MAP
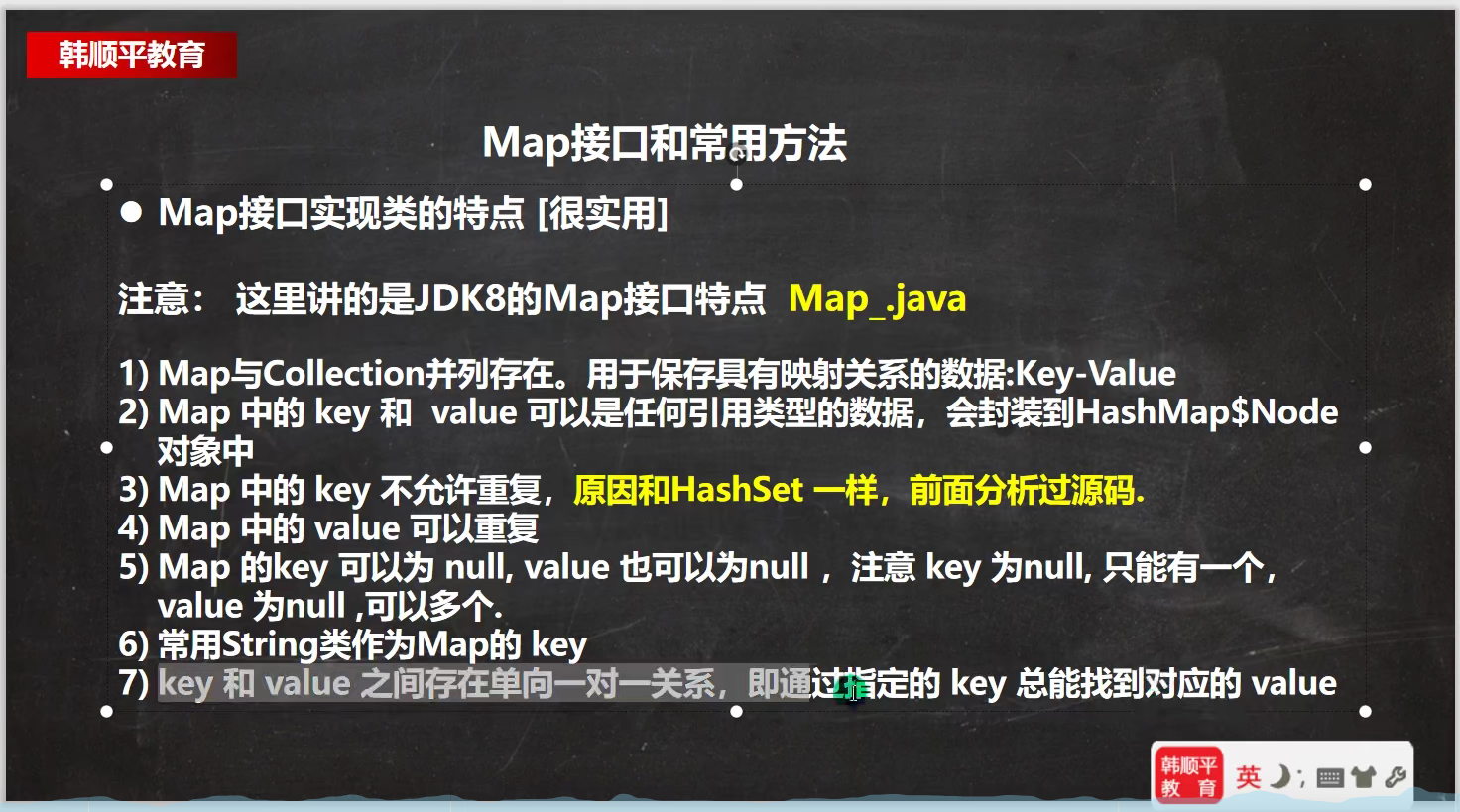
MAP接口的常用方法
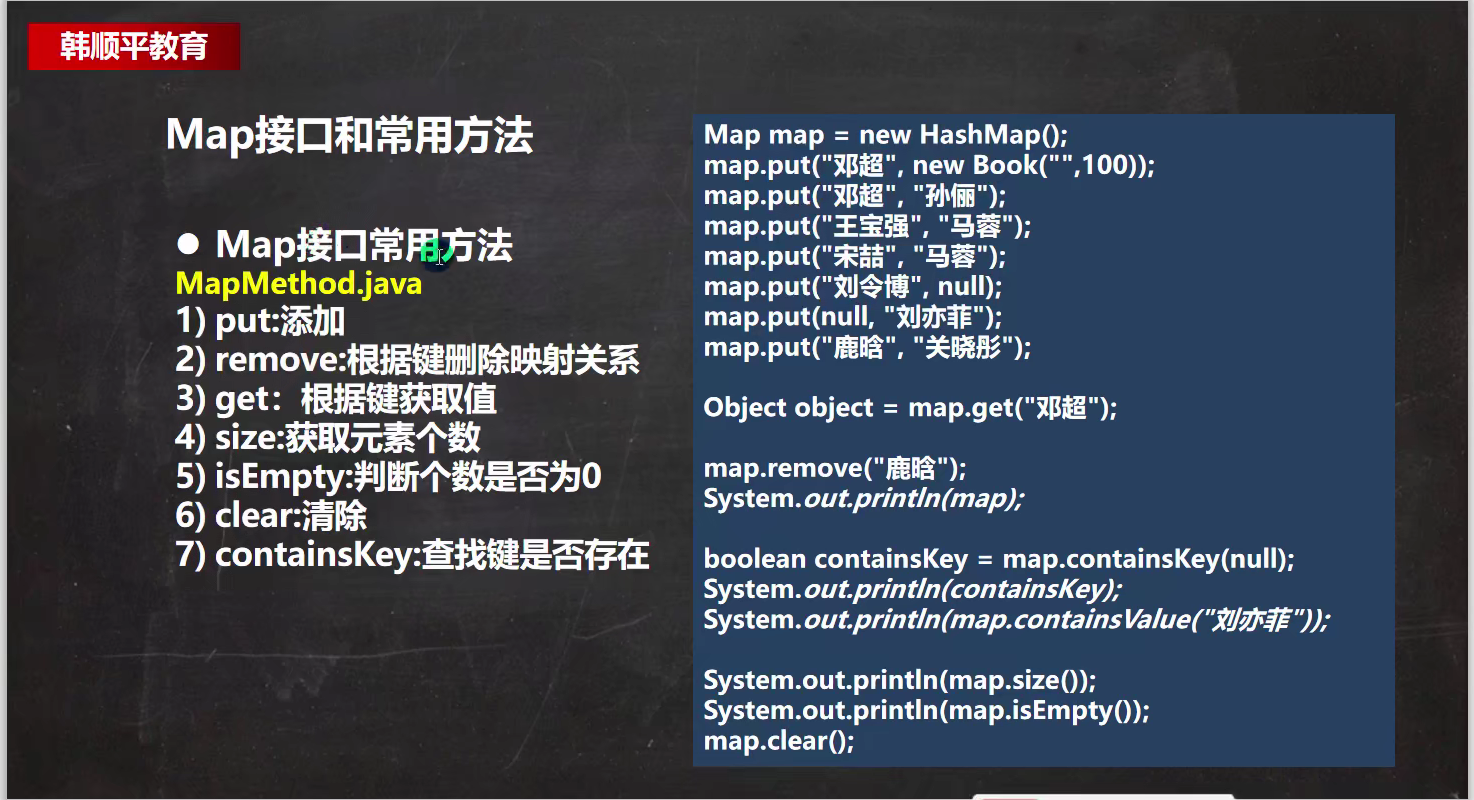
import java.util.HashMap;
import java.util.Map;
public class HashMap01 {
public static void main(String[] args) {
Map map=new HashMap();
map.put("cai","y");
map.put("chun","a");
map.put("mei","n");
map.put("cai","cai");
System.out.println(map);//{chun=a, mei=n, cai=cai}
map.remove("cai");
System.out.println(map);
System.out.println(map.get("mei"));//n
}
}
MAP的遍历
- 通过keySet集合遍历key 查找value
- 直接通过values集合 遍历输出value
- 通过entry集合里面的 Map.Entry (k,v)输出数据,注意Map.Entry和entry的转型
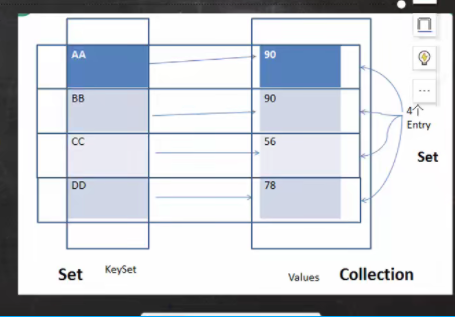
import arraylist.Iterator01;
import javax.swing.text.html.HTMLDocument;
import java.util.*;
public class HashMap02 {
public static void main(String[] args) {
Map map= new HashMap();
map.put("cai","蔡");
map.put("chun","春");
map.put("mei","梅");
//这是在keyset 集合里面通过遍历key 然后取出value
Set set=map.keySet();
for (Object key:set) {
System.out.println(key+ "-" + map.get(key));
}
//用迭代器一定要调用!!
Iterator iterator= set.iterator();
while (iterator.hasNext()){
Object key=iterator.next();
System.out.println(key +"-" +map.get(key));
}
//通过遍历 values集合 ,获取value的值
Collection values = map.values();
for (Object value:values) {
System.out.println(value);
}
//迭代器要引用
Iterator iterator1= values.iterator();
while (iterator1.hasNext()){
Object value=iterator1.next();
System.out.println(value);
}
//通过entry这个集合 来查找Map.Entry里面的(k,v)
Set entrySet = map.entrySet();
for (Object entry :entrySet) {
Map.Entry m=(Map.Entry)entry;//向下转型,entry(object)
System.out.println(m.getKey() +"-"+ m.getValue());
}
Iterator iterator2 = entrySet.iterator();
while (iterator2.hasNext()){
Map.Entry m=(Map.Entry)iterator2.next();//向下转型,iterator(objec)
System.out.println(m.getKey() +"-"+m.getValue());
}
}
}
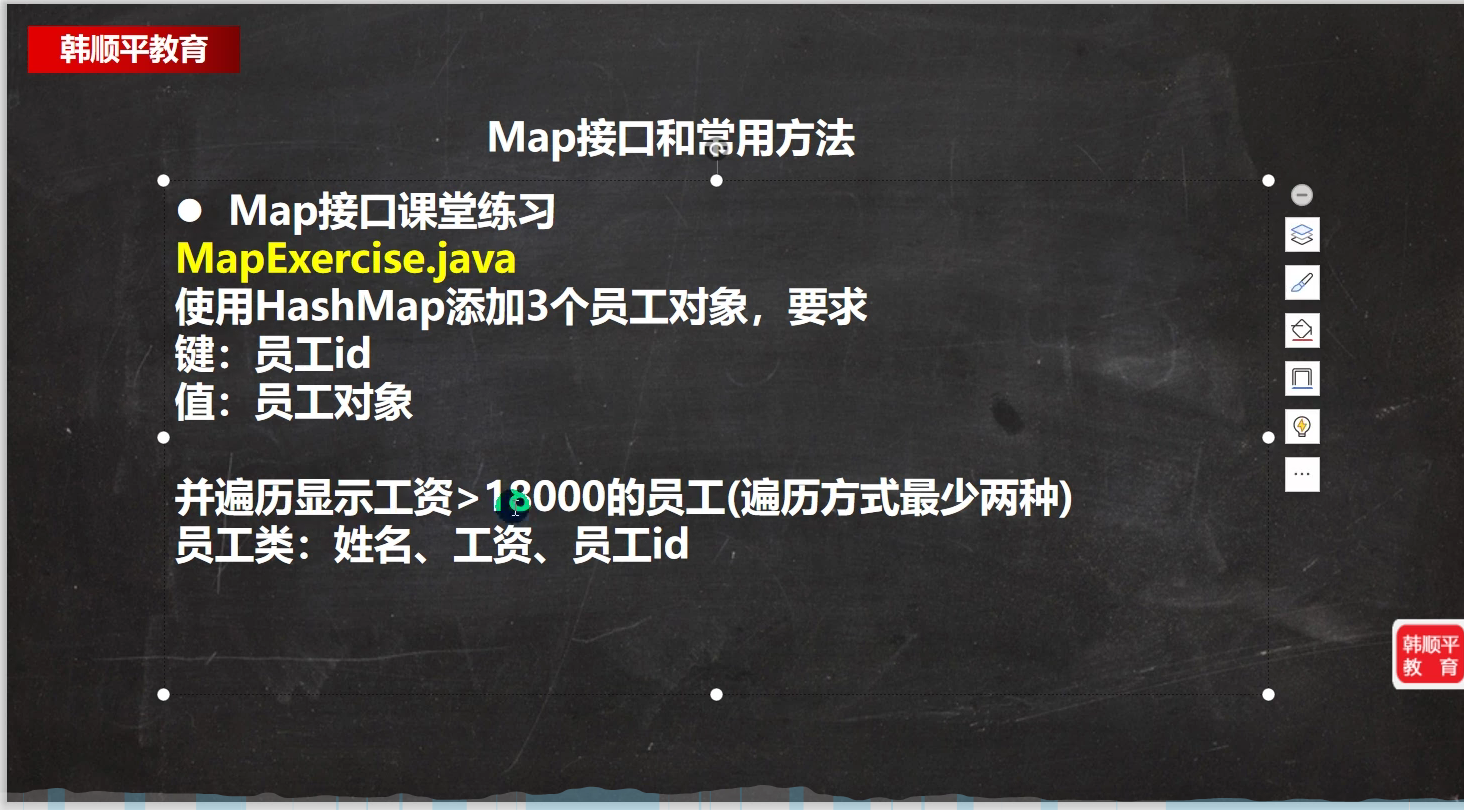
import com.sun.scenario.effect.impl.sw.sse.SSEBlend_SRC_OUTPeer;
import java.security.KeyStore;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
public class HashMapexer01 {
public static void main(String[] args) {
Map map = new HashMap();
map.put(1,new Person(1,"cai",12000));
map.put(2,new Person(2,"cai1",1200));
map.put(3,new Person(3,"cai2",12001));
Set keySet = map.keySet();
//用增强for循环
for (Object key :keySet) {
Person person=(Person) map.get(key);
if (person.getSal()>1880){
System.out.println(person);
}
}
//用entrySet迭代器
Set entrySet = map.entrySet();
Iterator iterator = entrySet.iterator();
while (iterator.hasNext()){
Map.Entry entry=(Map.Entry)iterator.next();
Person person=(Person) entry.getValue();
if (person.getSal()>10000){
System.out.println(person);
}
}
}
}
class Person{
private int id;
private String name;
private int sal;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSal() {
return sal;
}
public void setSal(int sal) {
this.sal = sal;
}
@Override
public String toString() {
return "Person{" +
"id=" + id +
", name='" + name + '\'' +
", sal=" + sal +
'}';
}
public Person(int id, String name, int sal) {
this.id = id;
this.name = name;
this.sal = sal;
}
}
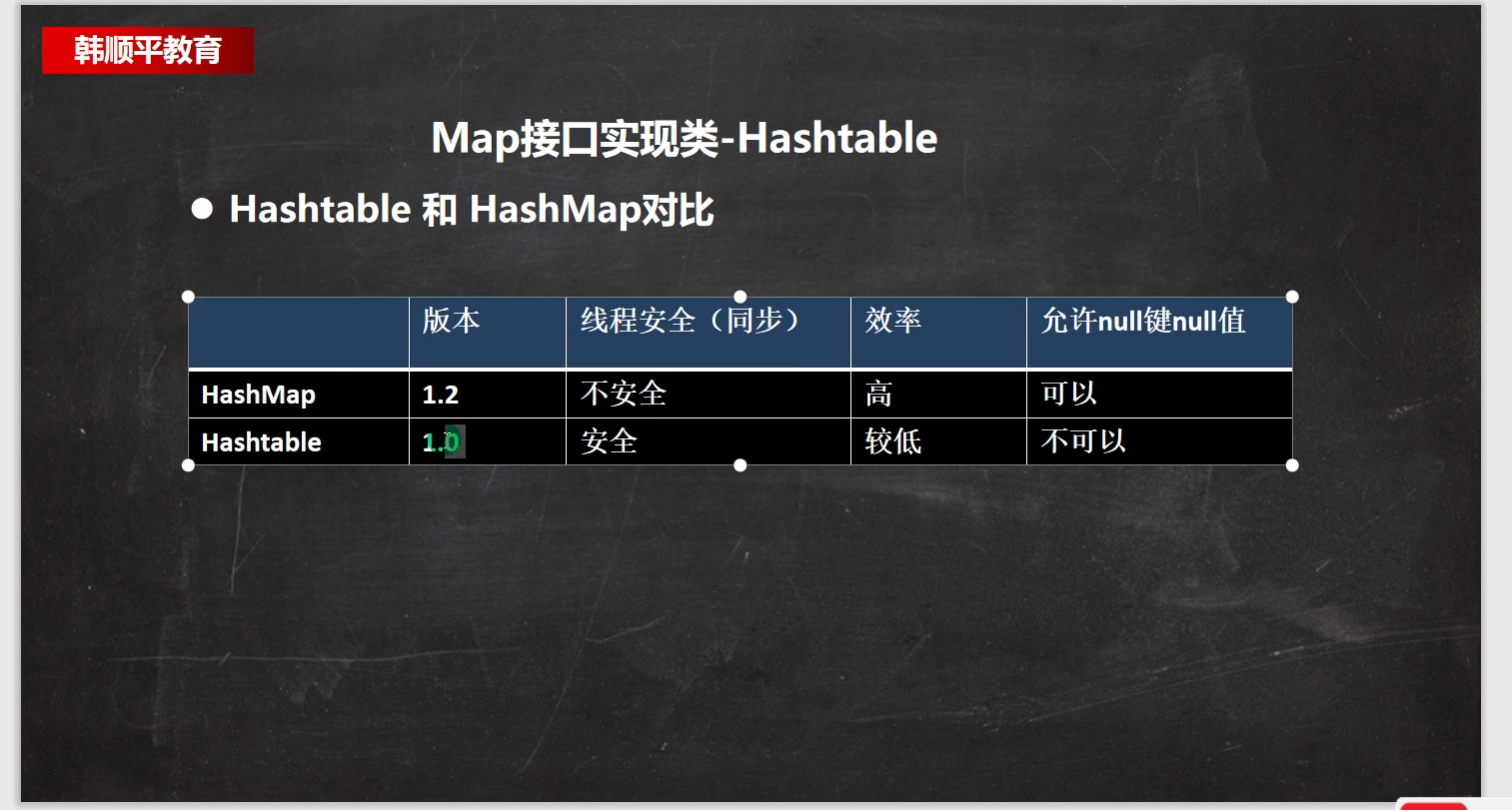
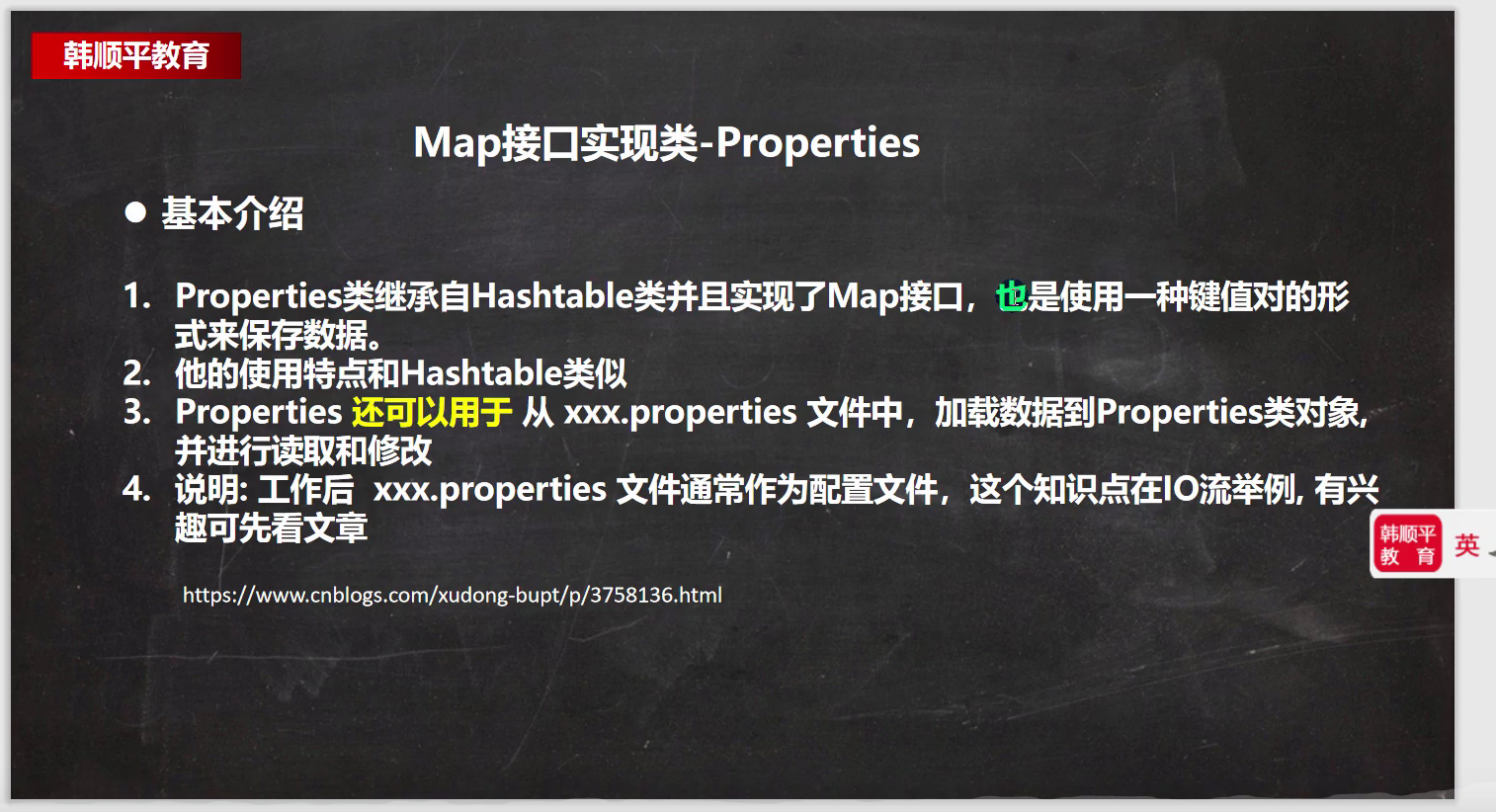