
}
String content = new String(buffer.array());
if (!content.equals(" ")) {
System.out.println(socketChannel.socket().getRemoteSocketAddress().toString() + " > " + content);
process(content);
state = 1; // 改变状态
selectionKey.interestOps(SelectionKey.OP_WRITE); // 通过 key 改变通道注册事件
selectionKey.selector().wakeup(); // 唤醒 selector
}
}
private void send() throws IOException {
String response = "Your message has send to " + socketChannel.socket().getRemoteSocketAddress().toString() + "\r\n";
ByteBuffer buffer = ByteBuffer.wrap(response.getBytes());
while (buffer.hasRemaining()) {
socketChannel.write(buffer);
}
state = 0;
selectionKey.interestOps(SelectionKey.OP_READ); // 通过 key 改变通道注册事件
selectionKey.selector().wakeup(); // 唤醒 selector
}
private void process(String content) {
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
二、多线程模型
2.1 模型图
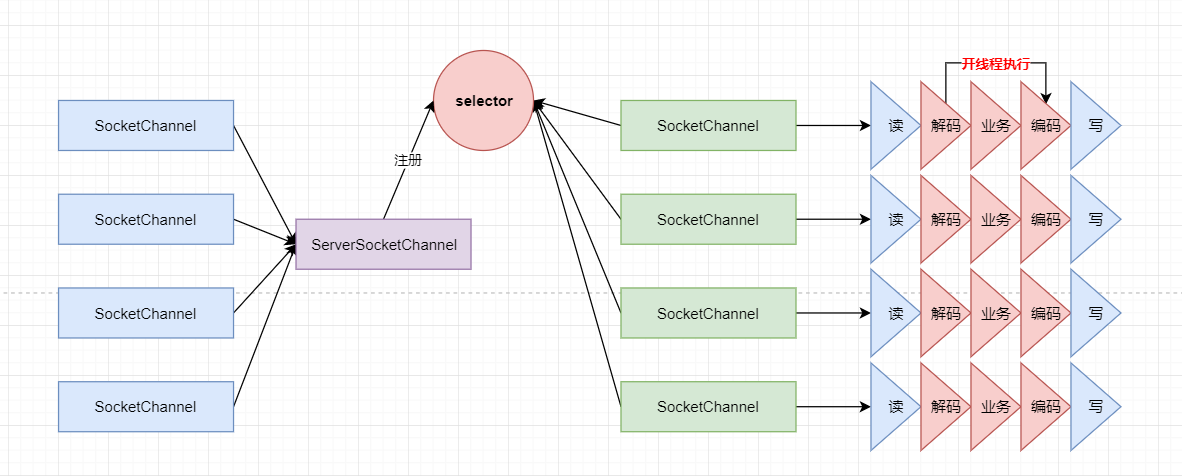
2.2 代码实现
public class Main {
public static void main(String[] args) throws IOException {
TCPReactor tcpReactor = new TCPReactor(9090);
tcpReactor.run();
}
}
public class TCPReactor implements Runnable {
private ServerSocketChannel serverSocketChannel;
private Selector selector;
public TCPReactor(int port) throws IOException {
selector = Selector.open();
serverSocketChannel = ServerSocketChannel.open();
ServerSocket socket = serverSocketChannel.socket();
socket.bind(new InetSocketAddress(port)); // 绑定端口
serverSocketChannel.configureBlocking(false); // 设置非阻塞
SelectionKey selectionKey = serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT); // 处理 accept 事件
selectionKey.attach(new Acceptor(selector, serverSocketChannel)); // 附件一个对象
}
@Override
public void run() {
while (!Thread.interrupted()) {
System.out.println("Waiting for new event on port: " + serverSocketChannel.socket().getLocalPort());
try {
if (selector.select() == 0) {
continue;
}
} catch (IOException e) {
e.printStackTrace();
}
Set<SelectionKey> selectionKeys = selector.selectedKeys();
Iterator<SelectionKey> iterator = selectionKeys.iterator();
while (iterator.hasNext()) {
SelectionKey selectionKey = iterator.next();
dispatch(selectionKey);
iterator.remove();
}
}
}
private void dispatch(SelectionKey selectionKey) {
// 第一次是 accept 事件(Acceptor)
// 后面就有可能是 read 事件(TCPHandler),根据需要进行 dispatch
Runnable r = (Runnable) selectionKey.attachment();
if (r != null) {
r.run();
}
}
}
public class TCPHandler implements Runnable {
SelectionKey selectionKey;
SocketChannel socketChannel;
HandlerState state;
private static final int THREAD_COUNTING = 10;
static ThreadPoolExecutor pool = new ThreadPoolExecutor(THREAD_COUNTING, THREAD_COUNTING,
120, TimeUnit.SECONDS, new LinkedBlockingDeque<>());
public TCPHandler(SelectionKey selectionKey, SocketChannel socketChannel) {
this.selectionKey = selectionKey;
this.socketChannel = socketChannel;
state = new ReadState(); // 初始状态设置未 READING
pool.setMaximumPoolSize(32);
}
@Override
public void run() {
try {
state.handle(this, selectionKey, socketChannel, pool);
} catch (Exception ex) {
System.out.println("[warning] A client has been closed");
closeChannel();
}
}
public void closeChannel() {
try {
socketChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void setState(HandlerState state) {
this.state = state;
}
}
public interface HandlerState {
void changeState(TCPHandler handler);
void handle(TCPHandler handler, SelectionKey key, SocketChannel sc, ThreadPoolExecutor pool) throws IOException;
}
public class ReadState implements HandlerState {
private SelectionKey sk;
@Override
public void changeState(TCPHandler handler) {
handler.setState(new WorkState());
}
@Override
public void handle(TCPHandler handler, SelectionKey sk, SocketChannel sc, ThreadPoolExecutor pool) throws IOException {
this.sk = sk;
byte[] arr = new byte[1024];
ByteBuffer buf = ByteBuffer.wrap(arr);
int numBytes = sc.read(buf); // 讀取字符串
if (numBytes == -1) {
System.out.println("[Warning!] A client has been closed.");
handler.closeChannel();
return;
}
String str = new String(arr);
if (!str.equals(" ")) {
handler.setState(new WorkState()); // 改變狀態(READING->WORKING)
pool.execute(new WorkerThread(handler, str)); // do process in worker thread
System.out.println(sc.socket().getRemoteSocketAddress().toString() + " > " + str);
}
}
/*
* 執行邏輯處理之函數
*/
synchronized void process(TCPHandler h, String str) {
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
h.setState(new WriteState()); // 改變狀態(WORKING->SENDING)
this.sk.interestOps(SelectionKey.OP_WRITE); // 通過key改變通道註冊的事件
this.sk.selector().wakeup(); // 使一個阻塞住的selector操作立即返回
}
class WorkerThread implements Runnable {
TCPHandler h;
String str;
public WorkerThread(TCPHandler h, String str) {
this.h = h;
this.str = str;
}
@Override
public void run() {
process(h, str);
}
}
}
public class WorkState implements HandlerState {
@Override
public void changeState(TCPHandler h) {
h.setState(new WriteState());
}
@Override
public void handle(TCPHandler h, SelectionKey sk, SocketChannel sc,
ThreadPoolExecutor pool) throws IOException {
}
}
public class WriteState implements HandlerState {
@Override
public void changeState(TCPHandler h) {
h.setState(new ReadState());
}
@Override
public void handle(TCPHandler h, SelectionKey sk, SocketChannel sc,
ThreadPoolExecutor pool) throws IOException {
// get message from message queue
String str = "Your message has sent to " + sc.socket().getLocalSocketAddress().toString() + "\r\n";
ByteBuffer buf = ByteBuffer.wrap(str.getBytes()); // wrap自動把buf的position設為0,所以不需要再flip()
while (buf.hasRemaining()) {
sc.write(buf); // 回傳給client回應字符串,發送buf的position位置 到limit位置為止之間的內容
}
h.setState(new ReadState()); // 改變狀態(SENDING->READING)
sk.interestOps(SelectionKey.OP_READ); // 通過key改變通道註冊的事件
sk.selector().wakeup(); // 使一個阻塞住的selector操作立即返回
}
}
三、主从模型
3.1 模型图
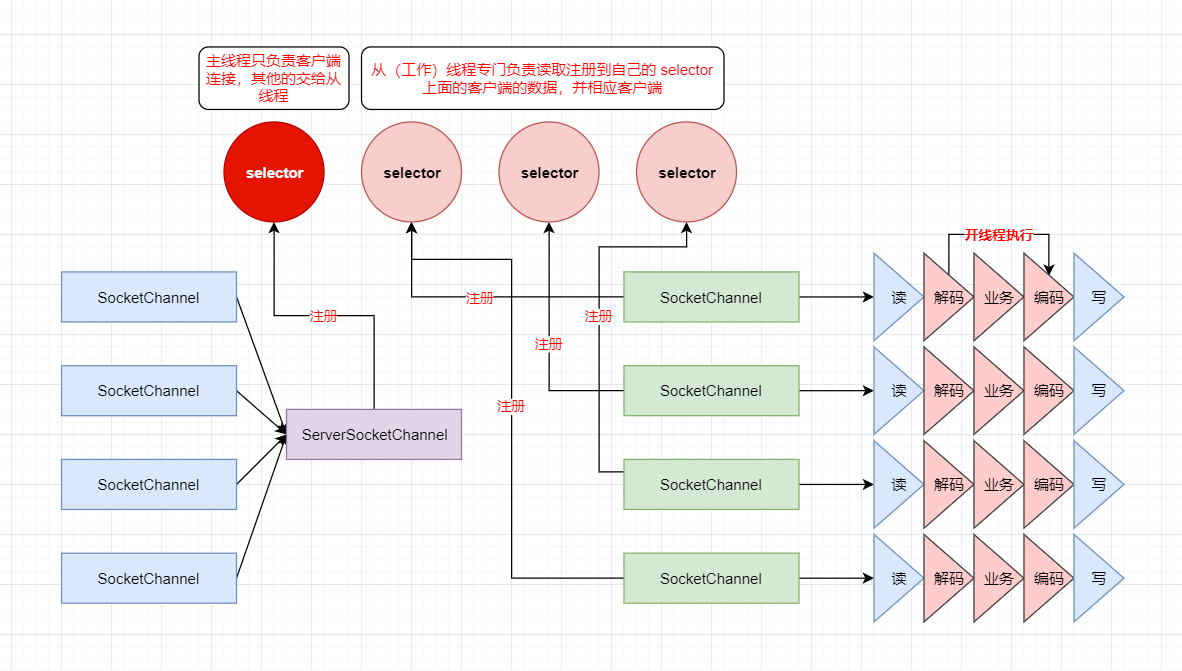
3.2 代码实现
public class Main {
public static void main(String[] args) {
try {
TCPReactor reactor = new TCPReactor(9090);
// reactor.run();
Thread thread = new Thread(reactor);
thread.start();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class TCPReactor implements Runnable {
private final ServerSocketChannel ssc;
private final Selector selector; // mainReactor用的selector
public TCPReactor(int port) throws IOException {
selector = Selector.open();
ssc = ServerSocketChannel.open();
ssc.configureBlocking(false); // 設置ServerSocketChannel為非阻塞
Acceptor acceptor = new Acceptor(ssc);
SelectionKey sk = ssc.register(selector, SelectionKey.OP_ACCEPT); // ServerSocketChannel向selector註冊一個OP_ACCEPT事件,然後返回該通道的key
sk.attach(acceptor); // 給定key一個附加的Acceptor對象
InetSocketAddress addr = new InetSocketAddress(port);
ssc.socket().bind(addr); // 在ServerSocketChannel綁定監聽端口
}
@Override
public void run() {
while (!Thread.interrupted()) { // 在線程被中斷前持續運行
System.out.println("mainReactor waiting for new event on port: " + ssc.socket().getLocalPort() + "...");
try {
if (selector.select() == 0) // 若沒有事件就緒則不往下執行
continue;
} catch (IOException e) {
e.printStackTrace();
}
Set<SelectionKey> selectedKeys = selector.selectedKeys(); // 取得所有已就緒事件的key集合
Iterator<SelectionKey> it = selectedKeys.iterator();
while (it.hasNext()) {
dispatch(it.next()); // 根據事件的key進行調度
it.remove();
}
}
}
/*
* name: dispatch(SelectionKey key)
* description: 調度方法,根據事件綁定的對象開新線程
*/
private void dispatch(SelectionKey key) {
Runnable r = (Runnable) (key.attachment()); // 根據事件之key綁定的對象開新線程
if (r != null)
r.run();
}
}
// 接受連線請求線程
package org.wesoft.study.reactor.masterandslave;
import java.io.IOException;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class Acceptor implements Runnable {
private final ServerSocketChannel ssc; // mainReactor監聽的socket通道
private final int cores = Runtime.getRuntime().availableProcessors(); // 取得CPU核心數
private final Selector[] selectors = new Selector[cores]; // 創建核心數個selector給subReactor用
private int selIdx = 0; // 當前可使用的subReactor索引
private TCPSubReactor[] r = new TCPSubReactor[cores]; // subReactor線程
private Thread[] t = new Thread[cores]; // subReactor線程
public Acceptor(ServerSocketChannel ssc) throws IOException {
this.ssc = ssc;
// 創建多個selector以及多個subReactor線程
for (int i = 0; i < cores; i++) {
selectors[i] = Selector.open();
r[i] = new TCPSubReactor(selectors[i], ssc, i);
t[i] = new Thread(r[i]);
t[i].start();
}
}
@Override
public synchronized void run() {
try {
SocketChannel sc = ssc.accept(); // 接受client連線請求
System.out.println(sc.socket().getRemoteSocketAddress().toString() + " is connected.");
if (sc != null) {
sc.configureBlocking(false); // 設置為非阻塞
r[selIdx].setRestart(true); // 暫停線程
selectors[selIdx].wakeup(); // 使一個阻塞住的selector操作立即返回
SelectionKey sk = sc.register(selectors[selIdx],
SelectionKey.OP_READ); // SocketChannel向selector[selIdx]註冊一個OP_READ事件,然後返回該通道的key
selectors[selIdx].wakeup(); // 使一個阻塞住的selector操作立即返回
r[selIdx].setRestart(false); // 重啟線程
sk.attach(new TCPHandler(sk, sc)); // 給定key一個附加的TCPHandler對象
if (++selIdx == selectors.length)
selIdx = 0;
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class TCPSubReactor implements Runnable {
private final ServerSocketChannel ssc;
private final Selector selector;
private boolean restart = false;
int num;
public TCPSubReactor(Selector selector, ServerSocketChannel ssc, int num) {
this.ssc = ssc;
this.selector = selector;
this.num = num;
}
@Override
public void run() {
while (!Thread.interrupted()) { // 在線程被中斷前持續運行
//System.out.println("ID:" + num
// + " subReactor waiting for new event on port: "
// + ssc.socket().getLocalPort() + "...");
System.out.println("waiting for restart");
while (!Thread.interrupted() && !restart) { // 在線程被中斷前以及被指定重啟前持續運行
try {
if (selector.select() == 0)
continue; // 若沒有事件就緒則不往下執行
} catch (IOException e) {
e.printStackTrace();
}
Set<SelectionKey> selectedKeys = selector.selectedKeys(); // 取得所有已就緒事件的key集合
Iterator<SelectionKey> it = selectedKeys.iterator();
while (it.hasNext()) {
dispatch((SelectionKey) (it.next())); // 根據事件的key進行調度
it.remove();
}
}
}
}
/*
* name: dispatch(SelectionKey key) description: 調度方法,根據事件綁定的對象開新線程
*/
private void dispatch(SelectionKey key) {
Runnable r = (Runnable) (key.attachment()); // 根據事件之key綁定的對象開新線程
if (r != null)
r.run();
}
public void setRestart(boolean restart) {
this.restart = restart;
}
}
public class TCPHandler implements Runnable {
private final SelectionKey sk;
private final SocketChannel sc;
private static final int THREAD_COUNTING = 10;
private static ThreadPoolExecutor pool = new ThreadPoolExecutor(THREAD_COUNTING, THREAD_COUNTING,
120, TimeUnit.SECONDS, new LinkedBlockingQueue<>()); // 線程池
HandlerState state; // 以狀態模式實現Handler
public TCPHandler(SelectionKey sk, SocketChannel sc) {
this.sk = sk;
this.sc = sc;
state = new ReadState(); // 初始狀態設定為READING
pool.setMaximumPoolSize(32); // 設置線程池最大線程數
}
@Override
public void run() {
try {
state.handle(this, sk, sc, pool);
} catch (IOException e) {
System.out.println("[Warning!] A client has been closed.");
closeChannel();
}
}
public void closeChannel() {
try {
sk.cancel();
sc.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
public void setState(HandlerState state) {
this.state = state;
}
}
public interface HandlerState {
void changeState(TCPHandler handler);
void handle(TCPHandler handler, SelectionKey key, SocketChannel sc, ThreadPoolExecutor pool) throws IOException;
}
public class ReadState implements HandlerState {
private SelectionKey sk;
@Override
public void changeState(TCPHandler handler) {
handler.setState(new WorkState());
}
@Override
public void handle(TCPHandler handler, SelectionKey sk, SocketChannel sc, ThreadPoolExecutor pool) throws IOException {
this.sk = sk;
byte[] arr = new byte[1024];
ByteBuffer buf = ByteBuffer.wrap(arr);
int numBytes = sc.read(buf); // 讀取字符串
if (numBytes == -1) {
System.out.println("[Warning!] A client has been closed.");
handler.closeChannel();
return;
}
String str = new String(arr);
if (!str.equals(" ")) {
handler.setState(new WorkState()); // 改變狀態(READING->WORKING)
pool.execute(new WorkerThread(handler, str)); // do process in worker thread
System.out.println(sc.socket().getRemoteSocketAddress().toString() + " > " + str);
}
}
/*
* 執行邏輯處理之函數
*/
synchronized void process(TCPHandler h, String str) {
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
h.setState(new WriteState()); // 改變狀態(WORKING->SENDING)
this.sk.interestOps(SelectionKey.OP_WRITE); // 通過key改變通道註冊的事件
this.sk.selector().wakeup(); // 使一個阻塞住的selector操作立即返回
}
class WorkerThread implements Runnable {
TCPHandler h;
String str;
public WorkerThread(TCPHandler h, String str) {
this.h = h;
this.str = str;
}
@Override
public void run() {
process(h, str);
}
}
}
public class WriteState implements HandlerState {
@Override
public void changeState(TCPHandler h) {
h.setState(new ReadState());
}
@Override
public void handle(TCPHandler h, SelectionKey sk, SocketChannel sc,
ThreadPoolExecutor pool) throws IOException {
// get message from message queue
String str = "Your message has sent to " + sc.socket().getLocalSocketAddress().toString() + "\r\n";
ByteBuffer buf = ByteBuffer.wrap(str.getBytes()); // wrap自動把buf的position設為0,所以不需要再flip()
while (buf.hasRemaining()) {
sc.write(buf); // 回傳給client回應字符串,發送buf的position位置 到limit位置為止之間的內容
}
h.setState(new ReadState()); // 改變狀態(SENDING->READING)
sk.interestOps(SelectionKey.OP_READ); // 通過key改變通道註冊的事件
sk.selector().wakeup(); // 使一個阻塞住的selector操作立即返回
}
}