1,HashMap 集合的 特点:
- 元素按照键不重复,无索引,存储和取出无顺序。(与Map体系一致)
- 集合中的:键为 key;值为 value;
- 两者都是泛型;
-
2,HashMap集合 的方法:
1,创建HashMap集合:Set<集合名.Entry
> Set<Map.Entry<String, String>> entrySet = map.entrySet();
添加数据:put
- 当值不存在,则是添加;
- 当值存在时,再添加数据会修改原来的值(修改);
mapA.put("da","dd");
通过键找值:get
System.out.println(mapA.get("da"));
根据键删除对应的值:remove
mapA.remove("da");
移除所有键值对的元素:clear
mapA.clear();
判断集合是否包含指定的键:containKey
boolean da = mapA.containsKey("da");
判断集合是否包含指定的值:containValue
boolean dd = mapA.containsValue("dd");
判断是否为空:isEmpty
boolean empty = mapA.isEmpty();
集合长度:size
mapA.size();
3,HashMap的遍历方式一:键找值:keySet()
仅通过键来遍历; ```java public class M { public static void main(String[] args) { HashMap
mapA = new HashMap<>(); mapA.put(“da”,”dd”); mapA.put(“dda”,”Gd”);
//利用set集合的特性将值存在set集合达到不重复的目的,再通过遍历set集合到达键找值; Set
setKeys = mapA.keySet(); //遍历set的集合 for (String key : setKeys) { String keyA=mapA.get(key);
System.out.println(keyA);
} }
//重写toSring的输出方法: public String toString() { return “M{ }”; }
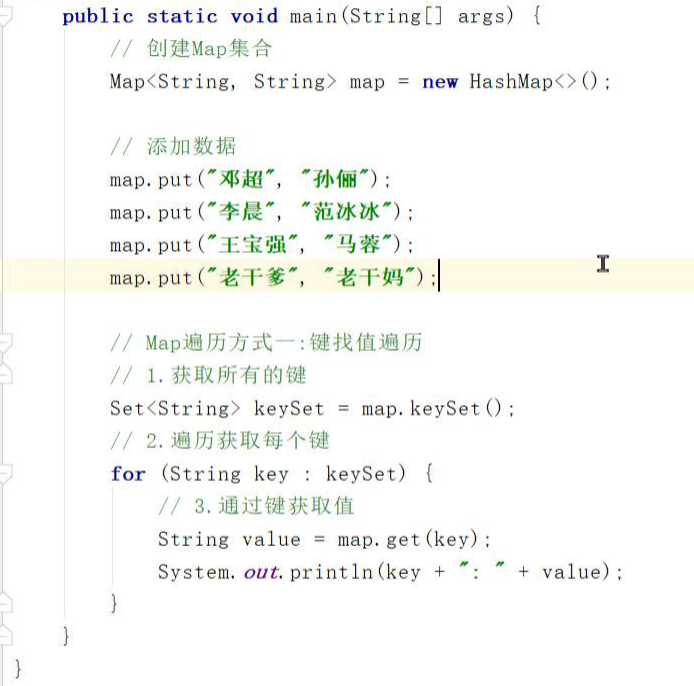
<a name="waWBr"></a>
## 4,HashMap的遍历方式二:键值对:entrySet()
1. 通过键和值同时遍历;键和值就存在一个Entry里面;而Entry存放在set集合中;**(一个键对应的一个值就是一个entry对象)**
1. 键值对的遍历流程:
1. 获取所有Entry对象;
1. 遍历每个取出的Entry;
1. 取出Entry的键和值;
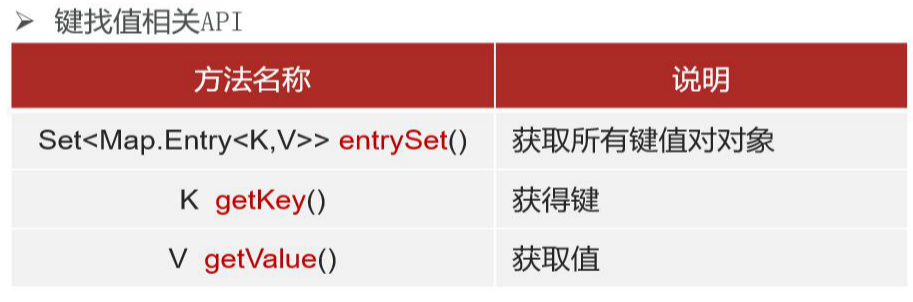
```java
//获取entry
Set<Map.Entry<String, String>> setKeys = mapA.entrySet();
//遍历取出的entry
for (Map.Entry<String, String> entry : setKeys) {
//取出entry里面的键和值:
String key = entry.getKey();
String value = entry.getValue();
System.out.println(key + " + " + value);
}
5,HashMap集合储存自定义对象并遍历:
- 去重就要去重写equals和hashcode方法,保证键的唯一性; ```java // //Student类: package day07_KeTang.Maps.Demo03;
/**
- @author Jztice5
- @date 2022年02月14日 16:43 */
public class Student { private String name; private int age; private String address;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public Student(String name, int age, String address) {
this.name = name;
this.age = age;
this.address = address;
}
/**
* 获取
* @return name
*/
public String getName() {
return name;
}
/**
* 设置
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* 获取
* @return age
*/
public int getAge() {
return age;
}
/**
* 设置
* @param age
*/
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "Student{name = " + name + ", age = " + age + "}";
}
/**
* 获取
* @return address
*/
public String getAddress() {
return address;
}
/**
* 设置
* @param address
*/
public void setAddress(String address) {
this.address = address;
}
//重写达到去重的目的:
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
if (age != student.age) return false;
if (name != null ? !name.equals(student.name) : student.name != null) return false;
return address != null ? address.equals(student.address) : student.address == null;
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age;
result = 31 * result + (address != null ? address.hashCode() : 0);
return result;
}
}
// //Text类: package day07_KeTang.Maps.Demo04;
import java.util.HashMap; import java.util.Map; import java.util.Set;
/**
- @author Jztice5
- @date 2022年02月14日 16:57 */
public class Text {
public static void main(String[] args) {
//创建Hashmap集合 mapA
HashMap
//创建学生对象并赋值;
Student s1 = new Student("da", 19);
Student s2 = new Student("da", 19);
Student s3 = new Student("da", 19);
//添加对象到集合
mapA.put(s1, "gz");
mapA.put(s2, "cq");
mapA.put(s3, "sc");
//获取mapA集合的entry对象 ;
Set<Map.Entry<Student, String>> entries = mapA.entrySet();
//遍历所有取出的entru对象
for (Map.Entry<Student, String> entry : entries) {
//将entry对象中的键和值遍历取出
Student key = entry.getKey();
String value = entry.getValue();
//out
System.out.println(key + ":" + value);
}
}
}
```