package p1;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
/*
* Jframe 框架
是一个容器(也是框架),他是各个组件的载体。继承java.swing,jframe 来创建窗体
1.新建Jframe对象:
new Jframe();创建一个没有标题的窗口;
new Jframe(String str); 创建标题为str的窗口
2.常用方法
setSize(int width,int height) // 设置大小
setLocation(intx int y ) //设置窗口的位置。默认位置(0,0);
set Bounds(int a ,int b,int width,int height);//设置窗口位置和宽度
setVisible(bool b) //设置窗口是否可见 默认不可见
setDefaultCloseOperation();//设置关闭方式
DO_NOTHING_ON_CLOSE
HIDE_ON_CLOSE
DISPOSE_ON_CLOSE
EXIT_ON_CLOSE
二.JDialog
继承java.awt.Dialog类 他是从一个窗体弹出来的另一个窗口 他和JFrame 类似
JDialog 可以当作是JFrame 使用 但是必须从属于JFrame
JDialog();
JDialog(Jframe f) //指定父窗口
JDialog(Jframe f,String str)//指定父窗口 加标题
*
三.JPanel 是一种简单的面板 他继承java.awt.Container
四.JScrollPanle 带滚动条的面板
*
* */
public class Windows {
public static void main(String[] args) {
JFrame jf = new JFrame("11");
// jf.setSize(400,300);
// jf.setLocation(200,500);
jf.setBounds(800,300,1400,1400);
jf.setVisible(true);
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
// JDialog jd = new JDialog(jf,"子窗口");
// jd.setBounds(850,350,300,300);
// jd.setVisible(true);
// jf.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
//创建两个按钮
JButton bt1 = new JButton("按钮1");
JButton bt2 = new JButton("按钮2");
//创建面板
JPanel jp = new JPanel();
//将按钮添加到面板中
jp.add(bt1);
jp.add(bt2);
//将面板添加到窗体中
jf.add(jp);
}
}
/*
* JButton(String str)指定文字
* JButton(Icon icon)指定图标
* JButton(String str Icon icon)指定文字加图标
* 常用方法
* setTooltipText(String Text) 设置文字
* setBorderPainted(bool b)设置边框是否显示
* setEnable()设置是否可用
* */
class Test_JButton{
public static void main(String[] args) {
JFrame jf = new JFrame();
jf.setBounds(200,200,700,500);
//修改布局方式 默认是流式布局 改为网格布局
jf.setLayout(new FlowLayout());
JButton button = new JButton("按钮");
//设置是否可用
button.setEnabled(true);
//设置边界是否可用
button.setBorderPainted(true);
jf.add(button);
jf.setVisible(true);
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
/*
* Jlabel:标题组件
* 文字提示以及提示信息
* new JLabel()
* new JLabel(Icon icon,int aligment) 图标+水平方向
* new JLabel(String str,int aligment) 文本+水平方向
* new JLabel(Icon icon,String str,int aligment) 图标+文本+水平方向
* */
class Test_Jlabel{
public static void main(String[] args) {
JFrame jf = new JFrame();
jf.setBounds(200,200,700,500);
//修改布局方式 默认是流式布局 改为网格布局
// jf.setLayout(new FlowLayout());
//创建标题组件
JLabel jLabel = new JLabel("账号",SwingConstants.CENTER);
jf.add(jLabel);
jf.setVisible(true);
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
/*
* 事件监听
* 一个事件模型中有三个部分:事件源、事件、监听程序
* 事件源:事件发生的地址
* 事件:要发生的事情
* 事件处理:针对这个事件 做出什么处理方案
* 事件监听:将事件源和事件关联起来
*
* 分类: 事件 事件源 监听接口
* 动作事件监听器: ActionEvent JBotton Jlist JTexFiled等 ActionListener
* 焦点事件监听器: FocusEvent Component FocusListener
* 监听接口: 方法:
* ActionListener addActionListener removeActionListener
* FocusListener addActionListener removeActionListener
* */
class Test_Listener{
public static void main(String[] args) {
JFrame jf = new JFrame("play");
//设置布局
jf.setLayout(new FlowLayout(FlowLayout.LEFT));
jf.setBounds(400,400,400,400);
//创建多行文本域
JTextArea tet = new JTextArea(10,20);
//设置自动换行
tet.setLineWrap(true);
JButton bt = new JButton("拉萨");
bt.addActionListener(new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
//文本域设置文字
tet.append("回到拉萨!!!!\n");
}
});
jf.add(tet);
jf.add(bt);
jf.setVisible(true);
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
class Airplane_battle{
public static void main(String[] args) {
JFrame jFrame = new JFrame("飞机大战游戏");
jFrame.setBounds(700,200,1200,1200);
jFrame.setLayout(new FlowLayout(FlowLayout.CENTER));
JButton jButton = new JButton("点击任意键进入游戏>>>");
jButton.setBorderPainted(false);
jButton.setEnabled(true);
jButton.addActionListener(new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
JFrame jFrame = new JFrame();
jFrame.setBounds(700,200,1200,1200);
jFrame.setLayout(new FlowLayout(FlowLayout.LEFT));
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
});
jFrame.add(jButton);
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
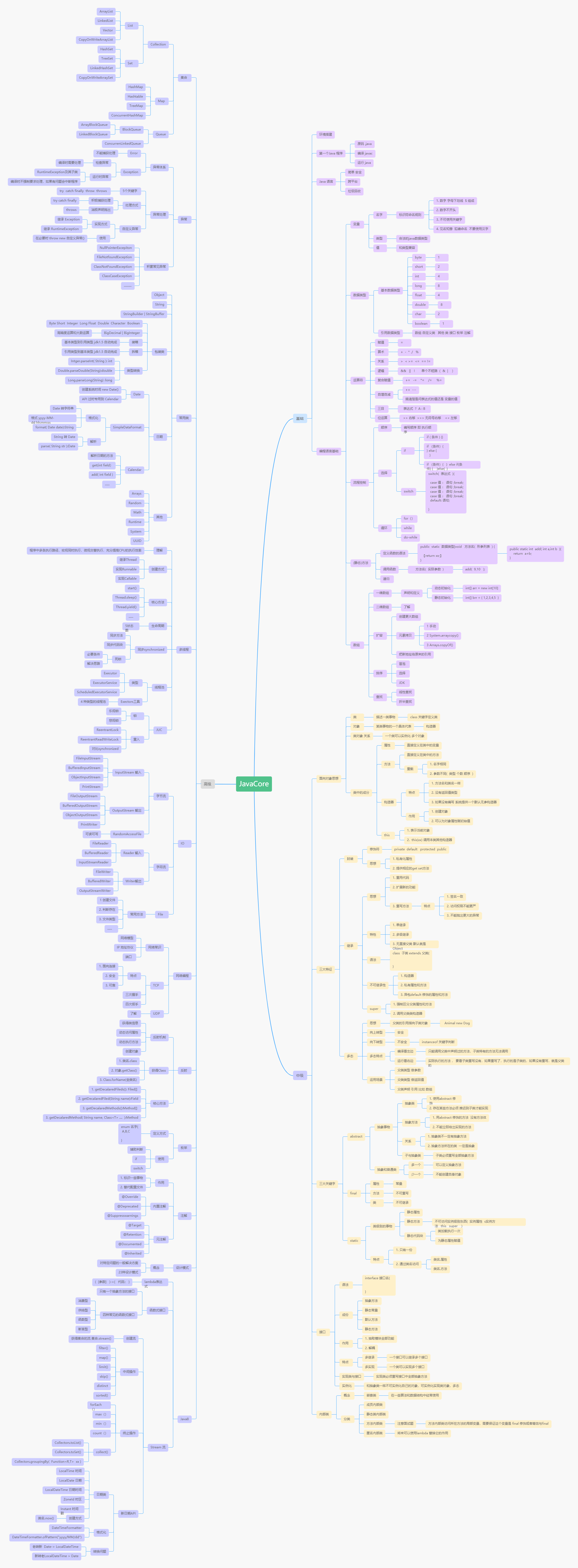