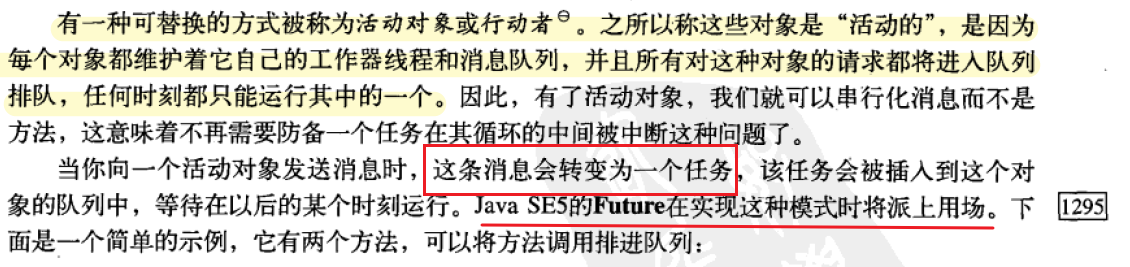
import java.util.concurrent.*;
import java.util.*;
public class ActiveObjectDemo1 {
private ExecutorService ex = Executors.newFixedThreadPool(1);
private Random rand = new Random(47);
private void pause(int factor) {
try {
TimeUnit.MILLISECONDS.sleep(100 + rand.nextInt(factor));
} catch (InterruptedException e) {
System.out.println("sleep() interrupted");
}
}
public Future<Integer> calculateInt(final int x, final int y) {
return ex.submit(new Callable<Integer>() {
public Integer call() {
System.out.println(Thread.currentThread().getName() + "->" + "starting " + x + " + " + y);
pause(500);//模拟计算的过程
return x + y;
}
});
}
public Future<Float> calculateFloat(final float x, final float y) {
return ex.submit(new Callable<Float>() {
public Float call() {
System.out.println(Thread.currentThread().getName() + "->" + "starting " + x + " + " + y);
pause(2000);
return x + y;
}
});
}
public void shutdown() {
ex.shutdown();
}
public static void main(String[] args) {
ActiveObjectDemo d1 = new ActiveObjectDemo();
// Prevents ConcurrentModificationException:
List<Future<?>> results = new CopyOnWriteArrayList<Future<?>>();
for (float f = 0.0f; f < 1.0f; f += 0.2f)
results.add(d1.calculateFloat(f, f));
for (int i = 0; i < 5; i++)
results.add(d1.calculateInt(i, i));
System.out.println("All asynch calls made");
//1,results可能添加一个null 但其size为1
while (results.size() > 0) {
for (Future<?> f : results)
//2,不阻塞 没关系,但其是一直遍历的
if (f.isDone()) {
try {
System.out.println("res="+f.get());//4,获取结果的时候去阻塞的,获取完结果再去remove
} catch (Exception e) {
throw new RuntimeException(e);
}
results.remove(f);//3,当把所有结果都移除后 while的size才为0
}
}
d1.shutdown();
}
}