中文文档参考 https://blog.csdn.net/zhongzh86/article/details/84070561
基础
get
static void get(String url) throws IOException {
CloseableHttpClient aDefault = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
//HttpPost httpPost = new HttpPost();
CloseableHttpResponse execute = aDefault.execute(httpGet);
execute.getStatusLine().getStatusCode(); // 获取请求状态
HttpEntity entity = execute.getEntity(); //获取消息体
String s = EntityUtils.toString(entity); // 消息体转成json字符串
Map map = JSON.parseObject(s, Map.class);
System.out.println(map);
}
post
public static def HttpPostUtil(String url,Map<String, String> header, List<BasicNameValuePair> params =null) throws IOException {
HttpPost httpPost = new HttpPost(url);
HttpClient httpClient = HttpClients.createDefault();
// 处理header
header.each {it ->httpPost.setHeader(it.key,it.value)};
// 处理请求参数
if (params !=null){
/** {@code URLEncodedUtils}
* 此处 {@code UrlEncodedFormEntity}
* 默认 "application/x-www-form-urlencoded"编码*/
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(params);
entity.setContentEncoding("UTF-8");
httpPost.setEntity(entity);
}
//执行接口请求
HttpResponse response = httpClient.execute(httpPost);
try {
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 200) {
//获取返回结果
HttpEntity resEntity = response.getEntity();
String jsonStr = EntityUtils.toString(resEntity, "UTF-8");
// System.out.println("**" + jsonStr);
JSONObject jsonObject = JSON.parseObject(jsonStr);
Boolean success = jsonObject.getBoolean("success");
if (success) {
// 成功
System.out.println("成功了");
return jsonObject
} else {
System.out.println("失败了");
println jsonObject
String errorCode = jsonObject.getString("errorCode");
String errorMsg = jsonObject.getString("errorMsg");
}
} else {
// 失败处理
throw new Exception("请求失败 code ${statusCode}")
}
} catch (Exception e) {
e.printStackTrace();
}finally {
httpClient.close()
}
}
常用方法
1:HttpClients.createDefault();
创建一个默认的HttpClient对象 ,这个对象是线程安全的,也就是说不需要每次请求都实例化一次,后面请求池会用到
这是httpclient的开始 等价于 new HttpClientBuilder().build 没有任何设置
2:HttpPost httpPost = new HttpPost(url); HttpGet httpGet = new HttpGet(url);
本次请求的方法, 常用的 就是get post,用于 HttpClient 执行请求
3:httpPost.setHeader
设置本次请求的 header 接收两个String 代表,header的键值对
4:httpPost.setEntity
本次请求的 请求体,也就是请求参数。 接收一个 Entity类型对象 常用的有<br />StringEntity 接收两个参数 <br />1:String string 代表本次请求参数<br />2: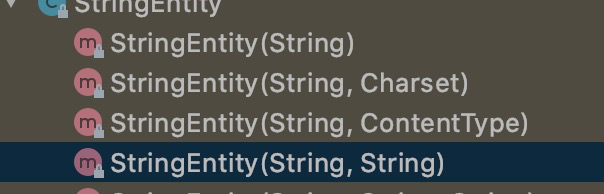第二个参数有几种选择<br />不填或 填 String 或填 charset 代表请求参数的编码,默认 的是 ISO-8859-1 这里要注意,一般我们用的都是 UTF-8 所以填字符串 UTF-8 或 new UTF-8() 效果一样<br />提交的请求头 (content-type)都是 text/plain 具体可查看源码<br />当然也可以自己指定 content-type 具体做法 可选对象查看源码
StringEntity stringEntity = new StringEntity("123", ContentType.create(ContentType.MULTIPART_FORM_DATA.getMimeType(), "UTF-8"));
HttpEntityEnclosingRequestBase httpGet = new HttpPost();
httpGet.setEntity(stringEntity);
UrlEncodedFormEntity
几个构造方法
第一个参数代表本次请求入参 常用 List <? extends NameValuePair> 形式
要求自己构建 NameValuePair ,本质上是一个键值对的列表
与 StringEntity的区别在于,默认请求头使用的是 application/x-www-form-urlencoded 默认 Charset 也是 SO-8859-1
5:HttpResponse response = httpClient.execute(httpPost)
执行http请求 返回 请求结果 常用的几个方法
execute.getStatusLine().getStatusCode(); 获取状态码
execute.getAllHeaders() 获取返回的header
execute.getEntity(); 获取返回体
此处返回的是一个 HttpEntity 对象,需要转换
提供的方法 EntityUtils.toString 可以转成字符串
_
idea自带的httpclient工具
1:路径 Tools->httpclient
2:脚本模式,任一位置 新建 XX.http 文件
3: 自带末班,新建文件右上角 有examples。各种都有
到此为止可以简单的进行使用了
后面的一般测试用不到
进阶
例子 https://www.cnblogs.com/bethunebtj/p/8493379.html
1:链接池