1.异常概述与异常体系结构
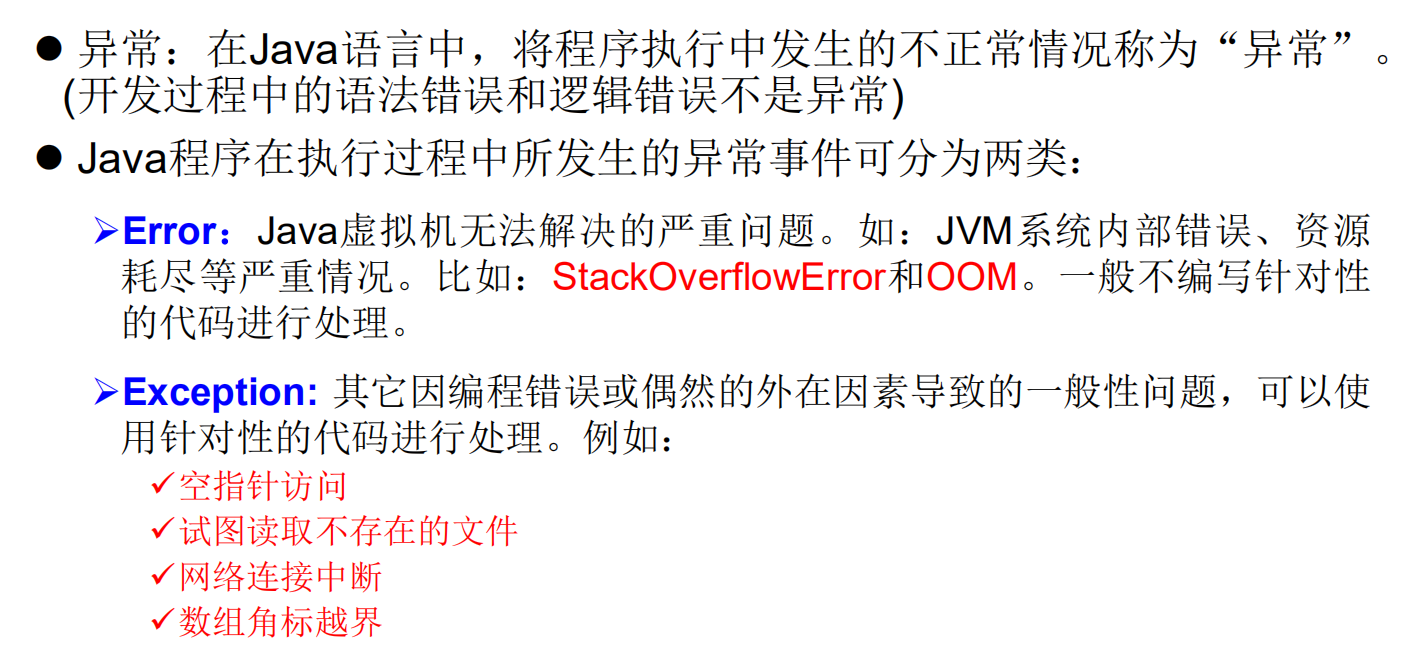
2.常见异常
package com.atguigu.java;
import java.io.File;
import java.util.Date;
import java.util.Scanner;
import org.junit.Test;
/*
* 一、异常体系结构
*
* java.lang.Throwable
* |------java.lang.Error:一般不便携针对性的代码进行处理
* |------java.lang.Exception:可以进行异常的处理
* |------编译时异常(check)
* |-------IOException
* |------FileNotFoundException
*
* |-------ClassNotFoundException
*
* |------运行时异常(uncheck)
* |-------NullPointException
* |-------ArrayIndexOutOfBoundsException
* |-------ClassCastException
* |-------NumberFormatException
* |-------InputMismatchException
* |-------ArithmeticException
* .
* .
* .
*
*/
public class ExceptionTest {
//******************以下是编译时异常***************************
@Test
public void test7(){
File file =new File("hello.txt");
见图片代码
}
//*******************以下是运行时异常***************************
//ArithmeticException算术异常
@Test
public void test6(){
// int a=10;
// int b=0;
// System.out.println(a/b);
}
//InputMismatchException 输入不匹配
@Test
public void test5(){
//当输入是一个int形的,那就正常输出,如果输入不一致类型的就会报错
Scanner scanner =new Scanner(System.in);
// int score =scanner.nextInt();
// System.out.println(score);
scanner.close();
}
//NumberFormatException
@Test
public void test4(){
String string ="123";
String s1="abc";
// int num =Integer.parseInt(s1);
}
//ClassCastException 类型转换异常
@Test
public void test3(){
// Object object=new Date();
// String string =(String)object;
}
//ArrayIndexOutOfBoundsException //数组角标越界
@Test
public void test2(){
// int[] arr =new int[3];
// System.out.println(arr[4]);
//StringIndexOutOfBoundsException //字符串角标越界
// String string ="abc";
// System.out.println(string.charAt(3));
//
}
//NullPointException 空指针异常
@Test
public void test1(){
// int[] arr =new int[3];
// System.out.println(arr[3]);
}
}
3.异常处理机制一:try-catch-finally
3.1try-catch的使用
package com.atguigu.java;
import org.junit.Test;
/*
* 一、异常的处理:抓抛模型
*
* 过程一:“抛”:程序在正常执行的过程中,一旦出现异常,就会在异常代码处生成一个对应异常类的对象
* 并将此对象抛出。
* 一旦抛出对象以后,其后的代码就不再执行。
*
* 过程二:“抓”:可以理解为异常的处理方式:①try-catch-finally ②throws
*
* 二、try-catch-finally的使用
*
* try{
* //可能出现异常的代码
*
* }catch(异常类型1 变量名1){
*
* //处理异常的方式1
* }
* catch(异常类型2 变量名2){
*
* //处理异常的方式2
* }
* catch(异常类型3 变量名3){
*
* //处理异常的方式3
* }
*.......
* finally{
*
* //一定会执行的代码
* }
*
* 说明:
* 1.finally是可选的
* 2.使用try将可能出现异常代码包装起来,在执行过程中,一旦出现异常,就会生成一个对应的异常类的对象,根据此对象的类型
* 去catch中进行匹配
* 3.一旦try的异常对象匹配到某一个catch时,就进入catch中进行异常的处理。一旦处理完成 ,就跳出try-catch结构
* (在没有写finally的情况下)继续执行其后的代码
*
* 4.catch中的异常没有子父类关系,则谁声明再上,谁在下都无所谓
* catch中的异常类型如果满足子父类关系,则要求子类一定要声明在父类之上,否则,报错
* 5.常用的异常对象处理的方式:① String getMessage() ②printStackTrace();
* 6.在try结构中声明的变量,在出了try结构以后,就不能再被调用
*
* 体会:使用try-catch-finally 处理编译时异常,是程序在编译时不再报错,但是运行时仍可能报错
* 相当于我们使用try-catch-finally将一个编译时可能出现的异常,延迟到运行时出现
*/
public class ExceptionTest1 {
@Test
public void test4(){
String string ="123";
String s1="abc";
int num=0;
try{
System.out.println("hello------1");
num =Integer.parseInt(s1);
//抓到抛出的异常后,就会去执行catch语句
System.out.println("hello------2");
}catch(NumberFormatException e){
// System.out.println("出现数值转换异常,不要着急......");
//使用string getMessage();
// System.out.println(e.getMessage());
//使用printStackTrace()
e.printStackTrace();
}
catch(NullPointerException e){
System.out.println("出现空指针异常,不要着急......");
}
catch(Exception e){
System.out.println("出现异常,不要着急......");
}
System.out.println(num);
}
}
3.2finally的使用
package com.atguigu.java;
import org.junit.Test;
/*
* 一、异常的处理:抓抛模型
*
* 过程一:“抛”:程序在正常执行的过程中,一旦出现异常,就会在异常代码处生成一个对应异常类的对象
* 并将此对象抛出。
* 一旦抛出对象以后,其后的代码就不再执行。
*
* 过程二:“抓”:可以理解为异常的处理方式:①try-catch-finally ②throws
*
* 二、try-catch-finally的使用
*
* try{
* //可能出现异常的代码
*
* }catch(异常类型1 变量名1){
*
* //处理异常的方式1
* }
* catch(异常类型2 变量名2){
*
* //处理异常的方式2
* }
* catch(异常类型3 变量名3){
*
* //处理异常的方式3
* }
*.......
* finally{
*
* //一定会执行的代码
* }
*
* 说明:
* 1.finally是可选的
* 2.使用try将可能出现异常代码包装起来,在执行过程中,一旦出现异常,就会生成一个对应的异常类的对象,根据此对象的类型
* 去catch中进行匹配
* 3.一旦try的异常对象匹配到某一个catch时,就进入catch中进行异常的处理。一旦处理完成 ,就跳出try-catch结构
* (在没有写finally的情况下)继续执行其后的代码
*
* 4.catch中的异常没有子父类关系,则谁声明再上,谁在下都无所谓
* catch中的异常类型如果满足子父类关系,则要求子类一定要声明在父类之上,否则,报错
* 5.常用的异常对象处理的方式:① String getMessage() ②printStackTrace();
* 6.在try结构中声明的变量,在出了try结构以后,就不能再被调用
*
* 体会1:使用try-catch-finally 处理编译时异常,是程序在编译时不再报错,但是运行时仍可能报错
* 相当于我们使用try-catch-finally将一个编译时可能出现的异常,延迟到运行时出现
*
* 体会2:开发中,由于运行时异常,所以我们通常就不针对运行时异常编写try-catch-finally了。
* 针对于编译时异常,我们说一定要考虑异常的处理。
*
*/
public class ExceptionTest1 {
@Test
public void test4(){
String string ="123";
String s1="abc";
int num=0;
try{
System.out.println("hello------1");
num =Integer.parseInt(s1);
//抓到抛出的异常后,就会去执行catch语句
System.out.println("hello------2");
}catch(NumberFormatException e){
// System.out.println("出现数值转换异常,不要着急......");
//使用string getMessage();
// System.out.println(e.getMessage());
//使用printStackTrace()
e.printStackTrace();
}
catch(NullPointerException e){
System.out.println("出现空指针异常,不要着急......");
}
catch(Exception e){
System.out.println("出现异常,不要着急......");
}
System.out.println(num);
}
}
4.异常处理机制二:throws
4.1异常处理的方式二:throws+异常类型
package com.atguigu.java;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.nio.file.FileAlreadyExistsException;
/*
* 异常处理的方式二:throws+异常类型
*
* 1."throws+异常类型"写在方法的声明处,指明此方法执行时,可能会抛出的异常类型
* 一旦当方法体执行时,出现异常,仍会在异常代码处生成一个异常类的对象,此对象满足
* throws后异常类型时,就会被抛出,异常代码的后续代码将不再被执行
*
* 2.体会:try-catch-finally:真正的将异常给处理掉了
* throws的方式只是将异常抛给了方法的调用者,并没有将异常真正的处理掉
*
* 3.开发中如何选择使用try—catch-finally 还是使用throws?
* 3.1 如果父类中被重写的方法没有throws方法处理异常,则子类重写的方法也不能使用throws,意味着如果
* 子类重写的方法中有异常,必须使用try-catch-finally方式处理
* 3.2执行的方法a中,先后又调用了另外的几个方法,这几个方法是递进关系执行的。我们建议这几个方法使用throws的方式进行处理
* 而执行的方法a可以考虑使用try-catch-finally进行处理
*
*/
public class ExceptionTest2 {
public static void main(String[] args){
try {
method2();
} catch (IOException e) {
e.printStackTrace();
}
method3();
}
public static void method3(){
try {
method2();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void method2() throws FileNotFoundException,IOException{
method1();
}
public static void method1() throws FileNotFoundException,IOException{
File file =new File("hello.txt");
FileInputStream fis =new FileInputStream(file);
int data =fis.read();
while(data!=-1){
System.out.println((char)data);
data=fis.read();
}
fis.close();
}
}
4.2异常方法重写的规则
package com.atguigu.java;
import java.io.FileNotFoundException;
import java.io.IOException;
/*
* 方法重写的规则之一:
* 子类重写的方法抛出的异常类型不大于父类被重写的方法抛出的异常类型
*
*
*
*
*/
public class OverrideTest {
public static void main(String[] args) {
OverrideTest test =new OverrideTest();
test.display(new SubClass());
}
public void display(SuperClass s){
try {
s.method();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class SuperClass{
public void method()throws IOException{
}
}
class SubClass extends SuperClass{
public void method() throws FileNotFoundException{
}
}
5.手动抛出异常:throw
package com.atguigu.java;
import javax.management.RuntimeErrorException;
public class throwhand {
public static void main(String[] args) throws Exception {
Student student=new Student();
student.regist(-1001);
System.out.println(student);
}
@Override
public String toString() {
return "throwhand [getClass()=" + getClass() + ", hashCode()="
+ hashCode() + ", toString()=" + super.toString() + "]";
}
}
class Student{
private int id;
public void regist(int id) throws Exception{
if(id>0){
this.id =id;
}else{
// System.out.println("您输入的数据非法!");
//手动抛出异常对象
// throw new RuntimeException("您输入的数据非法!");
throw new Exception("您输入的数据非法!");
}
}
}
6.用户自定义异常类