异常体系结构
java.lang.Throwable
- java.lang.Error:一般不编写针对性的代码进行处理。
java.lang.Exception:可以进行异常的处理
- 编译时异常(checked)
- IOException
- FileNotFoundException
- ClassNotFoundException
- IOException
运行时异常(unchecked,RuntimeException)
- NullPointerException
- ArrayIndexOutOfBoundsException
- ClassCastException
- NumberFormatException
- InputMismatchException
- ArithmeticException
Error
Java虚拟机无法解决的严重问题。如:JVM系统内部错误、资源耗尽等严重情况。(StackOverflowError和OOM)
一般不编写针对性的代码进行处理。 ```java public class ErrorTest { public static void main(String[] args) { //1.栈溢出:java.lang.StackOverflowError // main(args); //2.堆溢出:java.lang.OutOfMemoryError Integer[] arr = new Integer[102410241024];
} }
<a name="TdW44"></a>
## Exception
<a name="sQ9Fw"></a>
### 编译时异常(红色)
```java
//******************以下是编译时异常***************************
@Test
public void test7(){
File file = new File("hello.txt");
FileInputStream fis = new FileInputStream(file);
int data = fis.read();
while(data != -1){
System.out.print((char)data);
data = fis.read();
}
fis.close();
}
- 编译时异常(checked)
运行时异常(蓝色)
//******************以下是运行时异常***************************
//ArithmeticException
@Test
public void test6(){
int a = 10;
int b = 0;
System.out.println(a / b);
}
//InputMismatchException
@Test
public void test5(){
Scanner scanner = new Scanner(System.in);
int score = scanner.nextInt();
System.out.println(score);
scanner.close();
}
//NumberFormatException
@Test
public void test4(){
String str = "123";
str = "abc";
int num = Integer.parseInt(str);
}
//ClassCastException
@Test
public void test3(){
Object obj = new Date();
String str = (String)obj;
}
//IndexOutOfBoundsException
@Test
public void test2(){
//ArrayIndexOutOfBoundsException
// int[] arr = new int[10];
// System.out.println(arr[10]);
//StringIndexOutOfBoundsException
String str = "abc";
System.out.println(str.charAt(3));
}
//NullPointerException
@Test
public void test1(){
// int[] arr = null;
// System.out.println(arr[3]);
String str = "abc";
str = null;
System.out.println(str.charAt(0));
}
🌟异常处理机制
异常的处理:抓抛模型
- 过程一:”抛”:程序在正常执行的过程中,一旦出现异常,就会在异常代码处生成一个对应异常类的对象,并将此对象抛出,一旦抛出对象以后,其后的代码就不再执行。
- 关于异常对象的产生:① 系统自动生成的异常对象 ② 手动的生成一个异常对象,并抛出(throw)。
- 过程二:”抓”:可以理解为异常的处理方式:① try-catch-finally ② throws
try-catch-finally
```java public class ExceptionTest1 {try{ //可能出现异常的代码 }catch(异常类型1 变量名1){ //处理异常的方式1 }catch(异常类型2 变量名2){ //处理异常的方式2 }catch(异常类型3 变量名3){ //处理异常的方式3 } .... finally{ //一定会执行的代码 }
@Test
public void test2(){
try{
File file = new File("hello.txt");
FileInputStream fis = new FileInputStream(file);
int data = fis.read();
while(data != -1){
System.out.print((char)data);
data = fis.read();
}
fis.close();
}catch(FileNotFoundException e){
e.printStackTrace();
}catch(IOException e){
e.printStackTrace();
}
}
@Test
public void test1(){
String str = "123";
str = "abc";
int num = 0;
try{
num = Integer.parseInt(str);
System.out.println("hello-----1");
}catch(NumberFormatException e){
// System.out.println(“出现数值转换异常了,不要着急….”); //String getMessage(): // System.out.println(e.getMessage()); //printStackTrace(): e.printStackTrace(); }catch(NullPointerException e){ System.out.println(“出现空指针异常了,不要着急….”); }catch(Exception e){ System.out.println(“出现异常了,不要着急….”);
}
System.out.println(num);
System.out.println("hello-----2");
}
}
说明:
1. finally是可选的。
1. 使用try将可能出现异常代码包装起来,在执行过程中,一旦出现异常,就会生成一个对应异常类的对象,根据此对象的类型,去catch中进行匹配。
1. 一旦try中的异常对象匹配到某一个catch时,就进入catch中进行异常的处理。一旦处理完成,就跳出当前的try-catch结构(在没有写finally的情况)。继续执行其后的代码。
1. catch中的异常类型如果没有子父类关系,则谁声明在上,谁声明在下无所谓。
catch中的异常类型如果满足子父类关系,则要求子类一定声明在父类的上面,否则报错。
5. 常用的异常对象处理的方式: ① String getMessage() ② printStackTrace()
5. 在try结构中声明的变量,再出了try结构以后,就不能再被调用。
5. try-catch-finally结构可以嵌套。
体会1:使用try-catch-finally处理编译时异常,是得程序在编译时就不再报错,但是运行时仍可能报错。相当于我们使用try-catch-finally将一个编译时可能出现的异常,延迟到运行时出现。 <br />体会2:开发中,由于运行时异常比较常见,所以我们通常就不针对运行时异常编写try-catch-finally了。针对于编译时异常,我们说一定要考虑异常的处理。
<a name="ZLWRU"></a>
### finally的使用
1. finally是可选的。
1. finally中声明的**是一定会被执行的代码**。即使catch中又出现异常了,try中有return语句,catch中有return语句等情况。
1. 像数据库连接、输入输出流、网络编程Socket等资源,JVM是不能自动的回收的,我们需要自己手动的进行资源的释放。此时的资源释放,就需要声明在finally中。
```java
public class FinallyTest {
@Test
public void test2() {
FileInputStream fis = null;
try {
File file = new File("hello1.txt");
fis = new FileInputStream(file);
int data = fis.read();
while (data != -1) {
System.out.print((char) data);
data = fis.read();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void testMethod() {
int num = method();
System.out.println(num);
}
public int method() {
try {
int[] arr = new int[10];
System.out.println(arr[10]);
return 1;
} catch (ArrayIndexOutOfBoundsException e) {
e.printStackTrace();
return 2;
} finally {
System.out.println("我一定会被执行");
return 3;
}
}
@Test
public void test1() {
try {
int a = 10;
int b = 0;
System.out.println(a / b);
} catch (ArithmeticException e) {
e.printStackTrace();
// int[] arr = new int[10];
// System.out.println(arr[10]);
} catch (Exception e) {
e.printStackTrace();
}
// System.out.println("我好帅啊!!!~~");
finally {
System.out.println("我好帅啊~~");
}
}
}
throws
- “throws + 异常类型”写在方法的声明处。指明此方法执行时,可能会抛出的异常类型。
一旦当方法体执行时,出现异常,仍会在异常代码处生成一个异常类的对象,此对象满足throws后异常类型时,就会被抛出。异常代码后续的代码,就不再执行! - 体会:try-catch-finally 真正的将异常给处理掉了。
throws的方式只是将异常抛给了方法的调用者,并没有真正将异常处理掉。 开发中如何选择使用try-catch-finally 还是使用throws?
- 如果父类中被重写的方法没有throws方式处理异常,则子类重写的方法也不能使用throws,意味着如果子类重写的方法中有异常,必须使用try-catch-finally方式处理。
执行的方法a中,先后又调用了另外的几个方法,这几个方法是递进关系执行的。我们建议这几个方法使用throws的方式进行处理。而执行的方法a可以考虑使用try-catch-finally方式进行处理。 ```java public class ExceptionTest2 {
public static void main(String[] args) { try {
method2();
} catch (IOException e) {
e.printStackTrace();
}
// method3();
}
public static void method3() {
try {
method2();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void method2() throws IOException {
method1();
}
public static void method1() throws FileNotFoundException, IOException {
File file = new File("hello1.txt");
FileInputStream fis = new FileInputStream(file);
int data = fis.read();
while (data != -1) {
System.out.print((char) data);
data = fis.read();
}
fis.close();
System.out.println("hahaha!");
}
}
<a name="iIavy"></a>
## 手动抛出异常
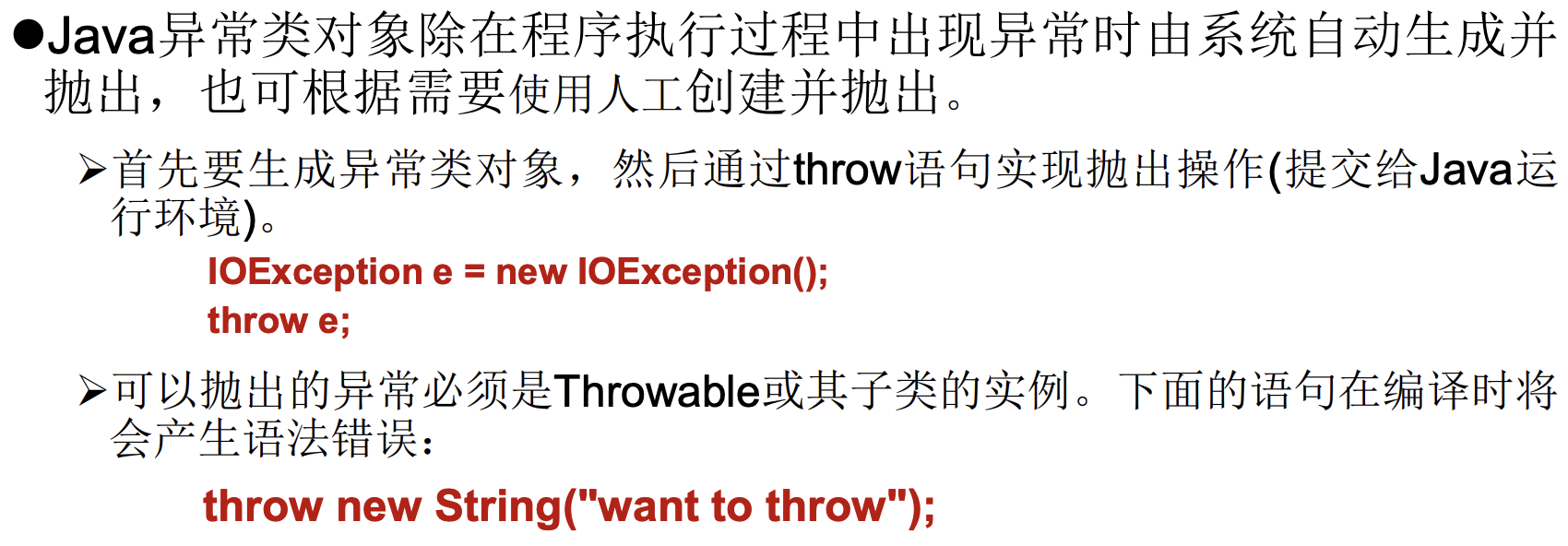
```java
public class StudentTest {
public static void main(String[] args) {
try {
Student s = new Student();
s.regist(-1001);
System.out.println(s);
} catch (Exception e) {
// e.printStackTrace();
System.out.println(e.getMessage());
}
}
}
class Student{
private int id;
public void regist(int id) throws Exception {
if(id > 0){
this.id = id;
}else{
// System.out.println("您输入的数据非法!");
//手动抛出异常对象
// throw new RuntimeException("您输入的数据非法!");
// throw new Exception("您输入的数据非法!");
throw new MyException("不能输入负数");
//错误的
// throw new String("不能输入负数");
}
}
@Override
public String toString() {
return "Student [id=" + id + "]";
}
}
用户自定义异常类
- 继承于现有的异常结构:RuntimeException 、Exception
- 提供全局常量:serialVersionUID
提供重载的构造器
public class MyException extends Exception{ static final long serialVersionUID = -7034897193246939L; public MyException(){ } public MyException(String msg){ super(msg); } }