导入所需依赖
<dependencies>
<!-- 接口展示插件 -->
<!-- swagger ui -->
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>${springdoc.version}</version>
</dependency>
<!-- 第三方接口ui展示插件,写此文章时尚未实现openapi3.0协议 -->
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>knife4j-spring-ui</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<fork>true</fork>
</configuration>
<executions>
<execution>
<id>pre-integration-test</id>
<goals>
<goal>start</goal>
</goals>
</execution>
<execution>
<id>post-integration-test</id>
<goals>
<goal>stop</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-maven-plugin</artifactId>
<version>0.2</version>
<executions>
<execution>
<id>integration-test</id>
<goals>
<goal>generate</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
application.yml 配置(可选)
spring:
resources:
cache:
period: 31536000
cachecontrol:
max-age: 31536000
chain:
html-application-cache: true
enabled: true
strategy:
content:
enabled: true
paths: /webjars/swagger-ui/**.*
WebMvcConfigurer 配置
/**
*
* 启动 springMvc自动配置即可,注意若是 extends WebMvcConfigurationSupport,需要自己配置
* 不理解的话请 Google WebMvcConfigurationSupport 和 WebMvcConfigurer 的区别,建议 implements WebMvcConfigurer
*/
@Configuration
public class SystemWebMvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
//上传文件配置
// registry.addResourceHandler("/upload/**").addResourceLocations("file:///" + uploadFilePath);
// spring boot 默认的资源位置配置
registry.addResourceHandler("/**").addResourceLocations("classpath:/static/", "classpath:/public/",
"classpath:/resources/", "classpath:/META-INF/resources/","/");// 静态资源路径
// swagger3 配置
registry.addResourceHandler("/webjars/**").addResourceLocations("classpath:/META-INF/resources/webjars/");
registry.addResourceHandler("/**/swagger-ui/**").addResourceLocations("/webjars/");
registry.addResourceHandler("/**/favicon.ico").addResourceLocations("classpath:/**/favicon.ico");
// registry.addResourceHandler("/webjars/**").addResourceLocations("/webjars/").resourceChain(false)
// .addResolver(new WebJarsResourceResolver()).addResolver(new PathResourceResolver());
}
}
统一返回数据格式配置
import org.springframework.core.MethodParameter;
import org.springframework.web.context.request.NativeWebRequest;
import org.springframework.web.method.support.HandlerMethodReturnValueHandler;
import org.springframework.web.method.support.ModelAndViewContainer;
public class HandlerMethodReturnValueHandlerProxy implements HandlerMethodReturnValueHandler {
private static final int STATUS_CODE_SUCCEEDED = 200;
//返回对象处理方法所在类的白名单
private String[] whiteNames = new String[]{"org.springdoc."};
private HandlerMethodReturnValueHandler proxyObject;
public HandlerMethodReturnValueHandlerProxy(HandlerMethodReturnValueHandler proxyObject) {
this.proxyObject = proxyObject;
}
@Override
public boolean supportsReturnType(MethodParameter returnType) {
return proxyObject.supportsReturnType(returnType);
}
@Override
public void handleReturnValue(Object returnValue, MethodParameter returnType, ModelAndViewContainer mavContainer,
NativeWebRequest webRequest) throws Exception {
boolean isProxy = true;
for (String whiteName : whiteNames) {
if (returnType.getDeclaringClass().getName().contains(whiteName)) {
isProxy = false;
break;
}
}
if (isProxy) {
R hs = new R(returnValue);
proxyObject.handleReturnValue(hs, returnType, mavContainer, webRequest);
} else {
proxyObject.handleReturnValue(returnValue, returnType, mavContainer, webRequest);
}
}
}
验证
打开浏览器 http://127.0.0.1/swagger-ui.html
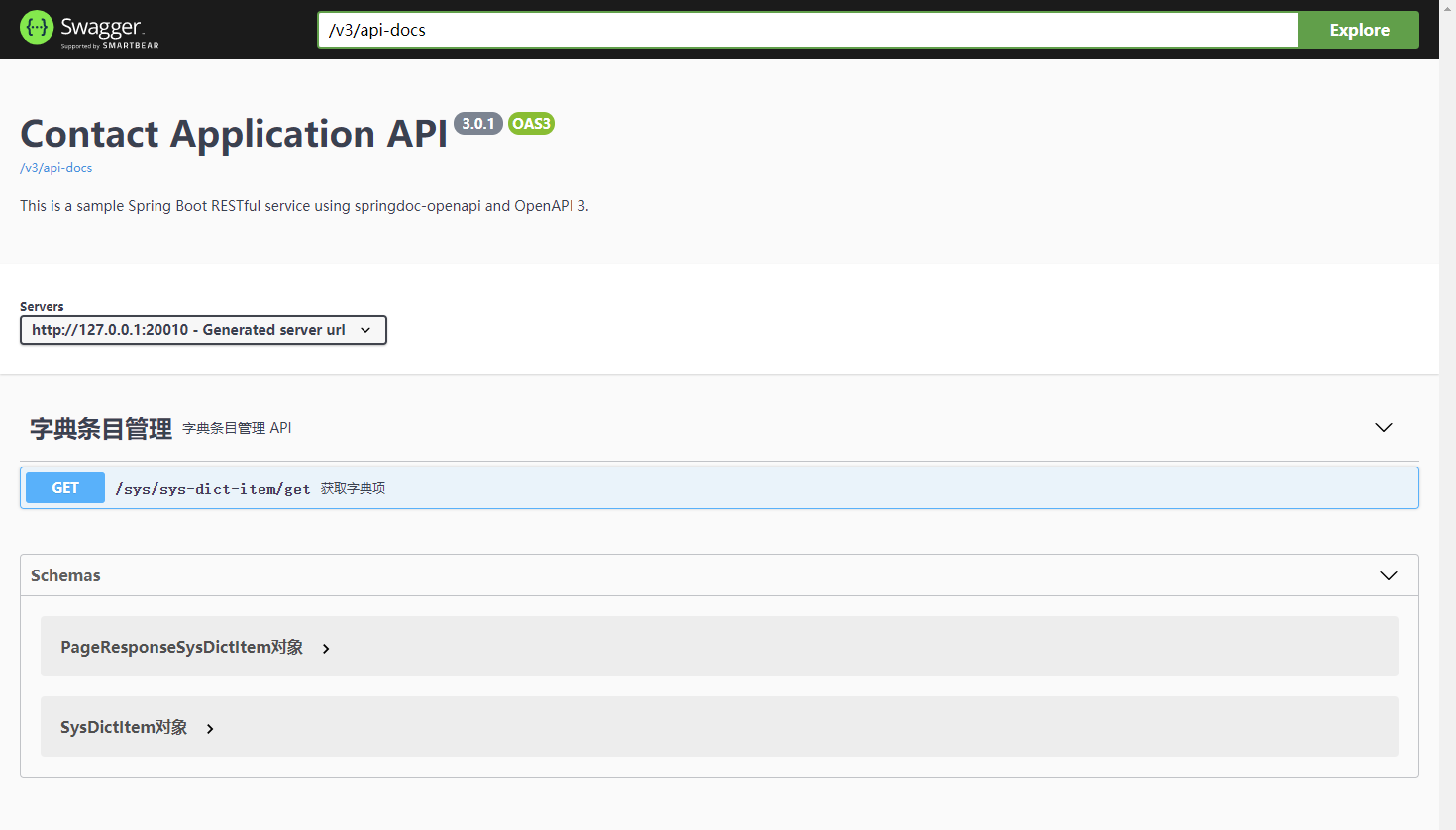