1、新建项目
2、编写Server端
1、创建一个文件夹,然后新建main.dart
2、按下F5后会出现
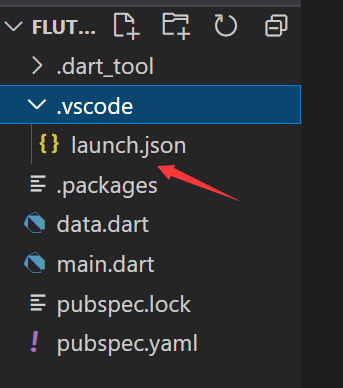
修改里面的内容
3、使用Dart编写一个服务处理
import 'dart:convert';
import 'dart:io';
import 'data.dart';
main() async{
var requestServer = await HttpServer.bind("192.168.51.211", 8080);
print("http服务启动起来了");
//处理请求
await for(HttpRequest request in requestServer){
// request.response..write('request success...')..close();//..是语法糖,表示可以一直请求,不用再写
handleMessage(request);
}
}
void handleMessage(HttpRequest request){
try{
if(request.method == "GET"){
handleGET(request);
}else if(request.method == "POST"){
//TODO
handleGET(request);
}
}catch(e){
print("捕获了一个异常:$e");
}
}
void handleGET(HttpRequest request){
//获取请求参数
var action = request.uri.queryParameters['action'];
if(action == 'getProducts'){
print("获取产品数据...");
request.response..statusCode = HttpStatus.ok
..write(json.encode(products))..close();
}
if(action == 'getNews'){
print("获取新闻数据...");
request.response..statusCode = HttpStatus.ok
..write(json.encode(news))..close();
}
}
void handlePOST(HttpRequest request){
//处理POST请求
}
4、编写data.dart
//产品数据
var products = {
"items":[
{
"desc": "屏幕尺寸: 13.3英寸 处理器: Intel Core i5-8259",
"imageUrl":"assets/images/products/1.jpeg",
"type":"新款",
"name":"苹果笔记本",
},
{
"desc": "处理器:Intel Core i7-5960X 内存大小:32GB 显卡芯片:双NVIDIA GeForce GTX ",
"imageUrl":"assets/images/products/2.jpeg",
"type": "新款",
"point":"强悍",
"name":"外星人笔记本",
},
{
"desc": "Intel,至强处理器E5系列 英特尔C610系列芯片组 机械硬盘:SAS,SATA,近线SAS;固态硬盘:SAS,SATA",
"imageUrl":"assets/images/products/3.jpeg",
"type": "经典",
"point":"稳定性好噪音小",
"name":"戴尔 PowerEdge R730",
},
{
"desc": "屏幕尺寸: 13.3英寸 处理器: Intel Core i5-8259",
"imageUrl":"assets/images/products/1.jpeg",
"type": "新款",
"point":"超轻超薄",
"name":"苹果笔记本",
},
{
"desc": "屏幕尺寸: 13.3英寸 处理器: Intel Core i5-8259",
"imageUrl":"assets/images/products/1.jpeg",
"type": "新款",
"point":"超轻超薄",
"name":"苹果笔记本",
},
{
"desc": "处理器:Intel Core i7-5960X 内存大小:32GB 显卡芯片:双NVIDIA GeForce GTX ",
"imageUrl":"assets/images/products/2.jpeg",
"type": "新款",
"point":"强悍",
"name":"外星人笔记本",
},
{
"desc": "Intel,至强处理器E5系列 英特尔C610系列芯片组 机械硬盘:SAS,SATA,近线SAS;固态硬盘:SAS,SATA",
"imageUrl":"assets/images/products/3.jpeg",
"type": "经典",
"point":"稳定性好噪音小",
"name":"戴尔 PowerEdge R730",
},
{
"desc": "屏幕尺寸: 13.3英寸 处理器: Intel Core i5-8259",
"imageUrl":"assets/images/products/1.jpeg",
"type": "新款",
"point":"超轻超薄",
"name":"苹果笔记本",
},
]
};
var news = {
"items":[
{
"author":"张小平",
"title":"添加商品",
"content":"小张添加了一个关于服务器的商品"
},
{
"author": "陈软发",
"title":"好消息",
"content": "公司服务器全年成功销售1万台",
},
{
"author": "李东升",
"title":"收集商品信息",
"content": "销售人员开始找每个商家收集商品信息,包括商品名称,价格,活动等",
},
]
};
测试请求
import 'dart:convert';
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: RaisedButton(
child: Text("按钮"),
onPressed: () async {
String url = "http://192.168.51.211:8080/?action=getProducts";
var res = await http.get(url);
var body = res.body;
print("body = $body");
var json = jsonDecode(body);
},
),
),
);
}
}