1- 底部弹窗组件(showModalBottomSheet)
1. 使用 showModalBottom,带阴影的底部弹窗
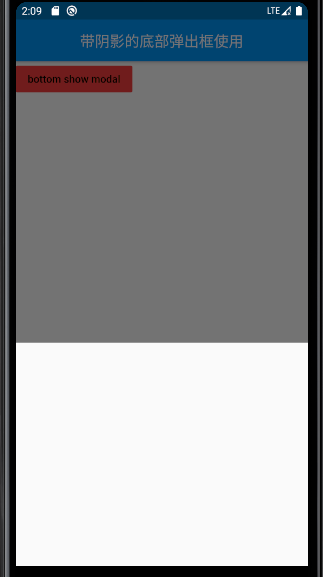
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: () {
// 带阴影的底部弹窗框
showModalBottomSheet(
context: context,
builder: (BuildContext context) {
return Container(
height: 300,
);
},
);
},
child: Text("bottom show modal"),
color: Colors.red,
),
);
}
}
2. 添加取消,确定按钮,点按钮可关闭弹窗
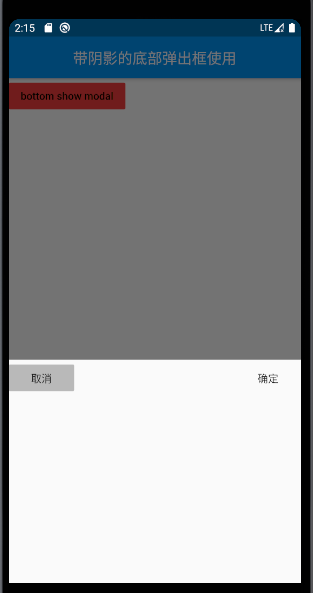
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: () {
showModalBottomSheet(
context: context,
builder: (BuildContext context) {
return Container(
height: 300,
child: Column(
children: [
// 添加取消,确定按钮
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
FlatButton(
onPressed: () {
// 点击关闭
Navigator.of(context).pop();
},
child: Text("取消")),
FlatButton(
onPressed: () {
// 点击关闭
Navigator.of(context).pop();
},
child: Text("确定")),
],
)
],
),
);
},
);
},
child: Text("bottom show modal"),
color: Colors.red,
),
);
}
}
2- 底部弹窗组件(showBottomSheet)
1. showBottomSheet 没有黑色蒙层的弹窗
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
// 先定义key
final _key = GlobalKey<ScaffoldState>();
// 打开底部弹窗
_openBottomSheet() {
_key.currentState.showBottomSheet(
(context) => Container(
height: 300,
color: Colors.grey,
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _key,
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: _openBottomSheet,
child: Text("bottom show modal"),
color: Colors.green,
),
);
}
}
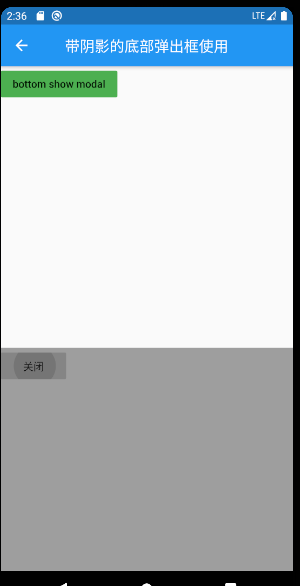
2.使用 Navigator.of(context).pop() 关闭弹窗
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
// 定义key
final _key = GlobalKey<ScaffoldState>();
// 打开底部弹窗
_openBottomSheet() {
_key.currentState.showBottomSheet(
(context) => Container(
height: 300,
color: Colors.grey,
child: Column(
children: [
Row(
children: [
FlatButton(
// 关闭底部弹窗
onPressed: () {
Navigator.of(context).pop();
},
child: Text("关闭"),
)
],
)
],
),
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _key,
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: _openBottomSheet,
child: Text("bottom show modal"),
color: Colors.green,
),
);
}
}
3. ios风格的底部滚动列表
1. 使用 CupertinoPicker 组件,实现滚动
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
// 第一行的左右按钮
_oneRowButton() {
return Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("取消"),
),
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("确定"),
)
],
);
}
// 打开底部弹窗
_openBottomSheet() {
showModalBottomSheet(
context: context,
builder: (BuildContext context) {
return Container(
height: 320,
child: Column(
children: [
_oneRowButton(),
// 底部的ios风格的滚动列表
_iosWheelWidget(),
],
),
);
},
);
}
// 滚动选择列表
_iosWheelWidget() {
return Expanded(
child: CupertinoPicker(
itemExtent: 35,
onSelectedItemChanged: (int index) {},
children: [
Text("1年工作经验"),
Text("2年工作经验"),
Text("3年工作经验"),
Text("4年工作经验"),
Text("5年工作经验"),
],
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: _openBottomSheet,
child: Text("ios底部滚动列表"),
color: Colors.green,
),
);
}
}
2. CupertinoPicker 组件的常用参数配置说明
可选,必选参数名 |
参数传值说明 |
itemExtent |
每个子项高度 |
children |
子widget组。List |
onSelectedItemChanged |
滚动选择的回调,每次滚动,都会触发此回调,会将选中的widget的 索引 返回 |
useMagnifier |
是否开启选中项的字体放大功能,默认false |
magnification |
选中的元素,放大的倍数,在useMagnifier:true 的情况下有效 |
backgroundColor |
给CupertinoPicker容器添加 背景颜色 |
offAxisFraction |
控制选中的widget元素的左右偏移量,默认是0.0。,正数向右偏移,负数向左偏移 |
scrollController |
控制器,通过 FixedExtentScrollController()创建,可以实现默认选中的哪一个 |
3. 给 CupertinoPicker 设置默认选中的值
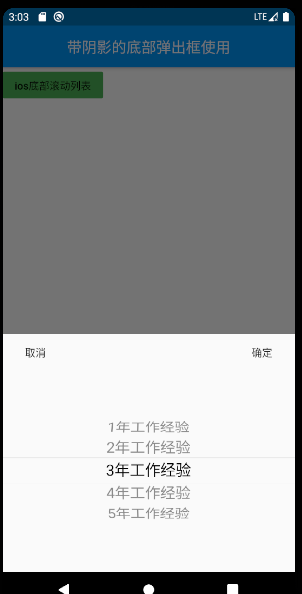
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class CityPage extends StatefulWidget {
@override
_CityPageState createState() => _CityPageState();
}
class _CityPageState extends State<CityPage> {
// 1. 创建 控制器
FixedExtentScrollController _controller;
@override
initState() {
super.initState();
// 控制器实例化, 传入默认选中的索引
_controller = FixedExtentScrollController(initialItem: 2);
}
// 第一行的左右按钮
_oneRowButton() {
return Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("取消"),
),
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("确定"),
)
],
);
}
// 打开底部弹窗
_openBottomSheet() {
showModalBottomSheet(
context: context,
builder: (BuildContext context) {
return Container(
height: 320,
child: Column(
children: [
_oneRowButton(),
// 底部的ios风格的滚动列表
_iosWheelWidget(),
],
),
);
},
);
}
// 滚动选择列表
_iosWheelWidget() {
return Expanded(
child: CupertinoPicker(
itemExtent: 35,
scrollController: _controller, // 绑定控制器
onSelectedItemChanged: (int index) {},
children: [
Text("1年工作经验"),
Text("2年工作经验"),
Text("3年工作经验"),
Text("4年工作经验"),
Text("5年工作经验"),
],
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("带阴影的底部弹出框使用"),
centerTitle: true,
),
body: FlatButton(
onPressed: _openBottomSheet,
child: Text("ios底部滚动列表"),
color: Colors.green,
),
);
}
}