基本应用
package com.hxw;
import javafx.application.Application;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.stage.Stage;
public class TestListView extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
ListView<String> listView = new ListView<>();
listView.setItems(FXCollections.observableArrayList("小明","小红","小黄"));
listView.getSelectionModel().selectedItemProperty().addListener(new ChangeListener<String>() {
@Override
public void changed(ObservableValue<? extends String> observable, String oldValue, String newValue) {
System.out.println("选中的item为"+newValue);
}
});
primaryStage.setHeight(400);
primaryStage.setWidth(400);
primaryStage.setScene(new Scene(new Group(listView)));
primaryStage.show();
}
public static void main(String[] args) {
launch();
}
}
Item的类型不为String的解决方案
- 设置ListCell ```java package com.hxw;
import javafx.application.Application; import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.collections.FXCollections; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.ListCell; import javafx.scene.control.ListView; import javafx.stage.Stage; import javafx.util.Callback;
public class TestListView extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
ListView<People> listView = new ListView<>();
listView.setItems(FXCollections.observableArrayList(new People("小明",12),new People("小红",10),new People("小率",18)));
listView.getSelectionModel().selectedItemProperty().addListener(new ChangeListener<People>() {
@Override
public void changed(ObservableValue<? extends People> observable, People oldValue, People newValue) {
System.out.println("选中的item为"+newValue.name + newValue.year);
}
});
listView.setCellFactory(new Callback<ListView<People>, ListCell<People>>() {
@Override
public ListCell<People> call(ListView<People> param) {
return new PeopleListCell();
}
});
primaryStage.setHeight(400);
primaryStage.setWidth(400);
primaryStage.setScene(new Scene(new Group(listView)));
primaryStage.show();
}
public static void main(String[] args) {
launch();
}
class PeopleListCell extends ListCell<People> {
@Override
protected void updateItem(People item, boolean empty) {
super.updateItem(item, empty);
if (item == null){
this.setText("");
}else{
this.setText(item.name+" "+item.year);
}
}
}
class People{
public String name;
public int year;
public People(String name, int year) {
this.name = name;
this.year = year;
}
}
}
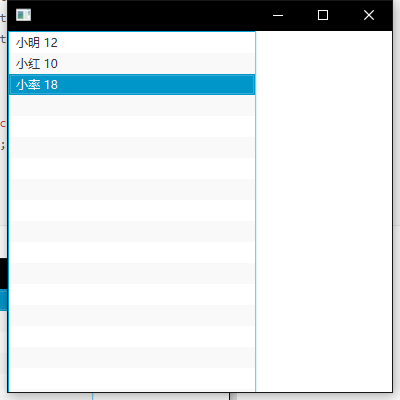<br />[TestListView.java](https://www.yuque.com/attachments/yuque/0/2020/java/645770/1605621221823-b36f156e-0438-4d52-8f1b-65c3b0bd1cc2.java?_lake_card=%7B%22uid%22%3A%221605621219593-0%22%2C%22src%22%3A%22https%3A%2F%2Fwww.yuque.com%2Fattachments%2Fyuque%2F0%2F2020%2Fjava%2F645770%2F1605621221823-b36f156e-0438-4d52-8f1b-65c3b0bd1cc2.java%22%2C%22name%22%3A%22TestListView.java%22%2C%22size%22%3A2140%2C%22type%22%3A%22%22%2C%22ext%22%3A%22java%22%2C%22progress%22%3A%7B%22percent%22%3A99%7D%2C%22status%22%3A%22done%22%2C%22percent%22%3A0%2C%22id%22%3A%22UaOTO%22%2C%22card%22%3A%22file%22%7D)
<a name="C3EEb"></a>
## Item为多个控件
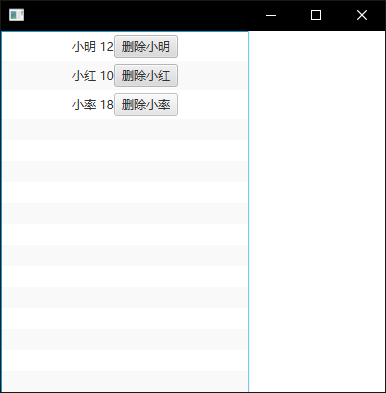
```java
import javafx.application.Application;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.collections.FXCollections;
import javafx.geometry.Pos;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.ListCell;
import javafx.scene.control.ListView;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
import javafx.util.Callback;
public class TestListView extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
ListView<People> listView = new ListView<>();
listView.setItems(FXCollections.observableArrayList(new People("小明",12),new People("小红",10),new People("小率",18)));
listView.getSelectionModel().selectedItemProperty().addListener(new ChangeListener<People>() {
@Override
public void changed(ObservableValue<? extends People> observable, People oldValue, People newValue) {
System.out.println("选中的item为"+newValue.name + newValue.year);
}
});
listView.setCellFactory(new Callback<ListView<People>, ListCell<People>>() {
@Override
public ListCell<People> call(ListView<People> param) {
return new PeopleListCell();
}
});
primaryStage.setHeight(400);
primaryStage.setWidth(400);
primaryStage.setScene(new Scene(new Group(listView)));
primaryStage.show();
}
public static void main(String[] args) {
launch();
}
class PeopleListCell extends ListCell<People> {
@Override
protected void updateItem(People item, boolean empty) {
super.updateItem(item, empty);
if (item == null){
this.setText("");
}else{
HBox hBox = new HBox();
hBox.setAlignment(Pos.CENTER);
Label info = new Label(item.name+" "+item.year);
Button delete = new Button("删除"+item.name);
hBox.getChildren().addAll(info,delete);
this.setGraphic(hBox);//核心就是这一句了
}
}
}
class People{
public String name;
public int year;
public People(String name, int year) {
this.name = name;
this.year = year;
}
}
}