Text
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Text widget',
home: Scaffold(
appBar: AppBar(
title: Text('哈利波特')
),
body: Center(
child: Text(
'哈利波特一共有八部,而我在2月份看了八部,里面哈利波特的勇敢,赫敏的睿智都给我留下了很深的印象。',
textAlign: TextAlign.center,
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
fontSize: 24.0,
color: Color.fromARGB(255, 255, 100, 100),
decoration: TextDecoration.underline,
decorationStyle: TextDecorationStyle.dashed,
),
),
),
),
);
}
}
Container
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Text Widget',
home: Scaffold(
appBar: AppBar(
title: Text('暮光之城'),
),
body: Center(
child: Container(
child: Text('暮光破晓', style: TextStyle(fontSize: 26.0, color: Color.fromARGB(255, 0, 0, 50))),
alignment: Alignment.topLeft,
width: 500.0,
height: 400.0,
// color: Colors.lightBlue,
// padding: const EdgeInsets.all(10.0),
padding: const EdgeInsets.fromLTRB(10.0, 100.0, 0.0, 0.0),
margin: const EdgeInsets.all(10.0),
decoration: new BoxDecoration(
gradient: const LinearGradient(
colors: [Colors.lightBlue, Colors.greenAccent, Colors.purple]
),
border: Border.all(width: 5.0, color: Colors.red)
),
),
),
),
);
}
}
在decoration下加渐变色、边框等。
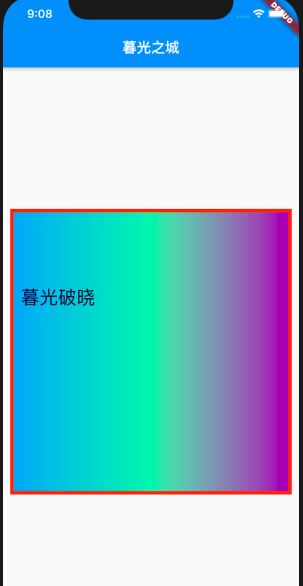
Image
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: '应用描述',
home: Scaffold(
appBar: AppBar(
title: Text('光明与黑暗')
),
body: Center(
child: Container(
child: new Image.netWork(
'图片链接',
fit: BoxFit.cover,
color: Colors.green,
colorBlendMode: BlendMode.darken,
repeat: ImageRepeat.noRepeat,
),
width: 300.0,
height: 500.0,
color: Colors.green,
),
),
),
);
}
}
ListView
import 'package:flutter/material.dart';
void main() => runApp(MyApp(
items: List<String>.generate(1000, (i) => "I love you $i")
));
class MyApp extends StatelessWidget {
final List<String> items;
MyApp({Key key, @required this.items}):super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: '爱丽丝',
home: Scaffold(
appBar: AppBar(
title: Text('爱丽丝'),
),
body: ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text('${items[index]}')
);
}
),
)
);
}
}
基础的路由跳转
import "package:flutter/material.dart";
void main() => runApp(MaterialApp(
title: '基础导航练习',
home: FirstScreen()
));
class FirstScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('商品页')),
body: Center(
child: RaisedButton(
child: Text('查看商品详情页'),
onPressed: () {
Navigator.push(context, MaterialPageRoute(builder: (context) => SecondScreen()));
},
),
),
);
}
}
class SecondScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('商品详情页')),
body: Center(
child: RaisedButton(
child: Text('返回到商品页'),
onPressed: () {
Navigator.pop(context);
},
)
),
);
}
}
通过route name跳转
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
initialRoute: '/',
routes: {
'/': (context) => FirstScreen(),
'/second': (context) => SecondScreen(),
},
));
class FirstScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('/匹配的是第一页')),
body: Center(
child: RaisedButton(
child: Text('/'),
onPressed: () {
Navigator.pushNamed(context, '/second');
},
)
)
);
}
}
class SecondScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('/second匹配的是第二页')),
body: Center(
child: RaisedButton(
child: Text('go back'),
onPressed: () {
Navigator.pop(context);
},
)
),
);
}
}
比如在app中许多地方都要导航到同一个页面,基础路由跳转会导致代码重复。解决方法就是 named routes。
页面跳转且传递数据
import 'package:flutter/material.dart';
class Todo {
final String title;
final String description;
Todo(this.title, this.description);
}
void main() => runApp(MaterialApp(
title: '传数据',
// todos 是传递给TodoScreen 的参数
home: TodoScreen(
todos: List.generate(20, (i) => Todo('Todo $i', 'A description of what needs to be done for Todo $i',))
),
));
class TodoScreen extends StatelessWidget {
final List<Todo> todos; // 接收参数 todos
TodoScreen({Key key, @required this.todos}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Todos')),
body: ListView.builder(
itemCount: todos.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(todos[index].title),
onTap: () {
Navigator.push(context, MaterialPageRoute(
builder: (context) => DetailScreen(todo: todos[index]) // 给 详情页传递数据 todo
));
},
);
}
),
);
}
}
class DetailScreen extends StatelessWidget {
final Todo todo; // 接收参数 todo
DetailScreen({Key key, @required this.todo}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(todo.title)),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Text(todo.description),
),
);
}
}
页面跳转并返回数据
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
title: 'Returning Data',
home: HomeScreen(),
));
}
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Returning Data Demo'),
),
body: Center(child: SelectionButton()),
);
}
}
class SelectionButton extends StatelessWidget {
@override
Widget build(BuildContext context) {
return RaisedButton(
child: Text('Pick an option, any option!'),
onPressed: () {
_navigateAndDisplaySelection(context);
},
);
}
// A method that launches the SelectionScreen and awaits the result from
// Navigator.pop.
_navigateAndDisplaySelection(BuildContext context) async {
// Navigator.push returns a Future that completes after calling
// Navigator.pop on the Selection Screen.
final result = await Navigator.push(
context,
MaterialPageRoute(builder: (context) => SelectionScreen()),
);
// After the Selection Screen returns a result, hide any previous snackbars
// and show the new result.
Scaffold.of(context)
..removeCurrentSnackBar()
..showSnackBar(SnackBar(content: Text("$result")));
}
}
class SelectionScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Pick an option'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(8.0),
child: RaisedButton(
onPressed: () {
// Close the screen and return "Yep!" as the result.
Navigator.pop(context, 'Yep!');
},
child: Text('Yep!'),
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: RaisedButton(
onPressed: () {
// Close the screen and return "Nope!" as the result.
Navigator.pop(context, 'Nope.');
},
child: Text('Nope.'),
),
)
],
),
),
);
}
}