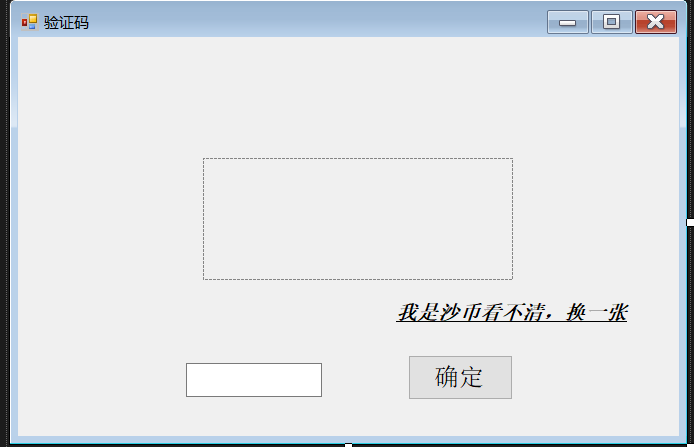
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _127_绘制验证码
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//用来记录验证码中的数字
string correct = null;
/// <summary>
/// 点击PictureBox时验证码更换
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void pictureBoxCode_Click(object sender, EventArgs e)
{
Random r = new Random();
string strCode = null;
for (int i = 0; i < 5; i++)
{
int rNumber = r.Next(0, 10);
strCode += rNumber;
}
//将正确数字记录下来
correct = strCode;
//创建随机数、干扰线和像素点的载体
//创建GDI对象 (画图像)
Bitmap bmp = new Bitmap(200, 50); //创建一个位图对象
Graphics g = Graphics.FromImage(bmp);
//将随机数画入bmp中
for (int i = 0; i < 5; i++)
{
//字体,颜色,样式要求全随机
string[] fonts = { "宋体", "微软雅黑", "幼圆", "华文行楷", "方正舒体", "华文彩云", "楷体" };
Color[] colors = { Color.Yellow, Color.Red, Color.Green, Color.Blue, Color.Black, Color.Violet, Color.Orange, Color.Azure };
FontStyle[] fStyle = { FontStyle.Regular, FontStyle.Bold, FontStyle.Italic, FontStyle.Underline, FontStyle.Strikeout };
//五个数字的横坐标不能相同,纵坐标可以
Point point = new Point(i * 30, 0);//在PictureBox中的坐标
//画string DrawString(str,font,brush,point)
//DrawString()方法需要 string font brush point四个参数 (Font需要字体,大小,类型三个参数) //定义一种颜色的画笔,这个方法可以将color传入
g.DrawString(strCode[i].ToString(), new Font(fonts[r.Next(0, fonts.Length)], r.Next(20, 55), fStyle[r.Next(0, fStyle.Length)]), new SolidBrush(colors[r.Next(0, colors.Length)]), point);
}
//画干扰线
for (int i = 0; i < 70; i++)
{
Color[] colors = { Color.Yellow, Color.Red, Color.Green, Color.Blue, Color.Black, Color.Violet, Color.Orange, Color.Azure };
Pen pen = new Pen(colors[r.Next(0, colors.Length)]);
Point p1 = new Point(r.Next(0, bmp.Width), r.Next(0, bmp.Height));
Point p2 = new Point(r.Next(0, bmp.Width), r.Next(0, bmp.Height));
g.DrawLine(pen, p1, p2);
}
//生成像素粒
for (int i = 0; i < 500; i++)
{
Point p = new Point(r.Next(0, bmp.Width), r.Next(0, bmp.Height));
Color[] colors = { Color.Yellow, Color.Red, Color.Green, Color.Blue, Color.Black, Color.Violet, Color.Orange, Color.Azure };
//在此Bitmap中设置指定像素的颜色
bmp.SetPixel(p.X, p.Y, colors[r.Next(0, colors.Length)]);
}
//将图片镶嵌到PictureBox中
pictureBoxCode.Image = bmp;
}
/// <summary>
/// 点击时,判断验证码是否与用户输入的相同
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btnEnter_Click(object sender, EventArgs e)
{
if (txtInput.Text == correct)
{
MessageBox.Show("输入正确");
Object ob = new object();
EventArgs ea = new EventArgs();
pictureBoxCode_Click(ob, ea);
}
else
{
MessageBox.Show("输入错误,沙币");
}
//输入后清空文本
txtInput.Clear();
txtInput.Focus();
}
private void label1_Click(object sender, EventArgs e)
{
Object ob = new object();
EventArgs ea = new EventArgs();
pictureBoxCode_Click(ob, ea);
}
private void Form1_Load(object sender, EventArgs e)
{
Object ob = new object();
EventArgs ea = new EventArgs();
pictureBoxCode_Click(ob, ea);
}
}
}