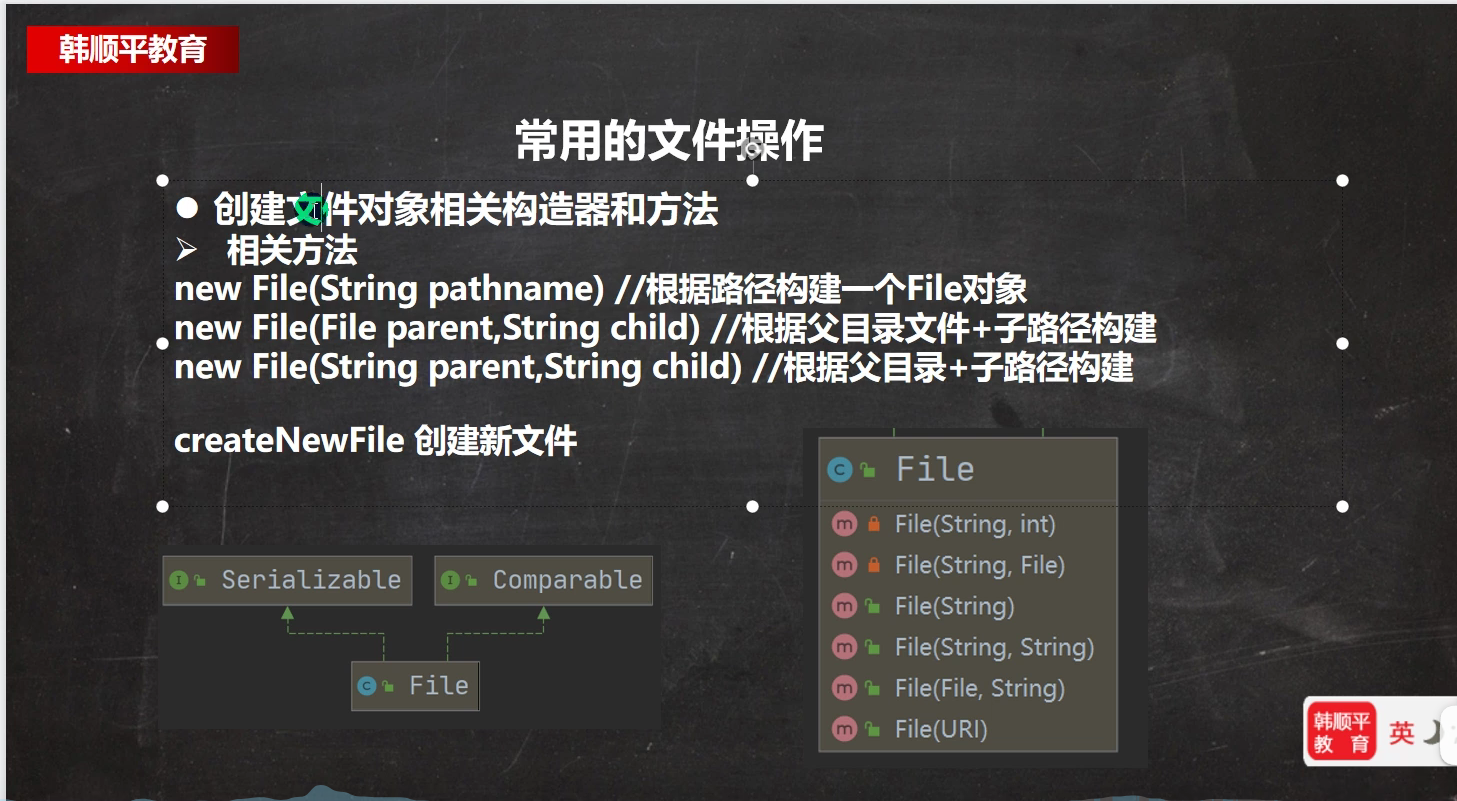
import org.junit.Test;
import java.io.File;
import java.io.IOException;
public class iofile {
public static void main(String[] args) {
}
@Test
public void creat01(){
File parentFile=new File("d:\\");
String fileName="news1.text";
File file = new File(parentFile,fileName);
try {
file.createNewFile();
System.out.println("创建成功");
} catch (IOException e) {
e.printStackTrace();
}
}
@Test
public void creat02(){
String filePath="d:\\new2.txt";
File file = new File(filePath);
try {
file.createNewFile();
System.out.println("文件创建成功");
} catch (IOException e) {
e.printStackTrace();
}
}
@Test
public void creat03(){
String parentFile="d:\\";
String fileName="text03.txt";
File file = new File(parentFile,fileName);
try {
file.createNewFile();
System.out.println("文件创建成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
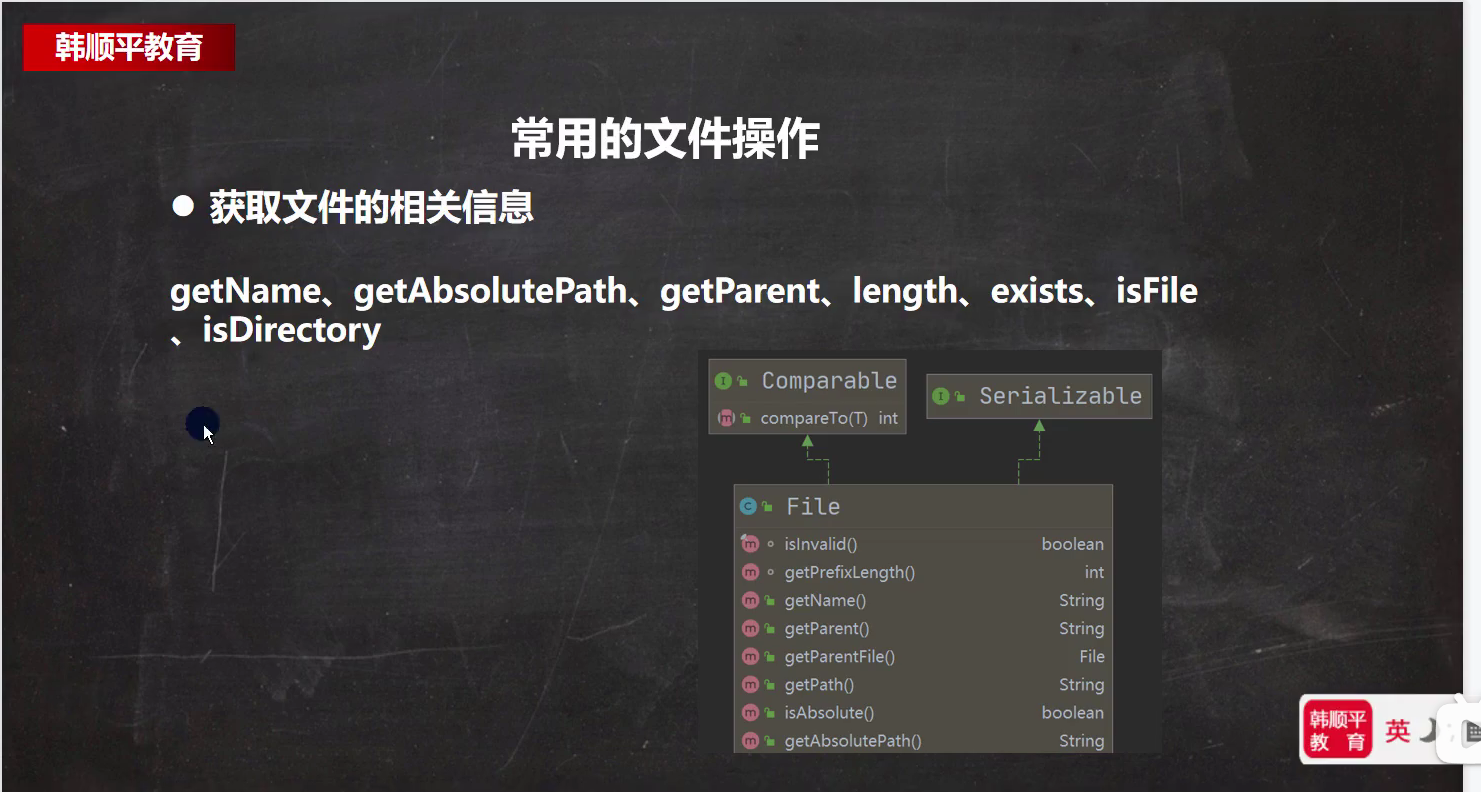
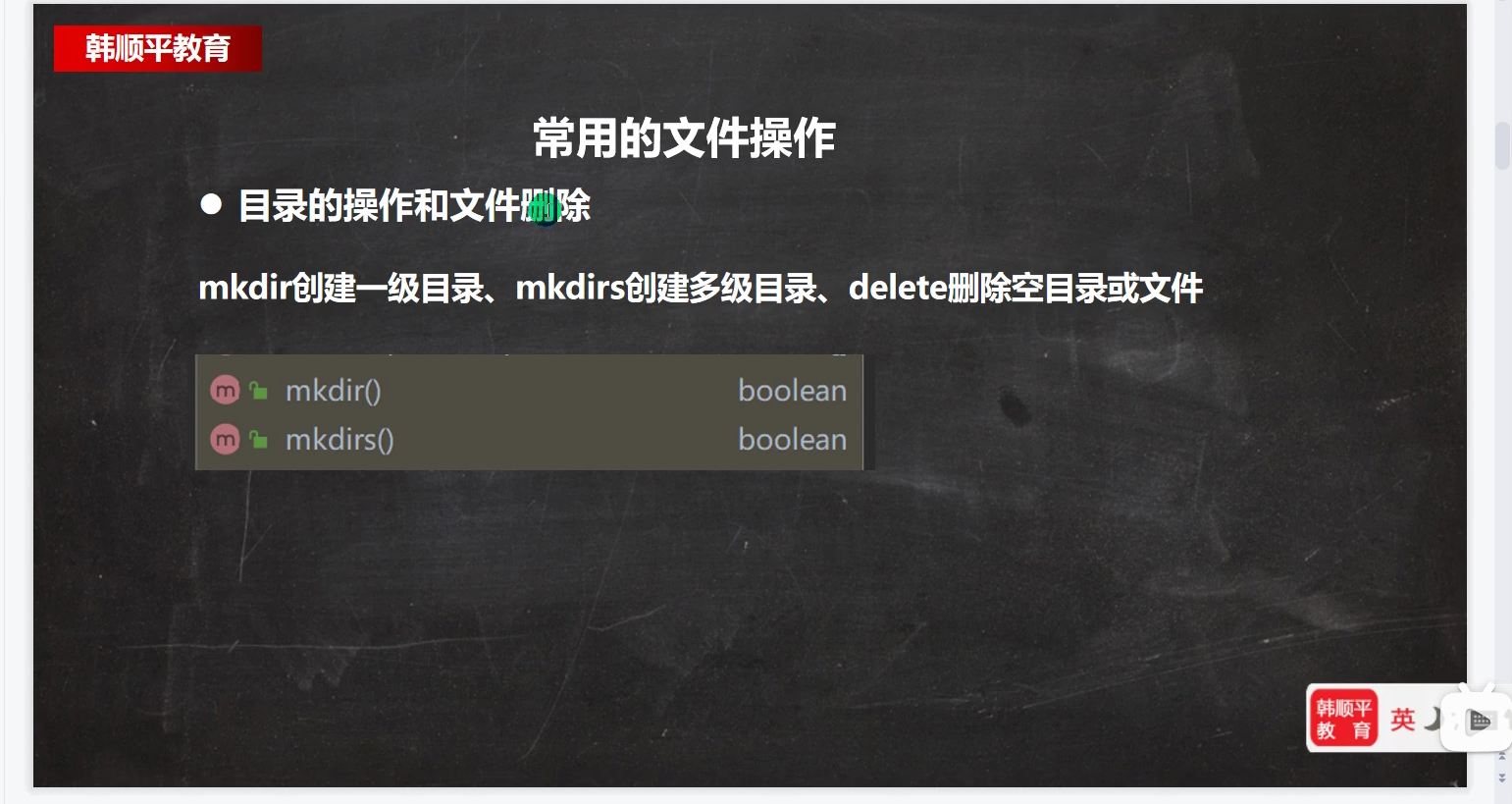

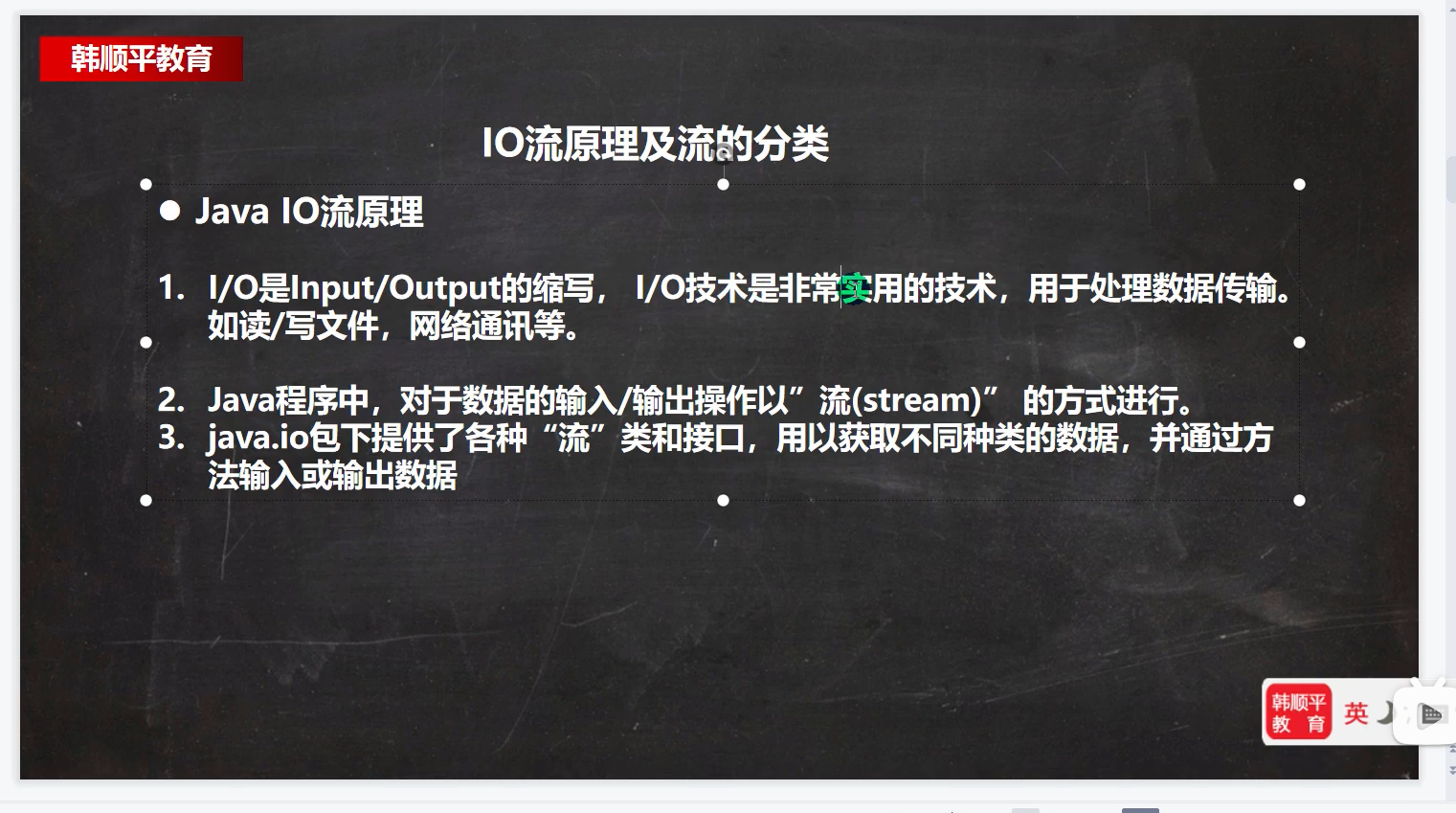
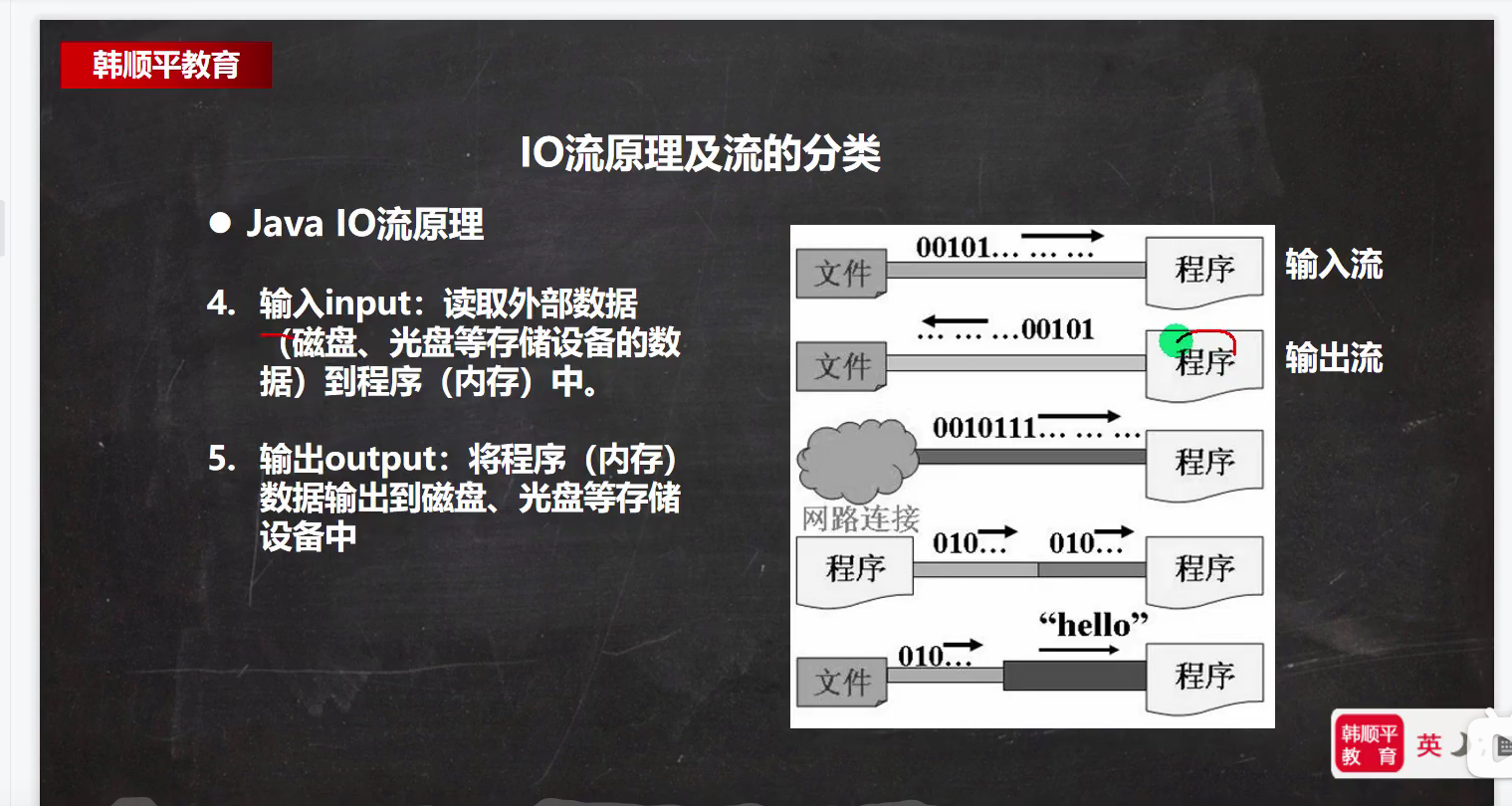
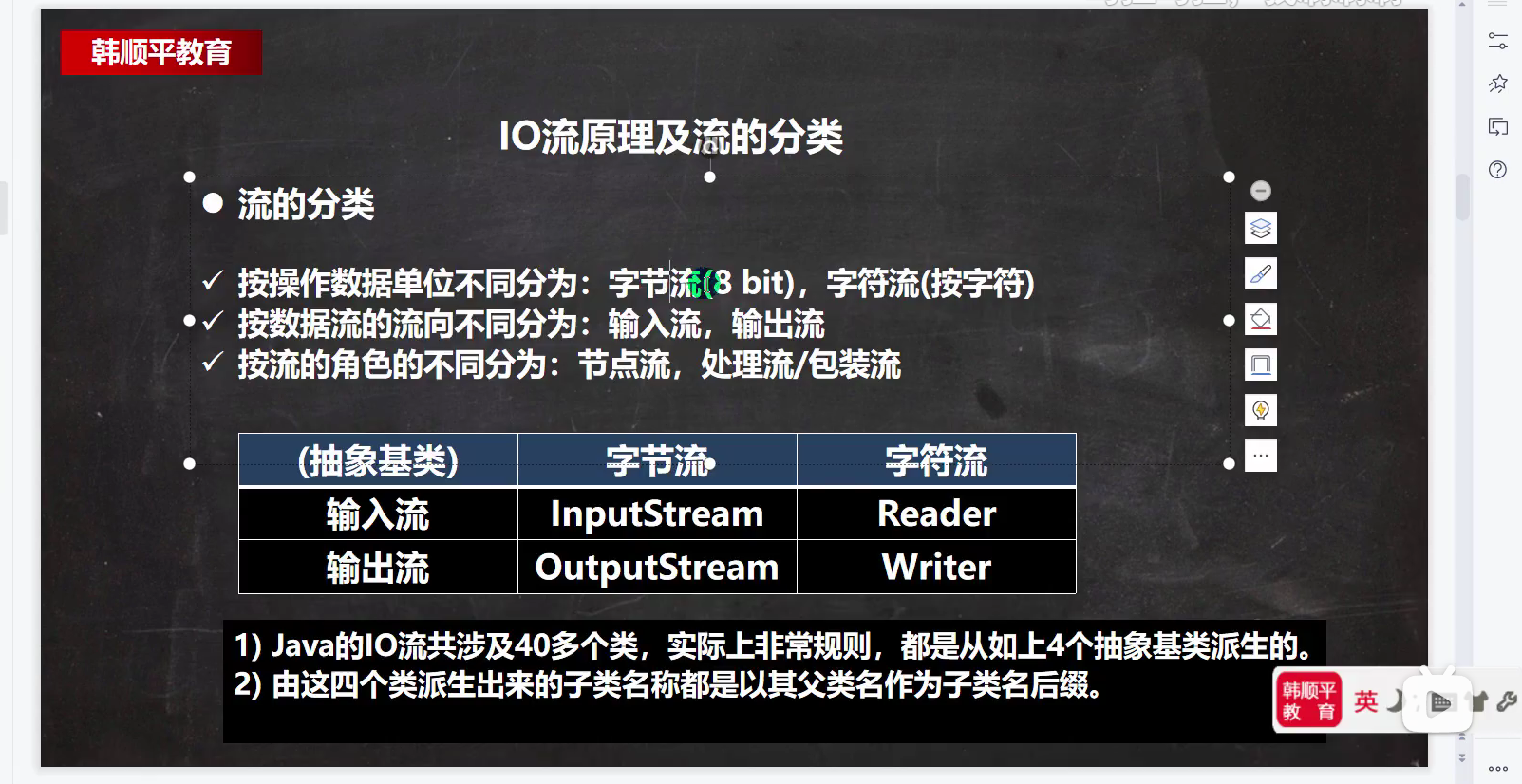
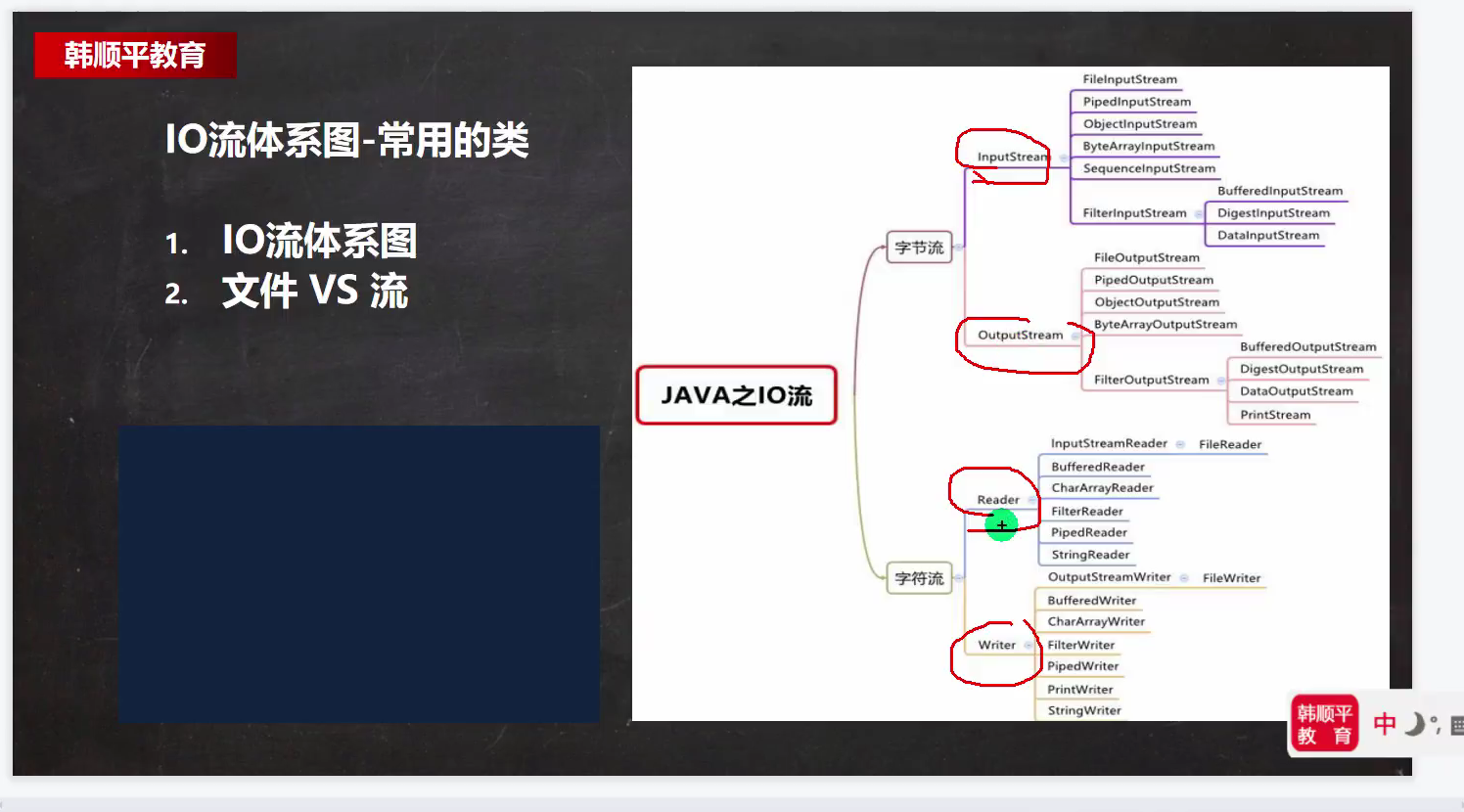
csdn上面的讲解
链接
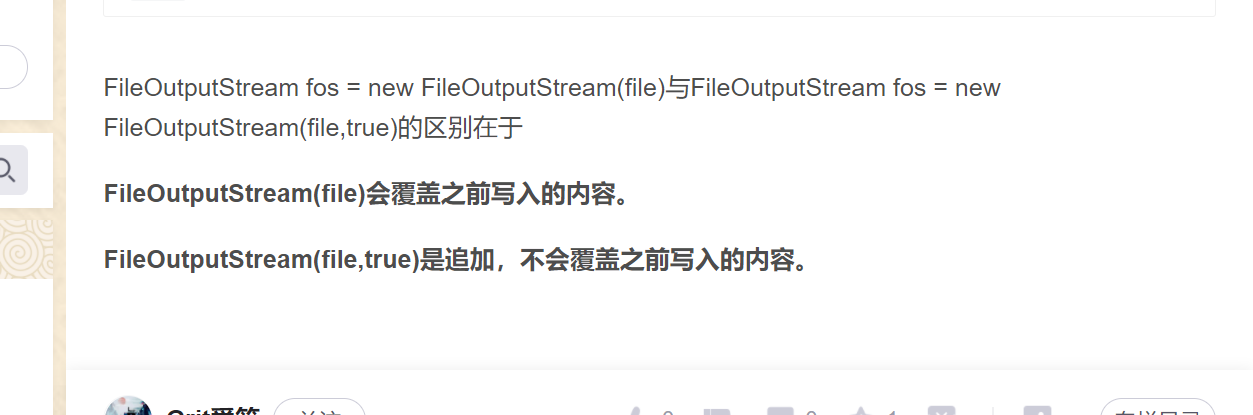
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class IoTest {
//字节流
public static void main(String[] args) throws IOException {
File file1 = new File("D:/test1.txt");
write(file1);
System.out.println(read(file1));
}
public static void write(File file)throws IOException {
FileOutputStream os = new FileOutputStream(file,true);
String s="人家最喜欢惠祁啦!";
os.write(s.getBytes());
os.close();
}
public static String read(File file)throws IOException{
FileInputStream fs = new FileInputStream(file);
byte[]bytes=new byte[1024];
StringBuilder sb = new StringBuilder();
int length;
while ((length=fs.read(bytes))!=-1){
sb.append(new String(bytes,0,length));
}
fs.close();
return sb.toString();
}
}
//缓冲字节流
public class IOTest01 {
public static void main(String[] args) throws IOException {
File file = new File("D:/test2.txt");
write(file);
System.out.println(read(file));
}
public static void write(File file)throws IOException {
BufferedOutputStream bs = new BufferedOutputStream(new FileOutputStream(file,true));
String s="垃圾惠祁垃圾惠祁!";
bs.write(s.getBytes());
bs.close();
}
public static String read(File file)throws IOException{
BufferedInputStream fs = new BufferedInputStream(new FileInputStream(file));
byte [] bytes=new byte[1024];
//用来接收读取的字节数组
StringBuilder sb=new StringBuilder();
//读取到字节数组的长度
int length;
while ((length=fs.read(bytes))!= -1){
//将读取到的内容转化为字符串
sb.append(new String(bytes,0,length));
}
fs.close();
return sb.toString();
}
}
//字符流
public class IOTest03 {
public static void main(String[] args)throws IOException {
File file = new File("D:/test03.txt");
write(file);
System.out.println(read(file));
}
public static void write(File file) throws IOException {
OutputStreamWriter ow = new OutputStreamWriter(new FileOutputStream(file, true));
String s = "io流好麻烦呀1";
ow.write(s);
ow.close();
}
public static String read(File file)throws IOException{
InputStreamReader ir = new InputStreamReader(new FileInputStream(file), "UTF-8");
//一起读取多少给字符
char[]chars=new char[1024];
//读取的字符数组先append到StringBuilder里面
StringBuilder sb=new StringBuilder();
int length;
while ((length= ir.read(chars))!= -1){
sb.append(new String(chars,0,length));
}
ir.close();
return sb.toString();
}
}
import java.io.*;
import java.nio.charset.StandardCharsets;
//字符流
public class IOTest03 {
public static void main(String[] args)throws IOException {
File file = new File("D:/test03.txt");
write(file);
System.out.println(read(file));
}
public static void write(File file) throws IOException {
OutputStreamWriter ow = new OutputStreamWriter(new FileOutputStream(file, true));
String s = "io流好麻烦呀1";
ow.write(s);
ow.close();
}
public static String read(File file)throws IOException{
InputStreamReader ir = new InputStreamReader(new FileInputStream(file), "UTF-8");
//一起读取多少给字符
char[]chars=new char[1024];
//读取的字符数组先append到StringBuilder里面
int length;
while ((length= ir.read(chars))!= -1){
System.out.println(new String(chars,0,length));
}
ir.close();
return new String();
}
///字符流便捷类
public class IOTest02 {
public static void main(String[] args)throws IOException {
File file = new File("D:/test04.txt");
write(file);
System.out.println(read(file));
}
public static void write(File file)throws IOException {
FileWriter fw = new FileWriter(file, true);
String s="慢慢学一定可以的!";
fw.write(s);
fw.close();
}
public static String read(File file)throws IOException{
FileReader fileReader = new FileReader(file);
char [] chars= new char[1024];
StringBuilder sb=new StringBuilder();
int length;
while ((length=fileReader.read(chars)) != -1){
sb.append(new String(chars,0,length));
}
fileReader.close();
return sb.toString();
}
}
文件的拷贝
import com.sun.xml.internal.ws.policy.privateutil.PolicyUtils;
import java.io.*;
//文件拷贝
public class IOTest04 {
public static void main(String[] args) throws IOException {
{
try {
FileInputStream fi = new FileInputStream("D:/test2.txt");
FileOutputStream fo = new FileOutputStream("D:/test03.txt",true);
byte[] bytes = new byte[1024];
StringBuilder stringBuilder = new StringBuilder();
int length;
while ((length = fi.read(bytes)) != -1) {
fo.write(bytes, 0, length);
}
fi.close();
fo.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
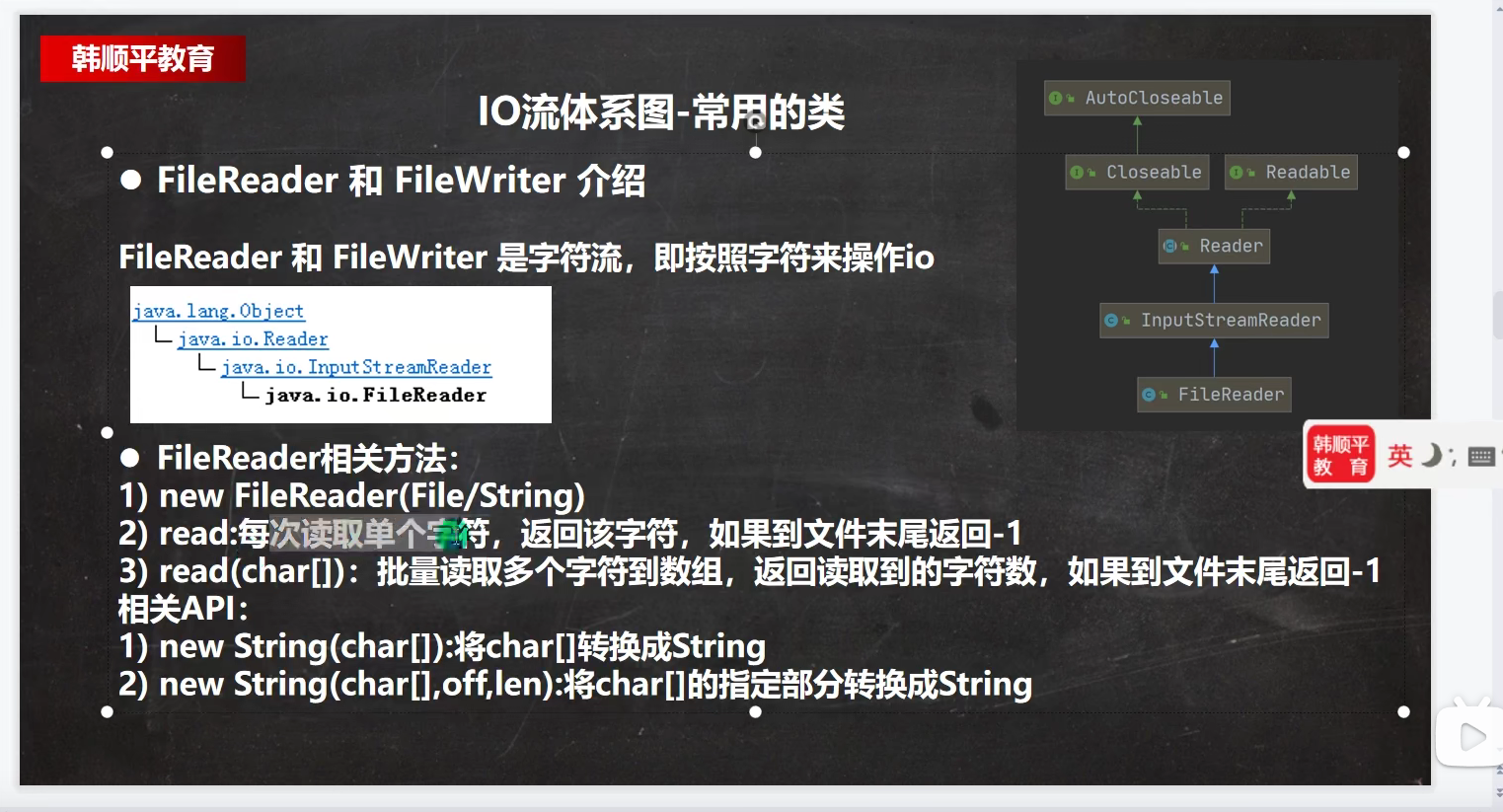
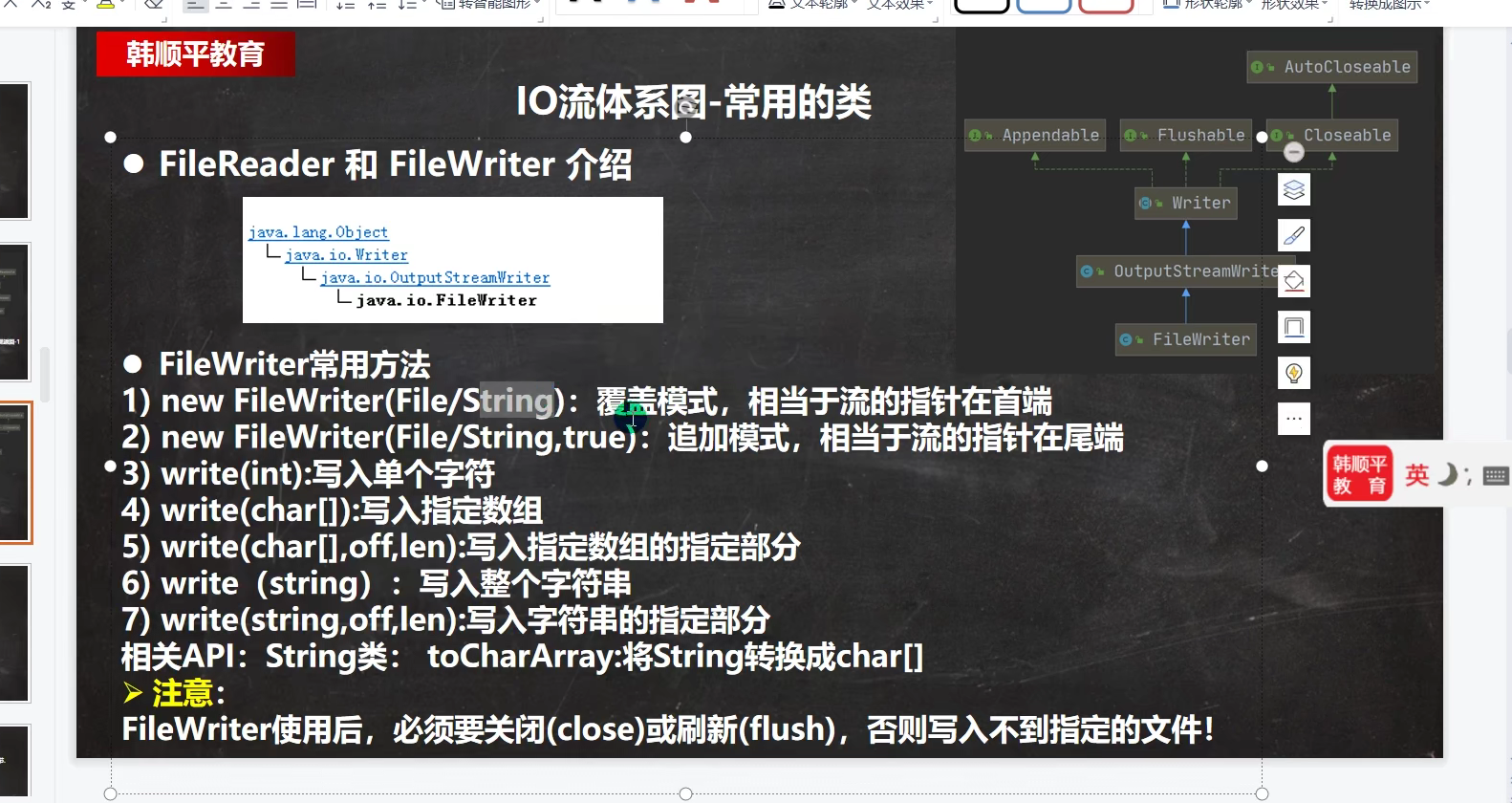