1、安装react-native-vector-icons
yarn add react-native-vector-icons -D
react-native-vector-icons
内置了很多字体图标,譬如:
[Entypo](http://entypo.com/)
by Daniel Bruce (411 icons)[EvilIcons](http://evil-icons.io/)
by Alexander Madyankin & Roman Shamin (v1.8.0, 70 icons)[FontAwesome](http://fortawesome.github.io/Font-Awesome/icons/)
by Dave Gandy (v4.7.0, 675 icons)[Foundation](http://zurb.com/playground/foundation-icon-fonts-3)
by ZURB, Inc. (v3.0, 283 icons)[Ionicons](http://ionicframework.com/docs/v2/ionicons/)
by Ben Sperry (v3.0.0, 859 icons)[MaterialIcons](https://www.google.com/design/icons/)
by Google, Inc. (v3.0.1, 932 icons)[MaterialCommunityIcons](https://materialdesignicons.com/)
by MaterialDesignIcons.com (v1.9.33, 1932 icons)[Octicons](http://octicons.github.com/)
by Github, Inc. (v5.0.1, 176 icons)[Zocial](http://zocial.smcllns.com/)
by Sam Collins (v1.0, 100 icons)[SimpleLineIcons](http://simplelineicons.com/)
by Sabbir & Contributors (v2.4.1, 189 icons)2、使用react-native-vector-icons库中的默认字体文件
2.1 链接资源库(可选)
react-native link react-native-vector-icons
:::info 使用命令操作资源库的链接则不需要手动复制。 :::
2.2 使用react-native-vector-icons库自带的图标
import Icon from 'react-native-vector-icons/FontAwesome';
<Icon name="rocket" size={30} color="#900" />
2.3 在
Icon
中使用Text
组件
从而实现如下的效果:<Icon.Button name="facebook" backgroundColor="#3b5998">
<Text style={{fontFamily: 'Arial', fontSize: 15}}>Login with Facebook</Text>
</Icon.Button>
3、使用Iconfont字体
3.1 选择要使用到的自定义字体加入到自己的项目中
3.2 将项目打包下载到本地
3.3 IOS配置文件引入字体包
3.4 安卓配置文件引入字体包
Android 平台中只需将 fontello.ttf 文件放入 android/app/src/main/assets/fonts文件夹即可3.5 生成图标名称和图标Unicode编码映射文件
为了方便使用,一般是直接配置字体名称,然后组件渲染显示对应图标样式。所以这边需要生成图标映射的json文件。该文件的数据可以依据解压包文件里的iconfont.json文件里的**glyphs**
字段生成。
示例代码如下:[ { "icon_id": "20360251", "name": "打卡", "font_class": "daka", "unicode": "f226", "unicode_decimal": 61990 }, { "icon_id": "20360252", "name": "卡包", "font_class": "kabao", "unicode": "f227", "unicode_decimal": 61991 } ].forEach((x) => console.log('"' + x.font_class + '", ' + x.unicode_decimal + ','))
iconfont.json文件示例如下{ "daka": 61990, "kabao": 61991 }
3.6 创建Iconfont组件
```jsx import createIconSet from ‘react-native-vector-icons/lib/create-icon-set’; import glyphMap from ‘./iconfont.json’;
const iconSet = createIconSet(glyphMap, ‘Iconfont’, ‘iconfont.ttf’);
export default iconSet;

<a name="DGGfr"></a>
### 3.7 iconfont.json映射文件和iconfont.js组件的文件结构
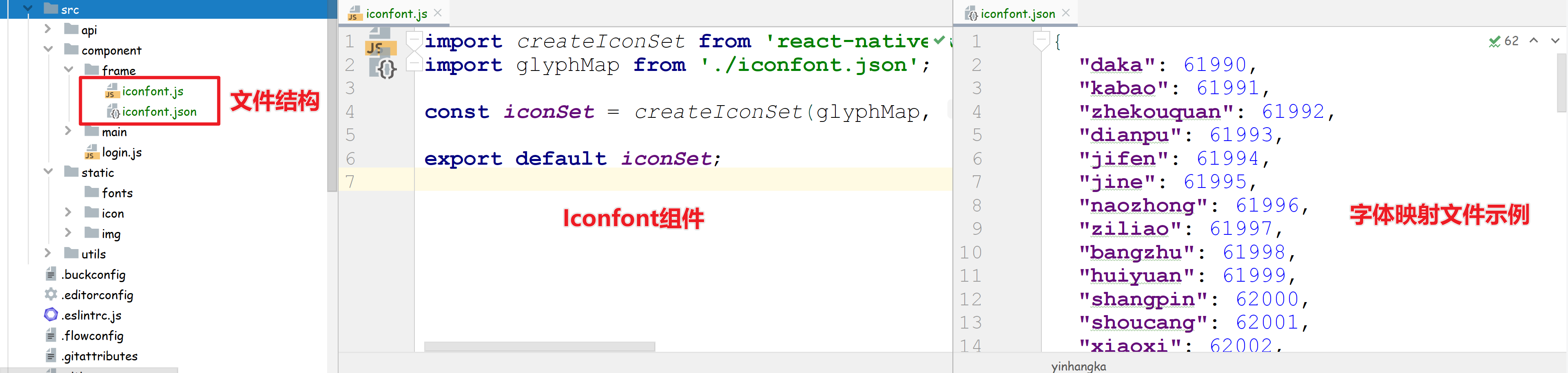
<a name="8LAF9"></a>
### 3.8 Iconfont组件的使用示例
```jsx
import React, { Component } from 'react';
import {
Text,
View,
StyleSheet,
Button,
} from 'react-native';
import Iconfont from '../common/iconfont';
export default class MyPage extends Component {
constructor(props) {
super(props);
this.state = { };
}
render() {
return (
<View style={styles.container}>
<Iconfont name="alipay" color="red" size={20} />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'pink',
},
});
:::danger 在使用时,图标的颜色,大小还需要自己设置。 :::
4、iconfont图标转换为React组件
具体使用查找其Github 的README.md描述,其特性和优势描述很具体,实用性很高。
https://github.com/iconfont-cli/react-native-iconfont-cli