join()
console.log(arr.join(""))
console.log(arr.join("|"));
console.log(arr.join(" "));
console.log(arr.join("*"));
console.log(typeof arr.join("")) //string
var directs = [
{name:"吴京",id:1001},
{name:"章子怡",id:1002},
{name:"徐峥",id:1003}
]
/* str =吴京/章子怡/徐峥 */
/* 1. var arr = ["吴京","章子怡","徐峥"] */
var arr = [ ];
for(var i=0;i<directs.length;i++){
console.log(directs[i].name)
arr.push(directs[i].name)
}
console.log(arr)
/* 2. str =吴京/章子怡/徐峥*/
var str = arr.join("/");
console.log(str)
/* 3. */
var app = document.getElementById("app");
app.innerHTML = str;
sort()
var arr = [12,3,5,11,1];
console.log(arr.sort())
/* 得到从小到大的数据结构 */
/*
arr.sort(function(a,b){
return a-b;
})
*/
var newArr = arr.sort(function(a,b){
return a-b;
})
var c = [12,14,45,2]
/*
降序
arr.sort(function(a,b){
return b-a;
})
*/
var test = c.sort(function(a,b){
return b-a;
})
console.log(newArr)
console.log(test)
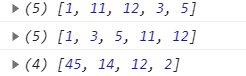
<body>
<select id="mySelect">
<option value="price">低价优先</option>
<option value="distance">距离优先</option>
</select>
<script>
var arr = [{
name:"肯德基",price:100,distance:100
},
{
name:"必胜客",price:200,distance:1500
},
{
name:"华莱士",price:50,distance:1000
}
]
var mySelect = document.getElementById("mySelect");
// console.log(mySelect)
mySelect.onchange = function(){
console.log(this.value);
var value = this.value;
arr.sort(function(a,b){
return a[value]-b[value];
})
/* 对象的某个属性是变量,不能使用.。只能使用[] */
console.log(arr);
}
</script>
</body>
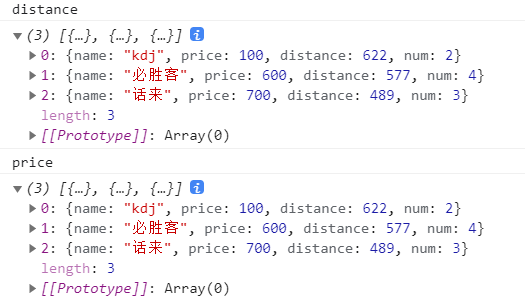
reduce()
var arr = [1,2,3];
/*
arr.reduce((a,b)=>a+b)
*/
var sum = arr.reduce((a,b)=>{
return a+b;
})
console.log(sum)
//输出6
reverse()
//将数组翻转,可以改变数组结构
for in
/* for in */
var arr = ["html","css","js"];
for(var key in arr){
console.log(key) //key获取的是数组的下标
// console.log(arr[key])
}
var obj = {
name:"lisi",
age:18
}
for(var k in obj){
console.log(obj[k])
}
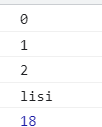
for of
var arr = [3,4,5];
for(var value of arr){
// console.log(value)
/* 获取下标怎么获 */
/* indexOf */
console.log(arr.indexOf(value))
}
for each
var names = [{name:"李四"},{name:"王五"}];
names.forEach(item=>{
item.like = true;
})
console.log(names)
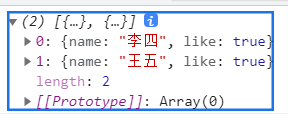
map()
var arr = [
{name:"html",value:"12px"},
{name:"css",value:"13px"},
{name:"js",value:"14px"}
]
/*
[
{name:"html",value:24},
{name:"css",value:26},
{name:"js",value:28}
]
*/
arr.map(item=>{
var value = parseInt(item.value)*2;
item.value = value;
})
console.log(arr)
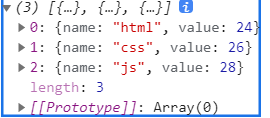
every()
var arr = [2,3,4];
var res = arr.every(item=>{
return item>2;
})
console.log(res)//返回false
some()
var arr = [3,4,5,6];
/* 只有数组中一项满足某个条件,结果就是true */
var res = arr.some(item=>{
return item>3;
})
console.log(res)//
filter()
/* 只要是达到某个条件,就会返回一个新的数组 */
var arr = [3,4,5,6];
var res = arr.filter(item=>{
return item>4;
})
console.log(res)
var students = [
{name:"李四",age:"18岁"},
{name:"李二",age:"11岁"},
{name:"李五",age:"19岁"},
{name:"王四",age:"22.5岁"},
]
/* 大于11岁,返回一个新的数组 */
var result = students.filter(item=>{
return parseFloat(item.age)>11;
})
console.log(result)
find()
/* find
返回数组中满足条件的某个值
*/
var arr = [2,3,4,3];
var res = arr.find(item=>{
return item == 3;
})
console.log(res)
findIndex()
/* 返回数组中满足某个条件的值的下标 */
var arr =[2,3,4];
var index = arr.findIndex(item=>{
return item==4;
})
console.log(index)