ArrayList与HashMap的嵌套使用
/*
ArrayList与HashMap的嵌套使用
*/
public static void main(String[] args) {
ArrayList<HashMap<String,String>> array = new ArrayList<>();
HashMap<String,String> hm1 = new HashMap<>();
hm1.put("孙策","大乔");
hm1.put("周瑜","小乔");
array.add(hm1);
HashMap<String,String> hm2 = new HashMap<>();
hm1.put("孙策1","大乔1");
hm1.put("周瑜1","小乔1");
array.add(hm2);
HashMap<String,String> hm3 = new HashMap<>();
hm1.put("孙策2","大乔2");
hm1.put("周瑜2","小乔2");
array.add(hm3);
for (HashMap<String,String> hm:array){
for (String key:hm.keySet()){
System.out.println(key+","+hm.get(key));
}
}
}
孙策2,大乔2 孙策1,大乔1 周瑜2,小乔2 孙策,大乔 周瑜1,小乔1 周瑜,小乔
Process finished with exit code 0
/*
ArrayList与HashMap的嵌套使用
*/
public static void main(String[] args) {
HashMap<String,ArrayList<String>> hm = new HashMap<>();
ArrayList<String> array1 = new ArrayList<>();
array1.add("诸葛");
array1.add("刘备");
hm.put("三国",array1);
ArrayList<String> array2 = new ArrayList<>();
array2.add("八戒");
array2.add("悟空");
hm.put("西游",array2);
ArrayList<String> array3 = new ArrayList<>();
array3.add("宋飞");
array3.add("武松");
hm.put("水浒",array3);
for (String s:hm.keySet()){
System.out.print(s+":");
for (String array:hm.get(s)){
System.out.print(array+"、");
}
System.out.println("\n");
}
}
水浒:宋飞、武松、
西游:八戒、悟空、
三国:诸葛、刘备、
Process finished with exit code 0
统计字符出现次数
hashmap 键值应为包装类
使用TreeMap可使结果有序
/*
统计字符串中各个字母出现的次数
*/
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入字符串:");
String line = sc.nextLine();
TreeMap<Character, Integer> hm = new TreeMap<>();
for (int i = 0; i < line.length(); i++) {
char key = line.charAt(i);
Integer value = hm.get(key);
//先判断键 是否存在
if (value == null){
hm.put(key,1);
}else{
value++;
hm.put(key,value);
}
}
StringBuffer sb = new StringBuffer();
Set<Character> keySet = hm.keySet();
for (Character key:keySet){
Integer value = hm.get(key);
sb.append(key).append("("+value+")");
}
String res = sb.toString();
System.out.println(res);
}
请输入字符串:bdacegf a(1)b(1)c(1)d(1)e(1)f(1)g(1)
Process finished with exit code 0
Collections 类
对集合操作的工具类
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(30);
list.add(10);
list.add(40);
list.add(50);
list.add(20);
//排序
Collections.sort(list);
System.out.println(list);
//[10, 20, 30, 40, 50]
//反转
Collections.reverse(list);
System.out.println(list);
//[50, 40, 30, 20, 10]
//随机排序
Collections.shuffle(list);
System.out.println(list);
//[20, 40, 50, 30, 10]
}
排序
public class Teacher {
private String name;
private int age;
public Teacher() {
}
public Teacher(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Teacher{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Teacher teacher = (Teacher) o;
if (age != teacher.age) return false;
return name != null ? name.equals(teacher.name) : teacher.name == null;
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age;
return result;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
创建匿名内部类排序
public static void main(String[] args) {
ArrayList<Teacher> ts = new ArrayList<>();
Teacher s1 = new Teacher("张三", 40);
Teacher s2 = new Teacher("李四", 30);
Teacher s3 = new Teacher("王五", 10);
Teacher s4 = new Teacher("李四", 20);
//Teacher s5 = new Teacher("m四", 20);
ts.add(s1);
ts.add(s2);
ts.add(s3);
ts.add(s4);
//set.add(s5);
//创建匿名内部类排序
Collections.sort(ts, new Comparator<Teacher>() {
@Override
public int compare(Teacher s1, Teacher s2) {
int num = s1.getAge() - s2.getAge(); //age升序
//如年龄相同,比较名字
int num2 = num == 0?s1.getName().compareTo(s2.getName()):num;
return num2;
}
});
for (Teacher t:ts){
System.out.println(t.getName()+","+t.getAge());
}
}
模拟斗地主
/*
模拟斗地主
*/
public static void main(String[] args) {
//定义花色
String[] colors = {"♥","♠","♦","♣"};
String[] numbers = {"2","3","4","5","6","7","8","9","10","J","Q","K","A"};
ArrayList<String> array = new ArrayList<>();
for (String color:colors){
for (String number:numbers){
array.add(color+number);
}
}
array.add("大王");
array.add("小王");
//洗牌
Collections.shuffle(array);
//System.out.println(array);
//发牌
ArrayList<String> player1 = new ArrayList<>();
ArrayList<String> player2 = new ArrayList<>();
ArrayList<String> player3 = new ArrayList<>();
ArrayList<String> less = new ArrayList<>();
for (int i = 0;i<array.size();i++){
String poker = array.get(i);
if (i>=array.size()-3){
less.add(poker);
}else if (i%3==0){
player1.add(poker);
}else if (i%3==1){
player2.add(poker);
}else if (i%3==2){
player3.add(poker);
}
}
showPoker("张三",player1);
showPoker("李四",player2);
showPoker("王五",player3);
showPoker("底牌",less);
}
public static void showPoker(String name,ArrayList<String> array){
System.out.print(name+":");
for (String s:array){
System.out.print(s+" ");
}
System.out.println();
}
张三:♦A ♠5 ♣7 ♣K ♣3 ♣9 ♦Q ♣2 小王 ♦K ♠3 ♦6 ♦8 ♥5 ♦10 ♠7 ♦4 李四:♠2 ♥A ♥8 ♥10 ♣A ♥6 ♣6 ♣J ♥Q ♠8 ♠J ♠9 ♥2 ♠Q ♥7 ♣8 ♠A 王五:♥3 ♣4 ♣10 ♥9 ♦5 ♠10 ♥4 ♦9 ♥K ♠4 ♠6 ♣Q ♥J ♦7 大王 ♠K ♦2 底牌:♣5 ♦J ♦3
对扑克排序
public class Demo {
/*
模拟斗地主
*/
public static void main(String[] args) {
//定义花色
String[] colors = {"♥", "♠", "♦", "♣"};
String[] numbers = {"2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"};
//对扑克按序 排列
//根据序列获取对应扑克
HashMap<Integer, String> hm = new HashMap<>();
ArrayList<Integer> array = new ArrayList<>();
int index = 1;
for (String number : numbers) {
for (String color : colors) {
array.add(index);
hm.put(index++, color + number);
}
}
array.add(index);
hm.put(index++, "♚"); //小王
array.add(index);
hm.put(index++, "♕"); //大王
System.out.println(array); //序列
/*
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17,
18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34,
35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51,
52, 53, 54]
*/
System.out.println(hm); //扑克
/*
{1=♥2, 2=♠2, 3=♦2, 4=♣2, 5=♥3, 6=♠3, 7=♦3, 8=♣3, 9=♥4, 10=♠4,
11=♦4, 12=♣4, 13=♥5, 14=♠5, 15=♦5, 16=♣5, 17=♥6, 18=♠6, 19=♦6, 20=♣6,
21=♥7, 22=♠7, 23=♦7, 24=♣7, 25=♥8, 26=♠8, 27=♦8, 28=♣8, 29=♥9, 30=♠9,
31=♦9, 32=♣9, 33=♥10, 34=♠10, 35=♦10, 36=♣10, 37=♥J, 38=♠J, 39=♦J, 40=♣J,
41=♥Q, 42=♠Q, 43=♦Q, 44=♣Q, 45=♥K, 46=♠K, 47=♦K, 48=♣K, 49=♥A, 50=♠A,
51=♦A, 52=♣A, 53=小王, 54=大王}
*/
//洗牌 -->打乱序号
Collections.shuffle(array);
//System.out.println(array);
//发牌 使用TreeSet集合存储序列,使之有序
TreeSet<Integer> player1 = new TreeSet<>();
TreeSet<Integer> player2 = new TreeSet<>();
TreeSet<Integer> player3 = new TreeSet<>();
TreeSet<Integer> less = new TreeSet<>();
//发牌 -->实际分发的是序号,最后根据序号,得到属于自己的牌
for (int i = 0;i<array.size();i++){
Integer poker = array.get(i);
if (i>=array.size()-3){
less.add(poker);
}else if (i%3==0){
player1.add(poker);
}else if (i%3==1){
player2.add(poker);
}else if (i%3==2){
player3.add(poker);
}
}
showPoker("张三",player1,hm);
showPoker("李四",player2,hm);
showPoker("王五",player3,hm);
showPoker("底牌",less,hm);
}
public static void showPoker(String name, TreeSet<Integer> array,HashMap<Integer,String> hm) {
System.out.print(name + ":");
for (Integer key : array) {
System.out.print(hm.get(key)+" ");
}
System.out.println(); //换行
}
}
张三:♣2 ♠3 ♦3 ♣3 ♥4 ♥5 ♠6 ♥7 ♥8 ♠8 ♣8 ♥9 ♣9 ♥10 ♠J ♣K ♦A 李四:♦2 ♥3 ♠4 ♦5 ♦7 ♠10 ♦10 ♣J ♥Q ♠Q ♦Q ♥K ♠K ♦K ♠A ♣A ♕ 王五:♥2 ♠2 ♦4 ♣4 ♠5 ♣5 ♥6 ♦6 ♣6 ♠7 ♣7 ♦8 ♠9 ♦9 ♣Q ♥A ♚ 底牌:♣10 ♥J ♦J
File类
构造方法
/*
File类
*/
public static void main(String[] args) {
File f1 = new File("D:\\Java\\test.txt");
System.out.println(f1); //D:\Java\test.txt
File f2 = new File("D:\\Java","test.txt");
System.out.println(f2); //D:\Java\test.txt
File f3 = new File("D:\\Java");
File f4 = new File(f3,"test.txt");
System.out.println(f4); //D:\Java\test.txt
}
创建文件或文件夹
public static void main(String[] args) throws IOException {
File f1 = new File("D:\\Java\\test.txt");
/*
抛出异常 throws IOException
如文件不存在,则创建文件,返回true
文件已存在则返回false
*/
//System.out.println(f1.createNewFile());
File f2 = new File("D:\\Java\\test");
/*
创建文件夹,不存在-->创建返回true
存在-->false
*/
//System.out.println(f2.mkdir());
File f3 = new File("D:\\Java\\test1\\test");
/*
创建多级文件夹,不存在-->创建返回true
存在-->false
*/
//System.out.println(f3.mkdirs());
}
常用方法
/*
File类
*/
public static void main(String[] args) throws IOException {
File f1 = new File("D:\\Java\\javaFound");
//是否为目录
System.out.println(f1.isDirectory());
//是否为文件
System.out.println(f1.isFile());
//是否存在
System.out.println(f1.exists());
//获取绝对路径
System.out.println(f1.getAbsolutePath());
//获取相对路径
System.out.println(f1.getPath());
//获取文件(夹)名
System.out.println(f1.getName());
File f = new File("D:\\Java");
//获取目录下文件列表
String[] str = f.list();
for (String s:str){
System.out.println(s);
}
System.out.println("-------------");
File[] files = f.listFiles();
for (File file:files){
if (file.isFile()){ //是否为文件
System.out.println(file); //路径
System.out.println(file.getName()); //文件名
}
}
}
true false true D:\Java\javaFound D:\Java\javaFound javaFound 09《Spring Boot企业级开发教材》 12《Java EE企业级应用开发Spring+Sspring MVC+MyBatis》 Java.md javaFound Java笔记.md SpringBoot SpringBoot.md SQL.md
毕业设计.md
D:\Java\Java.md Java.md D:\Java\Java笔记.md Java笔记.md D:\Java\SpringBoot.md SpringBoot.md D:\Java\SQL.md SQL.md D:\Java\毕业设计.md 毕业设计.md
Process finished with exit code 0
public static void main(String[] args) throws IOException {
File file = new File("test.txt");
//相对路径创建文件
//System.out.println(file.createNewFile());
//删除文件
System.out.println(file.delete());
File file1 = new File("src\\content");
//创建目录(文件夹)
System.out.println(file1.mkdir());
//删除目录
System.out.println(file1.delete());
//如目录下含有文件,则不能直接删除
}
递归
public static void main(String[] args) throws IOException {
System.out.println(rabbit(20)); //6765
System.out.println(jc(10)); //3628800
}
//求斐波那契数列第m项
public static int rabbit(int m){
//递归出口
if (m<3){
return 1;
}
return rabbit(m-1)+rabbit(m-2);
}
//求阶乘
public static int jc(int n){
if (n==1){
return 1;
}
return n*jc(n-1);
}
递归遍历文件
/*
递归
1.出口
2.规则
*/
public static void main(String[] args) throws IOException {
File f = new File("D:\\Java\\09_《Spring Boot企业级开发教材》");
fileContent(f);
}
//遍历目录下所有文件的绝对路径
public static void fileContent(File f) {
File[] files = f.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
fileContent(file);
} else {
System.out.println(file.getAbsolutePath());
}
}
}
}
D:\Java\09《Spring Boot企业级开发教材》\工具包\apache-maven-3.6.0-bin.zip D:\Java\09《Spring Boot企业级开发教材》\工具包\apache-tomcat-9.0.16.zip D:\Java\09《Spring Boot企业级开发教材》\工具包\jdk-8u201-windows-i586.exe D:\Java\09《Spring Boot企业级开发教材》\工具包\jdk-8u201-windows-x64.exe D:\Java\09《Spring Boot企业级开发教材》\工具包\otp_win64_21.2.exe D:\Java\09《Spring Boot企业级开发教材》\工具包\rabbitmq-server-3.7.9.exe D:\Java\09《Spring Boot企业级开发教材》\工具包\redis-desktop-manager-0.8.8.384.exe D:\Java\09《Spring Boot企业级开发教材》\工具包\Redis-x64-3.2.100.zip D:\Java\09《Spring Boot企业级开发教材》\源代码\Spring Boot教材资源.rar D:\Java\09《Spring Boot企业级开发教材》\源代码\Spring Boot配套源代码.zip D:\Java\09_《Spring Boot企业级开发教材》\课后题答案\SpringBoot教材课后习题答案.doc
Process finished with exit code 0
IO流概述
- 字节流
字符流
/*
字节流写入对象
*/
public static void main(String[] args) throws IOException {
//文件不存在则创建文件
FileOutputStream fos = new FileOutputStream("src\\taobao.txt");
//写入字节
fos.write(97); //a
fos.write(57); //9
byte[] bt = {57, 56, 55, 54, 53, 52, 51, 50, 49, 48};
fos.write(bt);
byte[] bt1 = "abcde".getBytes();
fos.write(bt1); //直接写入 abcde
//指定长度写入,从索引0开始,长度为3的bt1数组写入
fos.write(bt1,0,3);
//释放资源,关闭文件输出流
fos.close();
}
换行
换行
window: \r\n
linux: \n
mac: \r/*
字节流写入,异常处理,使程序更健壮
*/
public static void main(String[] args) {
//参数true,表示追加写入,默认false
FileOutputStream fos = null;
try {
fos = new FileOutputStream("src\\taobao.txt", true);
for (int i = 0; i < 10; i++) {
fos.write("hello".getBytes());
fos.write("\r\n".getBytes()); //换行
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
读取
当读到文件内容末位,返回值为-1
public static void main(String[] args) throws IOException {
//文件不存在则创建文件
FileInputStream fis = new FileInputStream("src\\taobao.txt");
//读取一个字节
int by;
//当读到文件内容末位,返回值为-1
while ((by = fis.read()) != -1) {
System.out.println((char) by);
}
//释放资源,关闭文件输出流
fis.close();
}
复制文件内容
public static void main(String[] args) throws IOException {
//将文件java.md的内容复制到taobao.txt
FileOutputStream fos = new FileOutputStream("src\\taobao.txt");//写入
FileInputStream fis = new FileInputStream("D:\\Java\\java.md"); //读取
//读取一个字节
int by;
//当读到文件内容末位,返回值为-1
while ((by = fis.read()) != -1) {
fos.write(by);
}
//释放资源,关闭文件输出流
fos.close();
fis.close();
}
读取文件—常用
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("src\\taobao.txt"); //读取
//读取N个字节
byte[] by = new byte[1024];
int len;
while ((len = fis.read(by)) != -1) {
System.out.println(new String(by, 0, len));
}
//释放资源,关闭文件输出流
fis.close();
}
复制图片
public static void main(String[] args) throws IOException {
FileOutputStream fos = new FileOutputStream("src\\aac.png");//写入
FileInputStream fis = new FileInputStream("D:\\Album\\1号线.png"); //读取
//读取N个字节
byte[] by = new byte[1024];
int len;
while ((len = fis.read(by)) != -1) {
//System.out.println(new String(by,0,len));
fos.write(by);
}
//释放资源,关闭文件输出流
fis.close();
fos.close();
}
字节缓冲流 读写数据
/*
字节缓冲流 读写数据
*/
public static void main(String[] args) throws IOException {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("src\\taobao.txt"));
bos.write("hello ".getBytes());
bos.write("world\n".getBytes());
bos.close();
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("src\\taobao.txt"));
byte[] by = new byte[1024];
int len;
while ((len = bis.read(by)) != -1) {
System.out.println(new String(by, 0, len));
}
bis.close();
}
复制视频
分别以4种方式复制
字节复制
- 字节数组复制
- 缓冲流字节复制
缓冲流字节数组复制 ——-最快!!!
public static void main(String[] args) throws IOException {
long start = System.currentTimeMillis();
CopyVideo2();
long end = System.currentTimeMillis();
System.out.println("耗时:" + (end - start) + "毫秒");
}
//耗时:156813毫秒
public static void CopyVideo() throws IOException {
//将文件java.md的内容复制到taobao.txt
FileOutputStream fos = new FileOutputStream("src\\code.mp4");//写入
FileInputStream fis = new FileInputStream("D:\\草稿\\202104182103.mp4"); //读取
//读取一个字节
int by;
//当读到文件内容末位,返回值为-1
while ((by = fis.read()) != -1) {
fos.write(by);
}
//释放资源,关闭文件输出流
fos.close();
fis.close();
}
//耗时:268毫秒
public static void CopyVideo1() throws IOException {
//将文件java.md的内容复制到taobao.txt
FileOutputStream fos = new FileOutputStream("src\\code.mp4");//写入
FileInputStream fis = new FileInputStream("D:\\草稿\\202104182103.mp4"); //读取
//读取一个字节
byte[] by = new byte[1024];
int len;
while ((len = fis.read(by)) != -1) {
fos.write(by);
}
//释放资源,关闭文件输出流
fos.close();
fis.close();
}
//耗时:1244毫秒
public static void CopyVideo2() throws IOException {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("src\\code.mp4"));
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("D:\\草稿\\202104182103.mp4"));
//读取一个字节
int by;
//当读到文件内容末位,返回值为-1
while ((by = bis.read()) != -1) {
bos.write(by);
}
bos.close();
bis.close();
}
//耗时:95毫秒
public static void CopyVideo3() throws IOException {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("src\\code.mp4"));
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("D:\\草稿\\202104182103.mp4"));
byte[] by = new byte[1024];
int len;
while ((len = bis.read(by)) != -1) {
bos.write(by);
}
bos.close();
bis.close();
}
字符流—编码-解码
```java
//String s = "abc"; //[97,98,99]
String s = "中国";
//相同,说明默认采用utf-8编码
//byte[] bys = s.getBytes(); //[-28, -72, -83, -27, -101, -67]
byte[] bys = s.getBytes("utf-8"); //[-28, -72, -83, -27, -101, -67]
//byte[] bys = s.getBytes("GBK"); //[-42, -48, -71, -6]
System.out.println(Arrays.toString(bys));
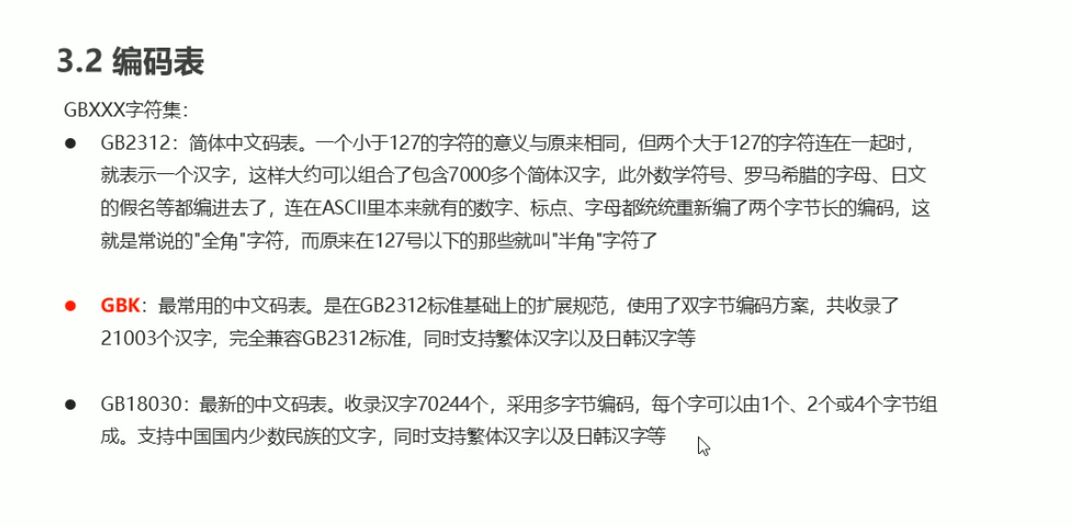<br />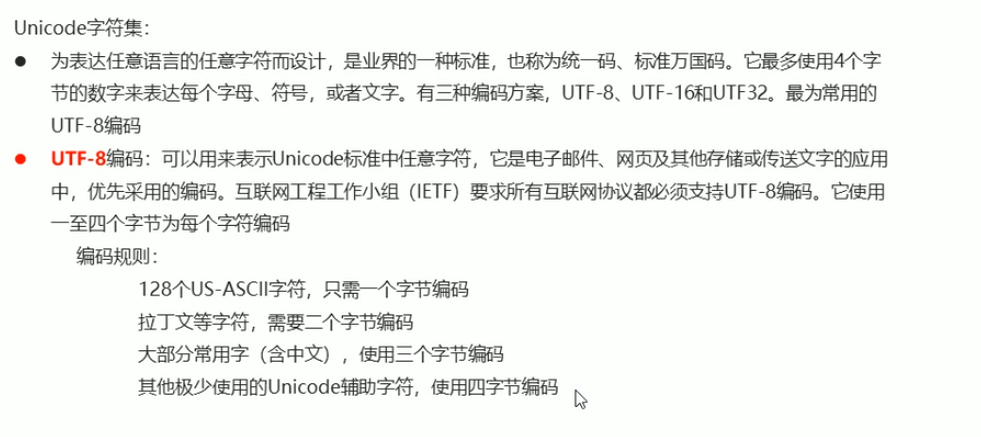
<a name="CptDh"></a>
## 读取文件
```java
public static void main(String[] args) throws IOException {
String s = "中国";
byte[] bys = s.getBytes("GBK"); //[-42, -48, -71, -6]
System.out.println(Arrays.toString(bys));
//用何编码,应以其解码,否则会乱码
String ss = new String(bys);
System.out.println(ss); //�й�
String ss1 = new String(bys,"GBK");
System.out.println(ss1); //中国
}
/**
* 字符流编码
* @param args
*/
public static void main(String[] args) throws IOException {
//字符流写入
OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("src\\owx.txt"), "utf-8");
osw.write("中国"); //默认utf-8编码
osw.write("你好世界", 2, 2); //只写入"世界"
osw.flush(); //刷新,从缓冲区存入
osw.close();
//字符流读取数据
InputStreamReader isr = new InputStreamReader(new FileInputStream("src\\Test01.java"), "utf-8");
//一次读一个字符
// int i;
// while ((i=isr.read())!=-1){
// System.out.print((char)i); //中国世界
// }
// 一次读一个数组
char[] chars = new char[1024];
int len;
while ((len = isr.read(chars)) != -1) {
System.out.println(new String(chars));
}
isr.close();
}
字符流复制—便捷类FileWriter-FileReader
public static void main(String[] args) throws IOException {
FileReader fd = new FileReader("src\\taobao.txt");
FileWriter fw = new FileWriter("src\\copy.txt");
char[] chars = new char[1024];
int len;
while ((len=fd.read(chars))!=-1){
fw.write(chars);
}
fd.close();
fw.close();
}
字符缓冲流
使用字节缓冲流复制文件
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("src\\taobao.txt"));
BufferedWriter bw = new BufferedWriter(new FileWriter("src\\copy.txt"));
char[] chars = new char[1024];
int len;
while ((len=br.read(chars))!=-1){
bw.write(chars);
}
br.close();
bw.close();
}
换行与一行读取
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("src\\taobao.txt"));
BufferedWriter bw = new BufferedWriter(new FileWriter("src\\copy.txt"));
bw.write("你好");
bw.newLine(); //换行
String line; //一行一行读取
while ((line = br.readLine())!=null){
System.out.println(line);
}
br.close();
bw.close();
}
最常用方式— 复制文件
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("src\\taobao.txt"));
BufferedWriter bw = new BufferedWriter(new FileWriter("src\\copy.txt"));
String line; //一行一行读取
while ((line = br.readLine())!=null){
bw.write(line);
bw.newLine();
bw.flush();
}
br.close();
bw.close();
}
}
点名
public class Demo {
/**
* 从文件中随机抽取一行
* @param args
* @throws IOException
*/
public static void main(String[] args) throws IOException {
ArrayList<String> arr = new ArrayList<>();
BufferedReader br = new BufferedReader(new FileReader("src\\taobao.txt"));
String line; //一行一行读取
while ((line = br.readLine())!=null){
arr.add(line);
}
br.close();
Random random = new Random();
int index = random.nextInt(arr.size());
String name = arr.get(index);
System.out.println(name);
}
}
集合对象写入
public static void main(String[] args) throws IOException {
ArrayList<Student> students = new ArrayList<>();
Student s1 = new Student("鞠婧祎",25,"武汉");
Student s2 = new Student("马保国",60,"重庆");
Student s3 = new Student("薛之谦",25,"上海");
students.add(s1);
students.add(s2);
students.add(s3);
BufferedWriter bw = new BufferedWriter(new FileWriter("src\\copy.txt"));
for (Student s:students){
bw.write(s.getName()+"--"+s.getAge()+"--"+s.getAddress());
bw.newLine();
}
bw.close();
}
复制单级文件夹—-有问题
public static void main(String[] args) throws IOException {
File file = new File("src\\duoc");
String srcname = file.getName();
File dest = new File("TestStudy",srcname);
if (!dest.exists()){
dest.mkdir();
}
File[] listFile = file.listFiles();
for (File f:listFile){
String srcFileName = f.getName();
File destFile = new File(dest,srcFileName);
copyFile(f,destFile);
}
}
public static void copyFile(File srcFile,File destFile) throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(srcFile));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destFile));
byte[] bys = new byte[1024];
int len;
while ((len=bis.read(bys))!=-1){
bos.write(bys,0,len);
}
bos.close();
bis.close();
}
复制多级文件夹—递归
打印流复制文件
对象序列化流
对象反序列化流
关键字 修饰符transient
Properties集合类
猜数字游戏,限制游戏次数
public class Demo {
/**
* 从文件中随机抽取一行
* @param args
* @throws IOException
*/
public static void main(String[] args) throws IOException {
Properties prop = new Properties();
FileReader fr = new FileReader("src\\owx.txt");
//文件写入 count = 0
prop.load(fr);
fr.close();
String count = prop.getProperty("count");
int number = Integer.parseInt(count);
if (number>2){
System.out.println("请充值后继续");
}else{
Demo.GuessNumber();
number++;
prop.setProperty("count",String.valueOf(number));
FileWriter fw = new FileWriter("src\\owx.txt");
prop.store(fw,null);
fw.close();
}
}
public static void GuessNumber(){
Random r = new Random();
int number = r.nextInt(100)+1;
while (true){
Scanner sc = new Scanner(System.in);
System.out.print("请输入猜的数值:");
int guessNum = sc.nextInt();
if (guessNum>number){
System.out.println("大了哦");
}else if (guessNum<number){
System.out.println("小了点");
}else{
System.out.println("猜对了");
break;
}
}
}
}
进程与线程
多线程的实现方式
方式一:继承Thread类
- 继承Tread类
- 重写run()方法
- 创建类对象
启动线程
class Demo{
public static void main(String[] args) {
MyThread m1 = new MyThread();
MyThread m2 = new MyThread();
//普通方式
// m1.run();
// m2.run();
//以start启动线程,再由JVM调用run方法
//不会等到m1的run方法跑完就会执行m2的run方法
m1.start();
m2.start();
}
}
public class MyThread extends Thread {
@Override
public void run() {
int i = 0;
while (i<100){
System.out.println(i++);
}
}
}
设定与获取线程名称
不设置获取默认值
线程名称默认值
第一次调用: Thread-0
第二次调用: Thread-1public static void main(String[] args) {
MyThread m1 = new MyThread();
MyThread m2 = new MyThread();
//普通方式
// m1.run();
// m2.run();
//以start启动线程,再由JVM调用run方法
//不会等到m1的run方法跑完就会执行m2的run方法
m1.setName("飞机");
System.out.println(m1.getName());
m1.start();
m2.setName("大炮");
System.out.println(m2.getName());
m2.start();
}
或者提供一个带参构造方法
public static void main(String[] args) {
MyThread m1 = new MyThread("飞机");
MyThread m2 = new MyThread("大炮");
//普通方式
// m1.run();
// m2.run();
//以start启动线程,再由JVM调用run方法
//不会等到m1的run方法跑完就会执行m2的run方法
System.out.println(m1.getName());
m1.start();
System.out.println(m2.getName());
m2.start();
}
class Demo{
public static void main(String[] args) {
//返回当前正在执行的线程名称
System.out.println(Thread.currentThread().getName());
//main
}
}
线程调度模型
- 分时调度模型
- 抢占式调度模型
java使用的是抢占式调度模型
随机性
优先级—存在范围 [1,10]
默认值— 5
m1.setPriority(10);
m3.setPriority(1);
线程控制
@Override
public void run() {
int i = 0;
while (i<100){
System.out.println(getName()+"--"+i++);
try {
Thread.sleep(1000); //每隔1秒执行每个线程一次
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
MyThread m1 = new MyThread("飞机");
MyThread m2 = new MyThread("高铁");
MyThread m3 = new MyThread("骑车");
// m1.setPriority(10);
// m3.setPriority(1);
m1.start();
try {
m1.join(); //只有m1线程停止后,才执行其他线程
} catch (InterruptedException e) {
e.printStackTrace();
}
m2.start();
m3.start();
}
守护线程 —不是立即结束!!
class Demo{
public static void main(String[] args) {
MyThread m1 = new MyThread("飞机");
MyThread m2 = new MyThread("高铁");
MyThread m3 = new MyThread("骑车");
// m1.setPriority(10);
// m3.setPriority(1);
m1.start();
m2.start();
m2.setDaemon(true); //设定为守护线程,主线程结束后,守护线程应也马上结束
m3.start();
m3.setDaemon(true);
Thread.currentThread().setName("主线程");
int i = 0;
while (i<10){
System.out.println(Thread.currentThread().getName()+"--"+i++);
}
}
}
线程的生命周期
方式二:实现Runnable接口
public class MyThread implements Runnable {
public MyThread() {
}
@Override
public void run() {
int i = 0;
while (i<100){
System.out.println(Thread.currentThread().getName()+"--"+i++);
// try {
// Thread.sleep(1000); //每隔1秒执行每个线程一次
// } catch (InterruptedException e) {
// e.printStackTrace();
// }
}
}
}
class Demo{
public static void main(String[] args) {
MyThread m = new MyThread();
Thread t1 = new Thread(m,"飞机");
Thread t2 = new Thread(m,"火车");
t1.start();
t2.start();
}
}
卖票案例
public class MyThread implements Runnable {
private int tickets = 100; //总票数
private Object obj = new Object();
public MyThread() {
}
@Override
public void run() {
while (true){
//解决线程同步安全问题,将不会出现两个窗口同时出售同一张票的情况
synchronized (obj){
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
if(tickets > 0){
System.out.println(Thread.currentThread().getName()+"正在出售第"+tickets--+"张票");
}
}
}
}
}
public static void main(String[] args) {
MyThread m = new MyThread();
Thread t1 = new Thread(m,"VIP窗口");
Thread t2 = new Thread(m,"普通窗口");
Thread t3 = new Thread(m,"附加窗口");
t1.setPriority(8);
t3.setPriority(2);
t1.start();
t2.start();
t3.start();
}
同步方法锁定this
以修饰符 synchronized 修饰方法