静态代理
//静态代理
public class Tank implements movable{
@Override
public void move() {
System.out.println("调用了move方法");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class MoveTimeProxy implements movable{
movable m;
public MoveTimeProxy(movable m) {
this.m = m;
}
@Override
public void move() {
long start = System.currentTimeMillis();
m.move();
long end = System.currentTimeMillis();
System.out.println("运行时间:"+(end-start));
}
}
class MoveLogProxy implements movable{
movable m;
public MoveLogProxy(movable m) {
this.m = m;
}
@Override
public void move() {
System.out.println("start move");
m.move();
System.out.println("move end");
}
public static void main(String[] args) {
new MoveLogProxy(new MoveTimeProxy(new Tank())).move();
}
}
interface movable{
public void move();
}
动态代理
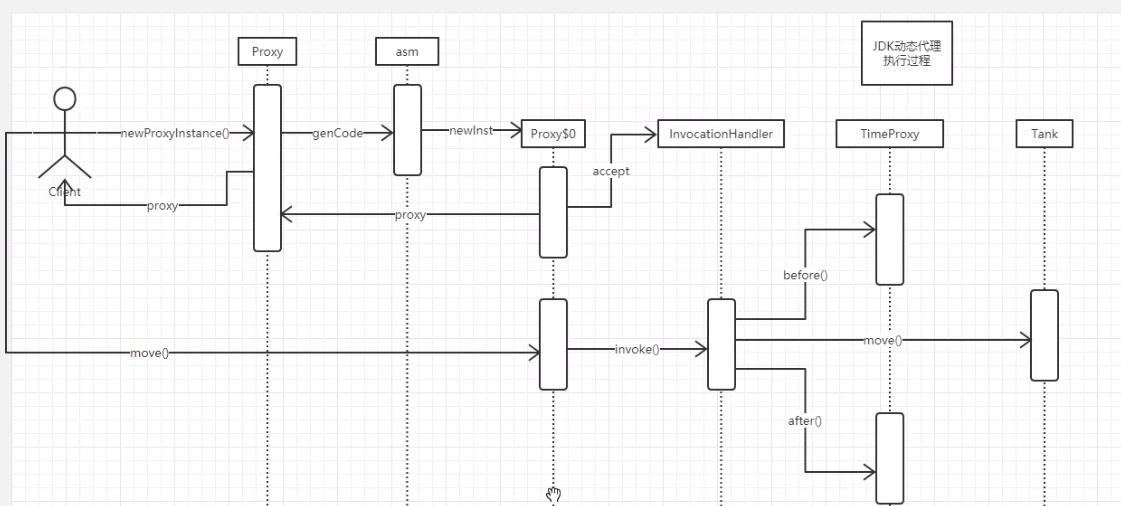
//静态代理
public class Tank implements movable{
@Override
public void move() {
System.out.println("调用了move方法");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Tank tank = new Tank();
movable movable = (movable) Proxy.newProxyInstance(
Tank.class.getClassLoader(),
new Class[]{movable.class},
new LogHander(tank));
movable.move();
}
}
class LogHander implements InvocationHandler{
Tank tank;
public LogHander(Tank tank) {
this.tank = tank;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("method:"+method.getName()+"start");
Object invoke = method.invoke(tank, args);
System.out.println("method:"+method.getName()+"end");
return invoke;
}
}
interface movable{
public void move();
}