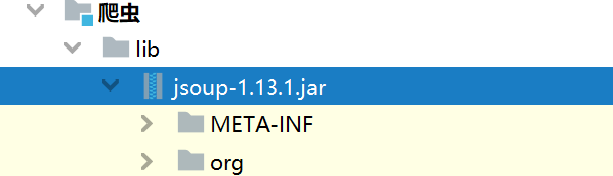
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import java.io.*;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class url {
public static void main(String[] args) throws IOException {
//存放 url地址
String url = "https://car.autohome.com.cn/pic/series/102.html#pvareaid=3454438";
URL u = new URL(url);
InputStream inputStream = u.openStream();
//一行一行读
BufferedReader bf = new BufferedReader(new InputStreamReader(inputStream,"GBK"));
//查看电脑 默认编码
System.out.println(Charset.defaultCharset());
String line;
StringBuilder sb = new StringBuilder();
while ((line=bf.readLine())!=null){
sb.append(line);
}
//用Jsoup解析html文件
Document parse = Jsoup.parse(sb.toString());
//得到所有的 img 元素集合
Elements img = parse.select("img");
ExecutorService es = Executors.newFixedThreadPool(20);
System.out.println(img.size());
for (int i = 0; i <img.size() ; i++) {
Element element = img.get(i);
String path = element.attr("src");
System.out.println(path);
//调用下载 方法
es.submit(new Runnable() {
@Override
public void run() {
try {
downloda(path);
} catch (IOException e) {
e.printStackTrace();
}
}
});
}
}
public static void downloda(String url) throws IOException {
if (url.indexOf("/")==0){
url="https:"+url;
}
URL u = new URL(url);
InputStream inputStream = u.openStream();
int begin = url.lastIndexOf("/");
//car2.autoimg.cn/cardfs/product/g20/M12/7D/FF/480x360_0_q95_c42_autohomecar__ChsElWDoSlWAESRaACQdsSr_S1I935.jpg
String filename = url.substring(begin + 1);
FileOutputStream fos = new FileOutputStream("D:/pic/"+filename);
byte[] bytes = new byte[1024];
int len ;
while ((len=inputStream.read(bytes))>0){
fos.write(bytes,0,len);
}
System.out.println("over");
}
}