1,HashSet集合的 特点:
- 无索引
- 不重复 ,自定义类型的去重要去重写 equals和 hashcode !!!
- 存储和取出没有顺序
- 底层数据结构是哈希表(后面再深入了解)
import java.util.HashSet;
/**
- @author Jztice5
- @date 2022年02月12日 14:56 */
public class S2 { public static void main(String[] args) {
//新建HashSet集合:
HashSet<Student> set = new HashSet<>();
//创建stuednt对象
Student s1 = new Student("L", 18, 100);
Student s2 = new Student("M", 21, 100);
Student s3= new Student("H", 18, 100);
//往set集合中添加对象属性;
set.add(s1);
set.add(s2);
set.add(s3);
//增强for循环遍历set集合(这里的底层是迭代器的循环遍历)
for (Student student : set) {
// System.out.println(student.getName()+”\t”+student.getAge()+”\t”+student.getFen());
//如果要这样写的话,记得在对象类中重写toString方法输出集合数据;
System.out.println(student);
}
}
}
//Student类; package Set;
import com.sun.xml.internal.ws.api.ha.StickyFeature;
/**
- @author Jztice5
- @date 2022年02月12日 14:56 */
public class Student { private String name; private int age; private int fen;
public Student() {
}
public Student(String name, int age, int fen) {
this.name = name;
this.age = age;
this.fen = fen;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getFen() {
return fen;
}
public void setFen(int fen) {
this.fen = fen;
}
//*************************************
//重写toString,以便能够直接用对象变量直接输出集合;
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", fen=" + fen +
'}';
}
}
<a name="f071i"></a>
## 3,(重点*)HashSet集合常用的 方法(存放元素的过程):
<a name="h5WsV"></a>
### 1,hashCode():哈希算法
1. 在此方法中返回的值称为:**哈希码值**;
1. 其中,在此方法内,计算元素存放位置的算法被称为**哈希算法**
1. **计算公式为: 存入位置=哈希值%数组长度;**
4. **其中存放元素的过程为:(链表法)**
1. **根据hashcode返回的值计算存放的位置;**
1. **如果这个位置没有元素,直接存储;**
1. **如果有元素,跳转到equals方法中进行判断;**
1. **如果元素内容不同,则存进当前位置,以挂靠的方式进行存储;**
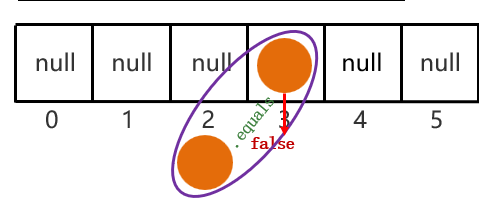 <br />横向的是数组,挂靠的是链表;数组内存的是链表的头节点key;
5. **如果元素内容相同,则不会存进去;**
5. **总结:因此,哪怕是哈希值为1 时,只要内容不同,就能一直存放下去;(但是这种方法不推荐,因为效率低下)**
<a name="AUbbC"></a>
### 2,equals():判断内容是否相同
<a name="aqGMx"></a>
### 3,代码示例:
```java
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
if (age != student.age) return false;
if (fen != student.fen) return false;
return name != null ? name.equals(student.name) : student.name == null;
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age;
result = 31 * result + fen;
return result;
}