官方文档:https://ding-doc.dingtalk.com/doc#/serverapi2/qf2nxq
本文参考来源:https://www.jianshu.com/p/cc42a7915acc
<?php
/**
* php 使用钉钉机器人发送消息.
* User: Administrator
* Date: 2019/8/29
* Time: 23:40
*/
function request_by_curl($remote_server, $post_string)
{
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $remote_server);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 5);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json;charset=utf-8'));
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_string);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// 不用开启curl证书验证
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$data = curl_exec($ch);
//$info = curl_getinfo($ch);
//var_dump($info);
curl_close($ch);
return $data;
}
$webhook = "https://oapi.dingtalk.com/robot/send?access_token=xxxxxx";
// text类型
$textString = json_encode([
'msgtype' => 'text',
'text' => [
"content" => "我就是我, 是不一样的烟火@156xxxx8827"
],
'at' => [
'atMobiles' => [
"156xxxx8827",
"189xxxx8325"
],
'isAtAll' => false
]
]);
// link类型
$textString = json_encode([
"msgtype" => "link",
"link" => [
"text" => "这个即将发布的新版本,创始人陈航(花名“无招”)称它为“红树林”。
而在此之前,每当面临重大升级,产品经理们都会取一个应景的代号,这一次,为什么是“红树林”?",
"title" => "时代的火车向前开",
"picUrl" => "",
"messageUrl" => "https://www.dingtalk.com/",
]
]);
// markdown类型
$textString = json_encode([
"msgtype" => "markdown",
"markdown" => [
"title" => "杭州天气",
"text" => "#### 杭州天气 @156xxxx8827\n" .
"> 9度,西北风1级,空气良89,相对温度73%\n\n" .
"> 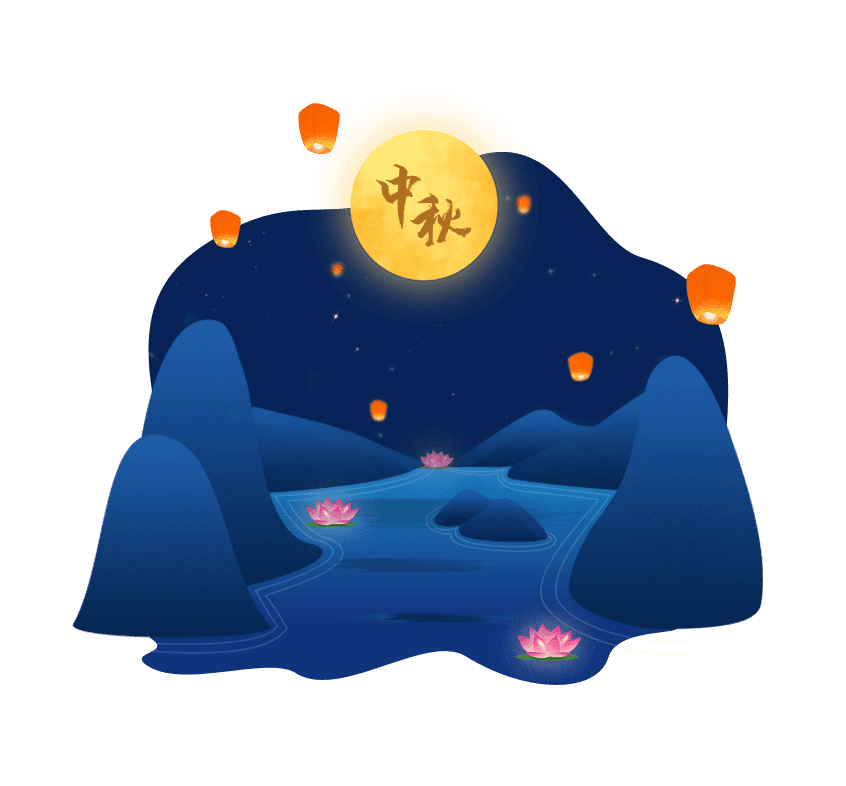\n" .
"> ###### 10点20分发布 [天气](http://www.thinkpage.cn/) \n"
],
"at" => [
"atMobiles" => [
"156xxxx8827",
"189xxxx8325"
],
"isAtAll" => false
]
]);
// 整体跳转ActionCard类型
$textString = json_encode([
"actionCard" => [
"title" => "乔布斯 20 年前想打造一间苹果咖啡厅,而它正是 Apple Store 的前身",
"text" => "
### 乔布斯 20 年前想打造的苹果咖啡厅
Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划",
"hideAvatar" => "0",
"btnOrientation" => "0",
"singleTitle" => "阅读全文",
"singleURL" => "https://www.dingtalk.com/"
],
"msgtype" => "actionCard"
]);
// 独立跳转ActionCard类型
$textString = json_encode([
"actionCard" => [
"title" => "乔布斯 20 年前想打造一间苹果咖啡厅,而它正是 Apple Store 的前身",
"text" => "
### 乔布斯 20 年前想打造的苹果咖啡厅
Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划",
"hideAvatar" => "0",
"btnOrientation" => "0",
"btns" => [
[
"title" => "内容不错",
"actionURL" => "https://www.dingtalk.com/"
],
[
"title" => "不感兴趣",
"actionURL" => "https://www.dingtalk.com/"
]
]
],
"msgtype" => "actionCard"
]);
// FeedCard类型
$textString = json_encode([
"feedCard" => [
"links" => [
[
"title" => "时代的火车向前开1",
"messageURL" => "https://www.dingtalk.com/",
"picURL" => "https://timgsa.baidu.com/timg?image&quality=80&size=b9999_10000&sec=1567105217584&di=4c91fefc045f54267edcf8c544e3bd3b&imgtype=0&src=http%3A%2F%2Fk.zol-img.com.cn%2Fdcbbs%2F16420%2Fa16419096_s.jpg"
],
[
"title" => "时代的火车向前开2",
"messageURL" => "https://www.dingtalk.com/",
"picURL" => ""
]
]
],
"msgtype" => "feedCard"
]);
$result = request_by_curl($webhook, $textString);
echo $result;
以上是使用的第一种方式,关键词,要想发送成功,文本内容必须得包含该关键词
若使用签名的方式,签名算法相关的如下所示,参考来源:https://blog.csdn.net/qq_34193883/article/details/106471299?utm_medium=distribute.pc_aggpage_search_result.none-task-blog-2~all~first_rank_v2~rank_v25-3-106471299.nonecase
<?php
$url = 'webhook地址';
// 第一步,把timestamp+"\n"+密钥当做签名字符串,使用HmacSHA256算法计算签名,然后进行Base64 encode,最后再把签名参数再进行urlEncode,得到最终的签名(需要使用UTF-8字符集)。
$time = time() *1000;//毫秒级时间戳,我这里为了方便,直接把时间*1000了
$secret = '这是密钥';
$sign = hash_hmac('sha256', $time . "\n" . $secret,$secret,true);
$sign = base64_encode($sign);
$sign = urlencode($sign);
$msg = [
'msgtype' => 'text',//这是文件发送类型,可以根据需求调整
'text' => [
'content' => '这是需要发送的内容',
],
];
$url = "{$url}×tamp={$time}&sign={$sign}";
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($msg));
curl_setopt($curl, CURLOPT_HEADER, 0);
curl_setopt($curl, CURLOPT_HTTPHEADER, ['Content-Type: application/json']);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$res = curl_exec($curl);
curl_close($curl);
var_dump($res);