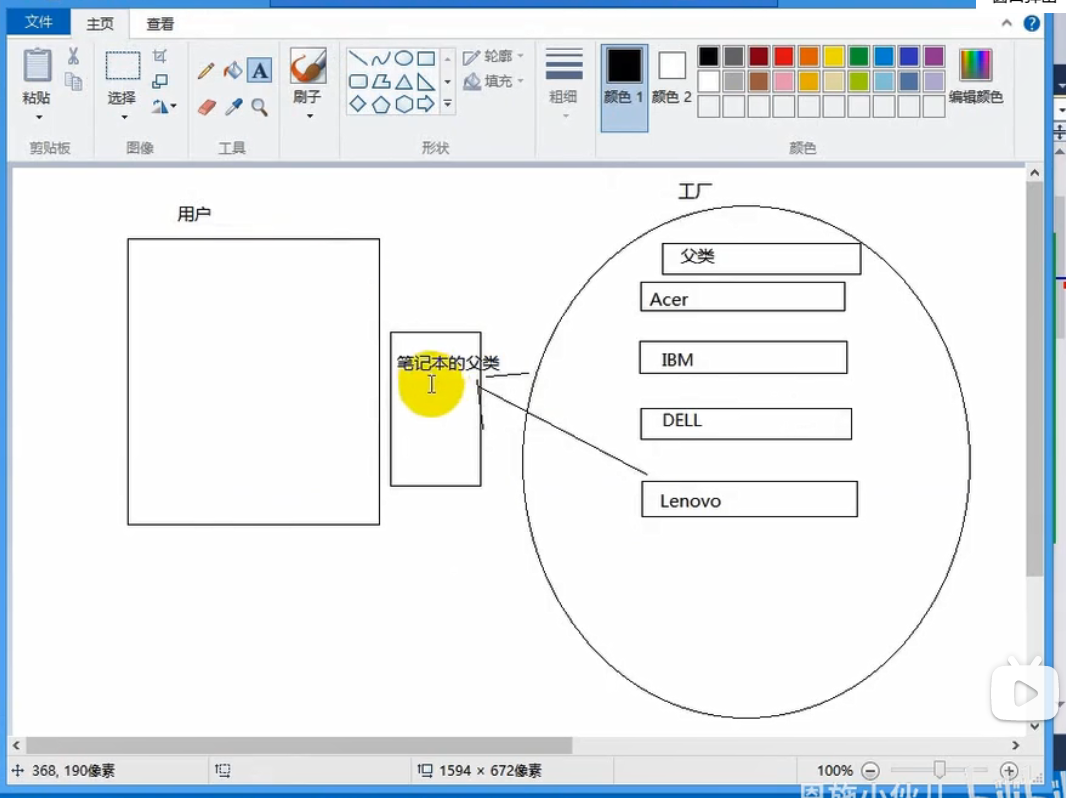
using System;
namespace _086_简单工厂设计模式
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入你想要的笔记本");
string brand = Console.ReadLine();
NoteBook nb = GetNoteBook(brand);
nb.SayHello();
Console.ReadKey();
}
/// <summary>
/// 简单工厂的核心,根据用户的输入创建对象赋值给父类
/// </summary>
/// <param name="brand">用户的输入</param>
/// <returns>父类</returns>
public static NoteBook GetNoteBook(string brand)
{
NoteBook nb = null;
switch (brand)
{
case "Lenovo":
nb = new Lenovo();
break;
case "Acer":
nb = new Acer();
break;
case "Dell":
nb = new Dell();
break;
case "IBM":
nb = new IBM();
break;
default:
break;
}
return nb;
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace _086_简单工厂设计模式
{
abstract class NoteBook
{
public abstract void SayHello();
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace _086_简单工厂设计模式
{
class Lenovo : NoteBook
{
public override void SayHello()
{
Console.WriteLine("我是联想笔记本");
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace _086_简单工厂设计模式
{
class Acer : NoteBook
{
public override void SayHello()
{
Console.WriteLine("我是鸿基笔记本");
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace _086_简单工厂设计模式
{
class Dell : NoteBook
{
public override void SayHello()
{
Console.WriteLine("我是戴尔笔记本");
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace _086_简单工厂设计模式
{
class IBM : NoteBook
{
public override void SayHello()
{
Console.WriteLine("我是IBM笔记本");
}
}
}