1-1 构造属性
## 创建构造函数的时候,原型对象上会有一个constructor属性,它是原型对象所独有的,它指回构造函数本身
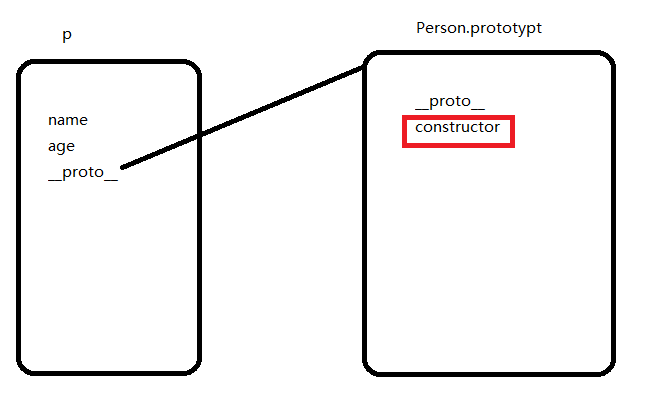
function Person(name,age){
this.name = name
this.age = age
}
var p = new Person("cheng",20)
console.log(p.constructor);
console.log(p.constructor == Person); // true
var arr = [1,2,3]
console.log(arr.constructor == Array); // true
1-2 给原型添加属性 ( 直接量形式 )
# 问题:我们以直接量(对象)形式,给原型添加属性的时候,它的constructor会指向Object
# 解决:重置 constructor
function Person(name,age){
this.name = name
this.age = age
}
Person.prototype = {
sayName:function(){
console.log(this.name);
},
sayAge(){
console.log(this.age);
}
}
var p = new Person("cheng",20)
console.log(p.constructor); //Object
console.log(p.constructor == Person); // false
console.log(p instanceof Person); // true
## 解决办法
Person.prototype = {
constructor:Person, // 重置 constructor
sayName:function(){
console.log(this.name);
},
sayAge(){
console.log(this.age);
}
}
var p = new Person("cheng",20)
console.log(p.constructor); // Person
console.log(p.constructor == Person); // true
1-3 公有属性和私有属性
# 公有属性: 一般在原型对象上
# 私有属性: 通过this关键字去添加的
1-3-1 hasOwnProperty: 可以判断属性是私有的还是公有的
function Person(name,age){
this.name = name
this.age = age
}
Person.prototype = {
constructor:Person,
sayName:function(){
console.log(this.name);
},
sayAge(){
console.log(this.age);
}
}
var p = new Person("cheng",20)
console.log(p.hasOwnProperty("name")); // true 私有的
console.log(p.hasOwnProperty("sayName")); // false 公有的
//判断constructor是否是原型对象的私有属性
console.log(Person.prototype.hasOwnProperty("constructor")); // true
1-3-2 in 判断对象是否包含这个属性,包括(私有和公有)
in 和 hasOwnProperty 区别
in:判断对象是否包含这个属性
hasOwnProperty:判断是否是私有属性
var person1 = {
name: "Nicholas",
sayName: function() {
console.log(this.name);
}
};
console.log("name" in person1); // true
console.log(person1.hasOwnProperty("name")); // true
console.log("toString" in person1); // true
console.log(person1.hasOwnProperty("toString")); // false
1-3-3 鉴别一个属性是否是原型属性
var book = {
title: "The Principles of Object-Oriented JavaScript"
};
function hasPrototypeProperty(object, name) {
return name in object &&!object.hasOwnProperty(name);
}
console.log(hasPrototypeProperty(book, "title")); // false
console.log(hasPrototypeProperty(book, "hasOwnProperty")); // true