管道模式也叫流水线模式,对于管道模式来说,有三个对象,管道,载荷,过滤器(阀门)<br />再管道种对附载进行一系列的处理,因为可以对过滤器进行动态添加,所以对负载的处理可以变得更加灵活<br />但同时过滤器过多,很淡把握整体的处理逻辑,而且再某一个过滤器对载荷处理后,因为载荷改变,会造成下一个过滤器种的逻辑出现问题<br />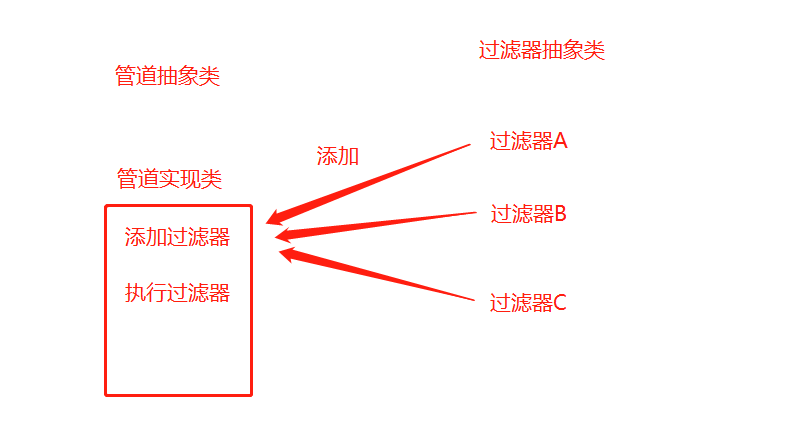
<?php
/**
* 管道抽象类
*/
interface PipelineInterface
{
//数据载荷
public function __construct($payLoad);
//添加过滤器
public function pipe(StageInterface $stage);
//执行
public function process();
}
/**
* 过滤器抽象类
*/
interface StageInterface
{
//处理逻辑
public function handle($payLoad);
}
/**
* 过滤器A
*/
class StageA implements StageInterface
{
public function handle($payLoad)
{
echo "StageAddHundred<pre>";
return $payLoad + 100;
}
}
class StageB implements StageInterface
{
public function handle($payLoad)
{
echo "StageMultiHundred<pre>";
return $payLoad * 100;
}
}
/**
* 管道实现类
*/
class Pipeline implements PipelineInterface
{
private $pipes;
private $payLoad;
public function __construct($payLoad)
{
$this->payLoad = $payLoad;
}
public function pipe(StageInterface $stage)
{
$this->pipes[] = $stage;
return $this;
}
public function process()
{
foreach ($this->pipes as $eachPipe) {
//执行类方法
$this->payLoad = call_user_func([
$eachPipe,
'handle'
], $this->payLoad);
}
return $this->payLoad;
}
}
//操作类
class Test
{
public function run()
{
$payLoad = 10;
$StageA = new StageA();
$StageB = new StageB();
$pipe = new Pipeline($payLoad);
return $pipe->pipe($StageA)
->pipe($StageB)
->process();
}
}
$test = new Test();
echo '<pre>';
var_dump($test->run());