#include <stdio.h>
#include <conio.h>
#define __STDC_CONSTANT_MACROS
#ifdef _WIN32
extern "C"{
#include "libavformat/avformat.h"
};
#else
#ifdef __cplusplus
extern "C"{
#endif
#include <libavformat/avformat.h>
#ifdef __cplusplus
};
#endif
#endif
#define errReport(info, val) do{ \
fprintf(stderr, "ERR:: %s %s(line=%d) code=%d\n", __FUNCTION__, info, __LINE__, val);\
getch(); exit(0);\
}while (0);
AVOutputFormat *ofmt_a = NULL, *ofmt_v = NULL;
AVFormatContext *ifmt_ctx = NULL, *ofmt_ctx_a = NULL, *ofmt_ctx_v = NULL;
AVPacket pkt;
int ret, i;
int videoindex = -1, audioindex = -1;
int frame_index = 0;
const char *in_filename = "in.ts";
const char *out_filename_v = "out.h264";
const char *out_filename_a = "out.aac";
int init_demuxer() {
av_register_all();
if (avformat_open_input(&ifmt_ctx, in_filename, 0, 0) < 0)
return -1;
if (avformat_find_stream_info(ifmt_ctx, 0) < 0)
return -2;
avformat_alloc_output_context2(&ofmt_ctx_v, NULL, NULL, out_filename_v);
if (!ofmt_ctx_v) return -3;
ofmt_v = ofmt_ctx_v->oformat;
avformat_alloc_output_context2(&ofmt_ctx_a, NULL, NULL, out_filename_a);
if (!ofmt_ctx_a) return -4;
ofmt_a = ofmt_ctx_a->oformat;
for (i = 0; i < ifmt_ctx->nb_streams; i++) {
AVFormatContext *ofmt_ctx;
AVStream *in_stream = ifmt_ctx->streams[i];
AVStream *out_stream = NULL;
if (ifmt_ctx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO) {
videoindex = i;
out_stream = avformat_new_stream(ofmt_ctx_v, in_stream->codec->codec);
ofmt_ctx = ofmt_ctx_v;
}
else if (ifmt_ctx->streams[i]->codec->codec_type == AVMEDIA_TYPE_AUDIO) {
audioindex = i;
out_stream = avformat_new_stream(ofmt_ctx_a, in_stream->codec->codec);
ofmt_ctx = ofmt_ctx_a;
}
else {
break;
}
if (!out_stream) return -5;
if (avcodec_copy_context(out_stream->codec, in_stream->codec) < 0)
return -6;
out_stream->codec->codec_tag = 0;
if (ofmt_ctx->oformat->flags & AVFMT_GLOBALHEADER)
out_stream->codec->flags |= CODEC_FLAG_GLOBAL_HEADER;
}
printf("================Input===============\n");
av_dump_format(ifmt_ctx, 0, in_filename, 0);
printf("================Output==============\n");
av_dump_format(ofmt_ctx_v, 0, out_filename_v, 1);
printf("++++++++++++++++++++++++++++++++++++\n");
av_dump_format(ofmt_ctx_a, 0, out_filename_a, 1);
printf("====================================\n");
if (!(ofmt_v->flags & AVFMT_NOFILE)) {
if (avio_open(&ofmt_ctx_v->pb, out_filename_v, AVIO_FLAG_WRITE) < 0)
return -7;
}
if (!(ofmt_a->flags & AVFMT_NOFILE)) {
if (avio_open(&ofmt_ctx_a->pb, out_filename_a, AVIO_FLAG_WRITE) < 0)
return -8;
}
if (avformat_write_header(ofmt_ctx_v, NULL) < 0)
return -9;
if (avformat_write_header(ofmt_ctx_a, NULL) < 0)
return -10;
return 0;
}
int main(int argc, char* argv[]){
if ((ret = init_demuxer()) < 0)
errReport("init_demuxer", ret);
while (1) {
AVFormatContext *ofmt_ctx;
AVStream *in_stream, *out_stream;
if (av_read_frame(ifmt_ctx, &pkt) < 0)
break;
in_stream = ifmt_ctx->streams[pkt.stream_index];
if (pkt.stream_index == videoindex) {
out_stream = ofmt_ctx_v->streams[0];
ofmt_ctx = ofmt_ctx_v;
printf("\nv#s:%d\tp:%lld", pkt.size, pkt.pts);
}
else if (pkt.stream_index == audioindex) {
out_stream = ofmt_ctx_a->streams[0];
ofmt_ctx = ofmt_ctx_a;
printf("a#s:%d\tp:%lld", pkt.size, pkt.pts);
}
else {
continue;
}
pkt.pts = av_rescale_q_rnd(pkt.pts, in_stream->time_base, out_stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX));
pkt.dts = av_rescale_q_rnd(pkt.dts, in_stream->time_base, out_stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX));
pkt.duration = av_rescale_q(pkt.duration, in_stream->time_base, out_stream->time_base);
pkt.pos = -1;
pkt.stream_index = 0;
if (av_interleaved_write_frame(ofmt_ctx, &pkt) < 0) {
printf("Error muxing packet\n");
break;
}
//printf("Write %8d frames to output file\n",frame_index);
av_free_packet(&pkt);
frame_index++;
}
av_write_trailer(ofmt_ctx_a);
av_write_trailer(ofmt_ctx_v);
avformat_close_input(&ifmt_ctx);
if (ofmt_ctx_a && !(ofmt_a->flags & AVFMT_NOFILE))
avio_close(ofmt_ctx_a->pb);
if (ofmt_ctx_v && !(ofmt_v->flags & AVFMT_NOFILE))
avio_close(ofmt_ctx_v->pb);
avformat_free_context(ofmt_ctx_a);
avformat_free_context(ofmt_ctx_v);
if (ret < 0 && ret != AVERROR_EOF) {
printf("Error occurred.\n");
return -1;
}
printf("successed.\n");
getch();
return 0;
}
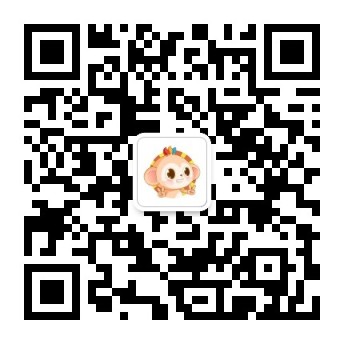