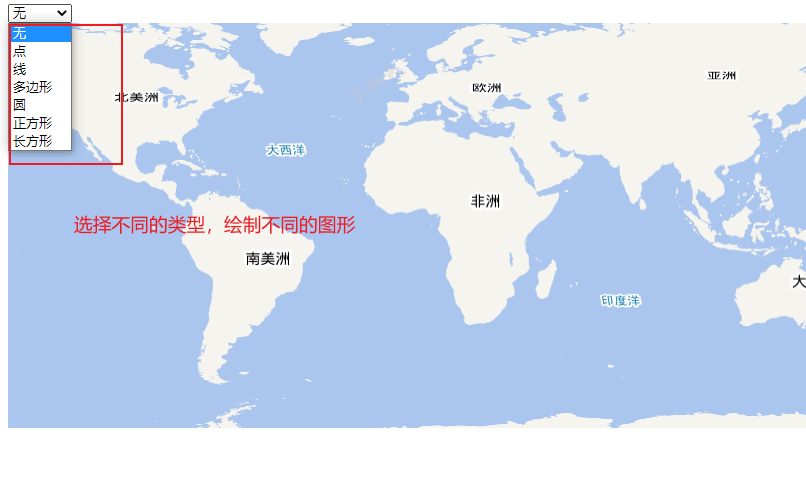
一、封装画笔
//如何是绘制正方方和矩形需要特殊处理
/*
@param{string}source 待添加的数据源
@param{string} type 不同的类型(Point,LineString,Polygon,Circle,Square,Box)
@param{function} callback 绘制完成调用
*/
//draw.js
function createDraw({source, type="Point", callback}) {
let draw = null
let geometryFunction = null
let maxPoints = 0
if (type == 'Square') {
type = 'Circle'
geometryFunction = ol.interaction.Draw.createRegularPolygon(4)
} else if (type == 'Rectangle') {
type = 'LineString'
geometryFunction = function (coordinates, geometry) {
if (!geometry) {
//多边形
geometry = new ol.geom.Polygon(null)
}
var start = coordinates[0]
var end = coordinates[1]
geometry.setCoordinates([
[start,[start[0],end[1]],end,[end[0],start[1]],start]
])
return geometry
}
maxPoints = 2
}
draw = new ol.interaction.Draw({
source: source,
type: type,
geometryFunction: geometryFunction,
maxPoints: maxPoints,
})
// callback && draw.on('drawend', callback)
if (callback) {
draw.on('drawend', callback)
}
return draw
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="../../lib/include-openlayers-local.js"></script>
<script src="../../js/Tian.js"></script>
<script src="../../js/Draw.js"></script>
</head>
<body>
<select id="select" onchange="handleChange(event)">
<option value="None" selected="selected">无</option>
<option value="Point">点</option>
<option value="LineString">线</option>
<option value="Polygon">多边形</option>
<option value="Circle">圆</option>
<option value="Square">正方形</option>
<option value="Box">长方形</option>
</select>
<div id="map_container"></div>
<script>
const map = new ol.Map({
target:"map_container",
layers:[TianDiMap_vec,TianDiMap_cva],
view:new ol.View({
center:[114,30],
projection:"EPSG:4326",
zoom:4
})
})
function handleChange(e){
var type = e.target.value;
}
</script>
</body>
</html>