目录结构
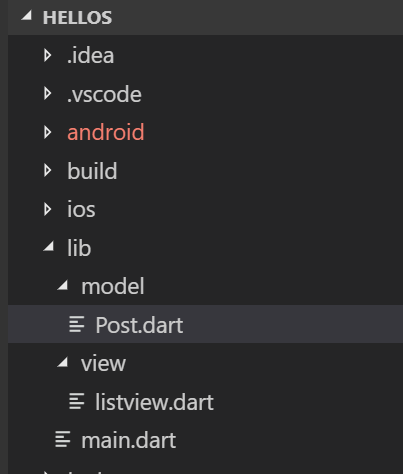
main.dart
import 'package:flutter/material.dart';
import './model/Post.dart';
/*
* 导入默认flutter文件中的material,Google公司推荐的规范
* 使用dart语言,多用dart后缀文件名
*/
//基本main函数
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
//生成一个Material风格的应用
return MaterialApp(
//关掉右上角debug标志
debugShowCheckedModeBanner: false,
//指定默认加载的部件
home: Home(),
//设置主题
theme: ThemeData(
primarySwatch: Colors.deepOrange,
));
}
}
//自定义主页面列表部件
class Home extends StatelessWidget {
//构建视图的方法
Widget _listItemBuilder(BuildContext context, int index) {
//容器部件
return Container(
color: Colors.white,
//设置Container外边距,在四周添加8.0
margin: EdgeInsets.all(8.0),
//Column部件E
child: Column(
children: <Widget>[
//图像部件,使用网络引用
Image.network(posts[index].imageUrl),
//部件间间距
SizedBox(height: 16.0),
Text(posts[index].title, style: Theme.of(context).textTheme.title),
SizedBox(
height: 16.0,
),
Text(posts[index].author, style: Theme.of(context).textTheme.subhead),
SizedBox(
height: 16.0,
),
],
),
);
}
//作为方法返回的
@override
Widget build(BuildContext context) {
//提供一个界面基本结构,顶部工具栏,标签,底部导航栏等
return Scaffold(
//设置背景颜色
backgroundColor: Colors.grey[100],
// 顶部工具栏
appBar: AppBar(
//添加页面标题
title: Text('gaox'),
elevation: 0.0,
),
//主体内容:加上一个列表视图
body: ListView.builder(
itemCount: posts.length,
itemBuilder: _listItemBuilder,
));
}
}
class FirstPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: Text(
'hello\n net',
//设置文字阅读方向
textDirection: TextDirection.ltr,
//定制文字样式
style: TextStyle(
//设置颜色
color: Colors.black87,
//设置文字颜色
fontSize: 80,
//设置文字样式
fontWeight: FontWeight.bold,
),
),
);
}
}
post.dart
class Post {
const Post({
this.title,
this.author,
this.imageUrl,
});
final String title;
final String author;
final String imageUrl;
}
final List<Post> posts = [
Post(
title: 'first',
author: 'gaox',
imageUrl: 'http://p0.so.qhimgs1.com/bdr/720__/t011ba6d48745e60340.jpg',
),Post(
title: 'second',
author: 'gaoxizhi',
imageUrl: 'http://p0.so.qhimgs1.com/bdr/790__/t017632d9ebb249e2ba.jpg',
),Post(
title: 'thirdly',
author: 'heia',
imageUrl: 'http://p2.so.qhimgs1.com/bdr/921__/t0145f2481a8b35382e.jpg',
),
];
页面效果
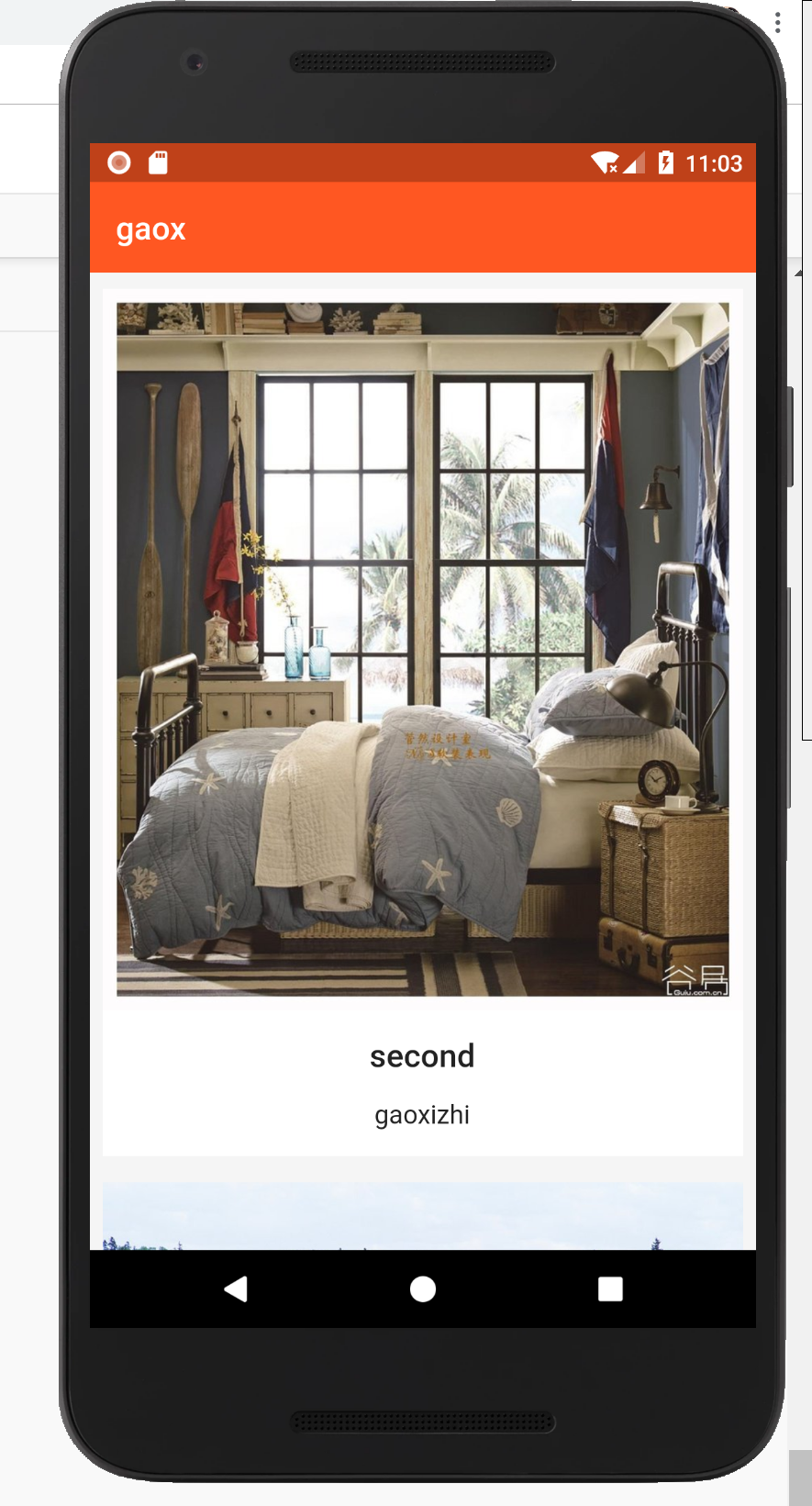