- 赋值运算
- 算数运算
- expr 命令:只能做整数运算
- let命令:只能做整数运算,且运算元素必须是变量,无法直接对整数做运算
- 双小圆括号运算,在shell中(( ))也可以用来做数学运算
- 浮点运算是采用的命令组合的方式来实现的 echo “scale=N;表达式”|bc
- 比较运算
- !usr/bin/bash
- Filename: comparison.sh
- Author: HelloChen
- CreatTime: 2021-1-13 17:42:26
- Description: Floating point comparison
- 1、交互或者外传参的方式获得两个整数
- $1 $2
- 采用外传参的方式接收数据并放大100倍,并处理为整数
- 2、比较运算
- 3、输出结果
- 逻辑运算
- !/bin/bash
- 1、输入用户名
- echo -n “password: “
- read -s -t 5 -n 2 pw
- 2、输入密码
- 3、与运算返回结果
赋值运算
算数运算
四则运算
+ - * / % **
加、减、乘、除、取余、开方
let命令:只能做整数运算,且运算元素必须是变量,无法直接对整数做运算
let a=100+3;echo $a 103
双小圆括号运算,在shell中(( ))也可以用来做数学运算
echo $((6 / 3)) 2
浮点运算是采用的命令组合的方式来实现的 echo “scale=N;表达式”|bc
echo “scale=2;100/3”|bc
<a name="y46Yy"></a>
#### 练习1
简单的计算器
```shell
#!usr/bin/bash
#
# Filename: calculator.sh
# Author: HelloChen
# CreatTime: 2021-1-13 16:42:26
# Description: calculator
echo "$1 $2 $3"|bc
------------------------------------
# root @ Tenyun in /home/Shell [16:47:46]
$ zsh ./calculator.sh 2 + 2
4
# root @ Tenyun in /home/Shell [16:48:15]
$ zsh ./calculator.sh 2 - 2
0
# root @ Tenyun in /home/Shell [16:48:19]
$ zsh ./calculator.sh 2 \* 2
4
# root @ Tenyun in /home/Shell [16:48:22]
$ zsh ./calculator.sh 2 / 2
1
练习2
打印内存使用率
#!usr/bin/bash
#
# Filename: memory_use.sh
# Author: HelloChen
# CreatTime: 2021-1-13 16:42:26
# Description: Print memory usage
# 获取内存总量
memory_total=`free -m|grep -i "mem"|tr -s " "|cut -d " " -f2`
# 获取内存使用量
memory_use=`free -m|grep -i "mem"|tr -s " "|cut -d " " -f3`
# 计算输出
echo "内存使用率: `echo "scale=2;$memory_use*100/$memory_total"|bc`%"
比较运算
整型比较符
精确比较
-eq 等于 equal
-gt 大于
-lt 小于
模糊比较
-ge 大于或等于
-le 小于或等于
-ne 不等于
使用test命令比较两个整数的关系
# root @ Tenyun in /home/Shell [17:03:37]
$ test 100 -eq 100;echo $?
0
# root @ Tenyun in /home/Shell [17:03:46]
$ test 100 -eq 200;echo $?
1
备注:linux命令test只能比较两个整数的关系,不会返回结果,需要通过$?才能看到结果
练习3
两个整数比较大小
#!usr/bin/bash
#
# Filename: comparison.sh
# Author: HelloChen
# CreatTime: 2021-1-13 16:42:26
# Description: Comparison of size
#1、输入两个数,$1 $2
#2、判断两个数关系
if [ $1 -gt $2 ];then
#3、输出结果
echo "$1 > $2 "
elif [ $1 -eq $2 ];then
echo "$1 = $2"
else
echo "$1 < $2"
fi
-----------------------------------------
# root @ Tenyun in /home/Shell [17:30:05]
$ zsh comparison.sh 1 2
1 < 2
# root @ Tenyun in /home/Shell [17:30:19]
$ zsh comparison.sh 5 2
5 > 2
# root @ Tenyun in /home/Shell [17:30:23]
$ zsh comparison.sh 5 5
5 = 5
浮点型判断
默认情况下shell是不能判断浮点的,那么在linux中又避免不了需要进行浮点运算,那怎么解决
解决思路如下:
- 两个数据同时放大到整数倍
- 处理掉小数点位,保留整数位
- 进行整形判断
练习4
两个浮点型数比较 ```shell!usr/bin/bash
#Filename: comparison.sh
Author: HelloChen
CreatTime: 2021-1-13 17:42:26
Description: Floating point comparison
1、交互或者外传参的方式获得两个整数
$1 $2
[ $# -lt 2 ]&&echo “need two args”&&exit 1
采用外传参的方式接收数据并放大100倍,并处理为整数
num1=echo "scale=2;$1*100"|bc|cut -d "." -f1
num2=echo "scale=2;$2*100"|bc|cut -d "." -f1
2、比较运算
if [ $num1 -gt $num2 ];then
3、输出结果
echo “$1 > $2” elif [ $num1 -lt $num2 ];then echo “$1 < $2” else echo “$1 = $2” fi
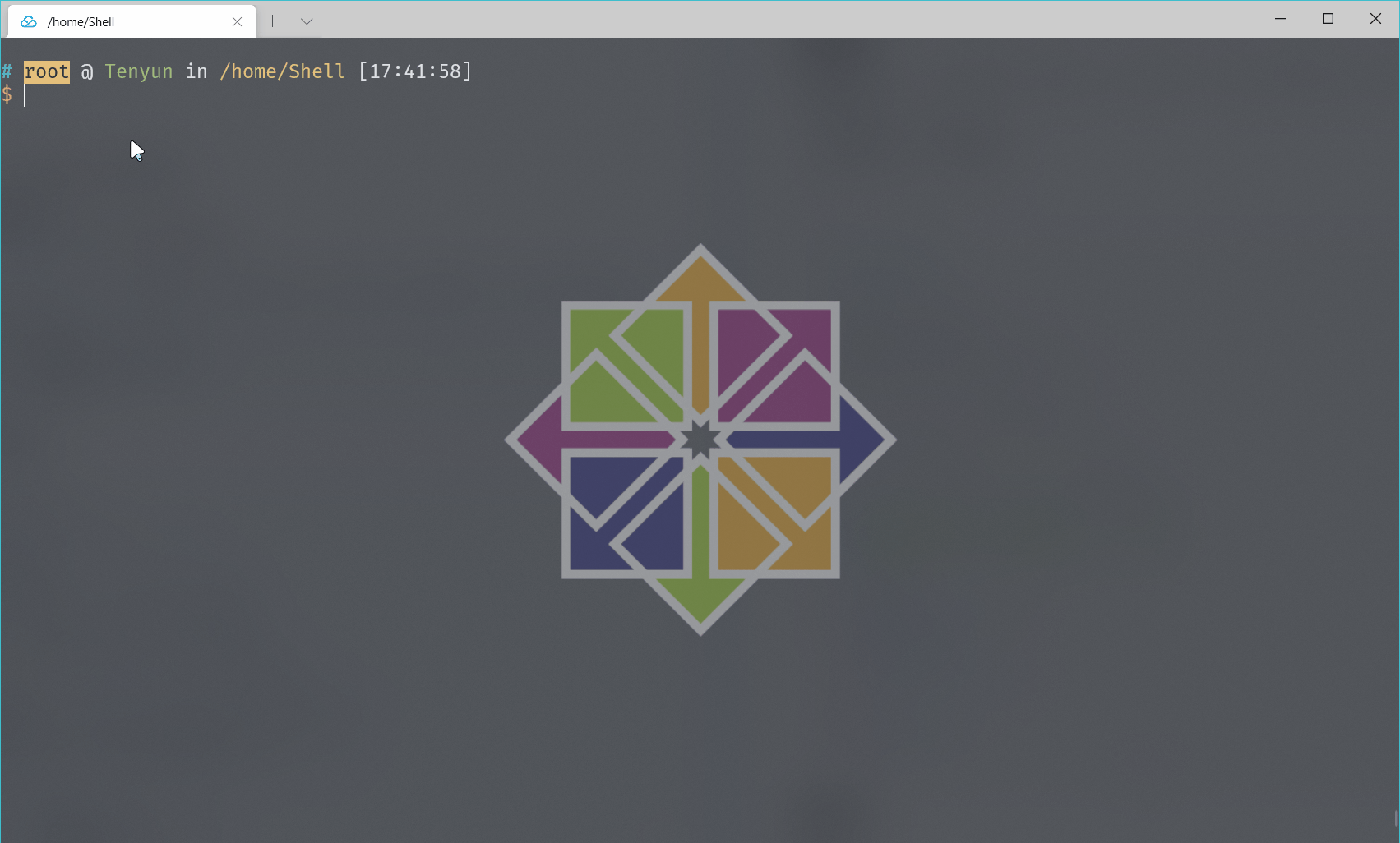
<a name="lsFnp"></a>
### 字符串比较运算
运算符解释,注意字符串一定别忘了使用引号引起来<br /> == 等于 <br /> != 不等于<br /> -n 检查字符串的长度是否大于0 <br /> -z 检查字符串的长度是否为0
```shell
# root @ Tenyun in /home/Shell [17:47:44] C:1
$ test 'root' \=\= 'root';echo $?
0
# root @ Tenyun in /home/Shell [17:47:48]
$ test 'root' \!= 'root';echo $?
1
# root @ Tenyun in /home/Shell [17:48:06]
$ name=
# root @ Tenyun in /home/Shell [17:48:24]
$ test -n "$name";echo $?
1
# root @ Tenyun in /home/Shell [17:48:46]
$ test -z "$name";echo $?
0
练习5
模拟一个linux文本界面登陆程序,要求账号密码验证成功进入系统,账号密码验证失败退回登陆界面
#!usr/bin/bash
#
# Filename: check_login.sh
# Author: HelloChen
# CreatTime: 2021-1-13 17:42:26
# Description: check login
####
default_account='root'
default_pw='123456'
######main
#1、清屏
clear
#2、输出提示信息
echo "CentOS Linux 8 (Core)"
echo -e "Kernel `uname -r` on an `uname -m`\n"
#3、交互输入登陆名
echo -n "$HOSTNAME login: "
read account
#4、交互输入密码
read -s -t30 -p "Password: " pw
#5、判断用户输入是否正确
if [ "$default_account" == "$account" ] && [ "$default_pw" == "$pw" ];then
clear
echo -e "\nwelcome to root"
else
echo "用户名或密码错误..."
#输入错误,再次调用本脚本
sh $0
fi
逻辑运算
- 逻辑与运算 &&
- 逻辑或运算 ||
- 逻辑非运算 !
练习6
使用逻辑运算写一个仿真用户登录验证程序 ```shell!/bin/bash
echo “CentOS linux 8 (Core)” echo -e “Kerneluname -r
on anuname -m
\n”
1、输入用户名
echo -n “$HOSTNAME login: “ read myuser
echo -n “password: “
read -s -t 5 -n 2 pw
2、输入密码
read -p “password: “ -s -t 5 -n 6 pw
3、与运算返回结果
[ $myuser == ‘root’ ] && [ $pw == ‘123456’ ] && echo yes || echo no ```