package work.artorias.test.lambda;
import java.util.Comparator;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* 拉姆达表达式基础学习
* @author LJ
*/
public class LambdaBase {
/**
* 总结: 拉姆达表达式就是作为方法入参的一种简单书写形式,当一个方法入参是一个接口,并且该接口只有一个方法未实现,这种接口叫做函数式接口
* ,就可以使用lambda表达式
* lambda表达式的作用就是匿名实现了这个接口作为入参
*
* 为了方便我们使用JDK提供了许多函数式接口,其中有4中核心接口。以提供更方便的编程
*
*/
/**
*
* @param args
*/
public static void main(String[] args) {
System.out.println("1:");
new LambdaBase().lambdaRule1();
System.out.println("--------------------------------");
System.out.println("2:");
new LambdaBase().lambdaRule2();
System.out.println("--------------------------------");
System.out.println("3:");
new LambdaBase().lambdaRule3();
System.out.println("--------------------------------");
System.out.println("4:");
new LambdaBase().lambdaRule4();
System.out.println("--------------------------------");
System.out.println("5:");
new LambdaBase().lambdaRule5();
System.out.println("--------------------------------");
}
/**
* 无参无返回值
* 方法体只有一行可以省略{}
*/
public void lambdaRule1(){
LambdaTest1 lambdaTest1=()-> System.out.println("无参无返回值方法");
lambdaTest1.a();
}
/**
* 一个参数无返回值
* 参数只有一个可以省略()
*
* Consumer<T> 接口是JDK提供用于使用lambda的接口,他是一个函数式接口,其中只有一个未实现方法。该接口也可以叫做消费者
* void accept(T t);
* 泛型标明了入参类型
*/
public void lambdaRule2(){
Consumer<String> stringConsumer=x->System.out.println(x);
stringConsumer.accept("一个参数无返回值");
}
/**
* 有多个参数,有返回值
* Comparator<T>这是JDK自带的比较器接口,实现该接口的类需要实现一个方法以实现实例的比较,泛型T标明实例类型,
* int compare(T o1, T o2);
*
* 这种就是通用写法,如果只有一条语句return也可以省略
*/
public void lambdaRule3(){
Comparator<Integer>integerComparator=(Integer x,Integer y)->{
System.out.println("x="+x+" "+"y="+y);
return Integer.compare(x,y);
};
int compare = integerComparator.compare(4, 5);
System.out.println(compare);
}
/**
上面的补充,入参类型是可以省略的
*/
public void lambdaRule4(){
Comparator<Integer>integerComparator=(x, y)->{
System.out.println("x="+x+" "+"y="+y);
return Integer.compare(x,y);
};
int compare = integerComparator.compare(4, 5);
System.out.println(compare);
}
/**
* lambda中::的使用
*
* 基本就是如果有某个实现类的方法和你需要写的lambda一样,就可以直接使用
* 就像类名可以直接调用静态方法一样,这里也可以
*
*/
public void lambdaRule5(){
Consumer<String>stringConsumer=System.out::println;
stringConsumer.accept("::的使用");
}
/**
* jdk提供的四大函数式接口
*/
public void functionInterfance(){
/**
*消费型接口
* Consumer<T>
* void accept(T t)
*/
Consumer<String>stringConsumer=x-> System.out.println(x);
/**
*供给型接口
* Supplier<T>
* T get()
*/
Supplier<String>stringSupplier=()->"供给型接口";
/**
*函数式接口
* Function<T, R>
* R apply(T t)
*/
Function<String,Integer>stringIntegerFunction=x->Integer.parseInt(x);
/**
*断言式接口
* Predicate<T>
* boolean test(T t)
*/
Predicate<Integer>integerPredicate=x->x.equals(0)?true:false;
}
interface LambdaTest1{
void a();
}
}
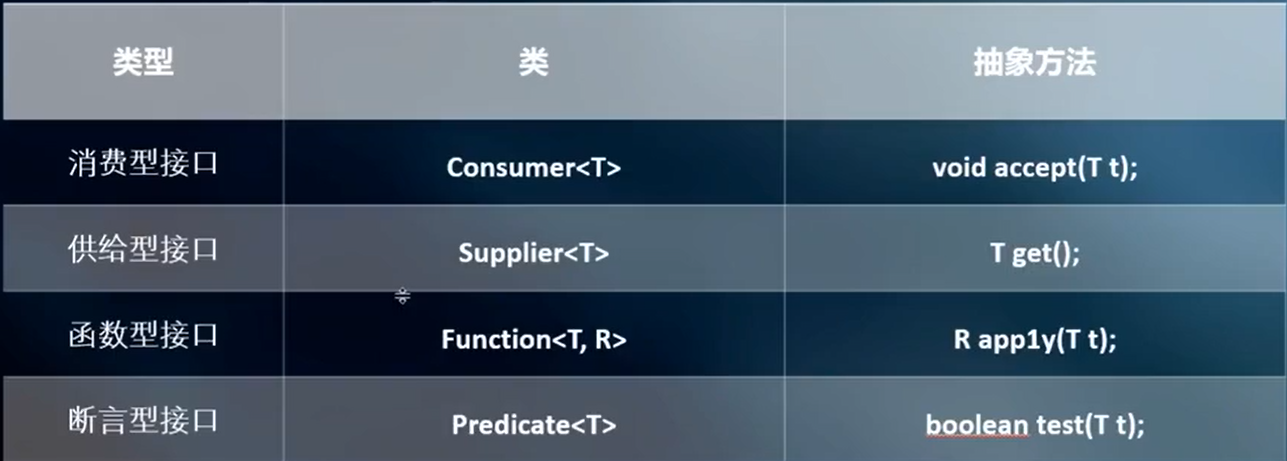