Coroutine
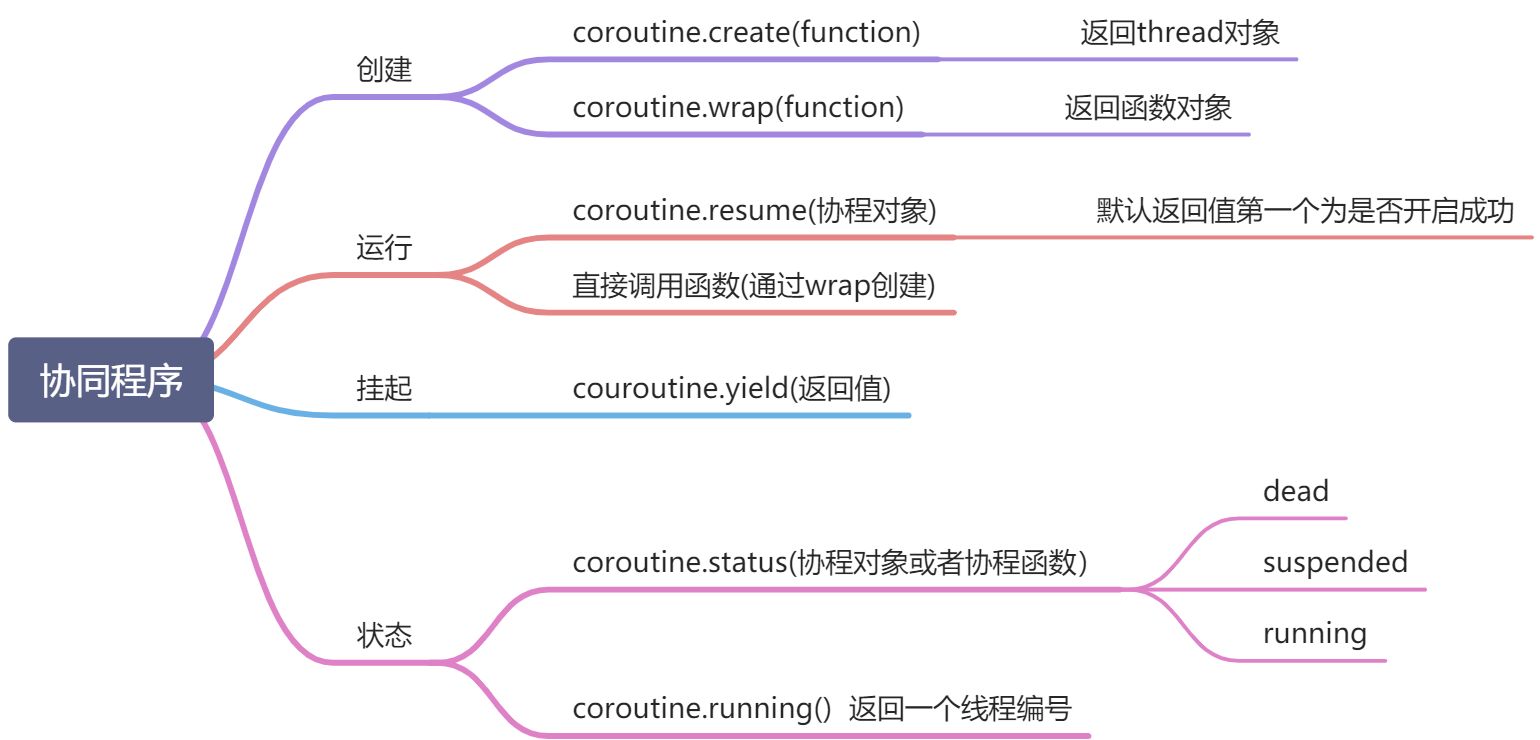
创建协程
-- *** 协程的创建 *** --
fun = function()
print(123)
end
-- 常用方式
co = coroutine.create(fun)
-- 协程的本质是一个线程对象
print(co) --> thread: 00000000007ce248
print(type(co)) --> thread
co2 = coroutine.wrap(fun)
print(co2) --> function: 0000000000a39720
print(type(co2)) --> function
-- *** 协程的运行 *** --
-- 第一种方式 对应 通过create创建的协程
coroutine.resume(co) --> 123
-- 第二种方式 对应 通过wrap创建的协程
co2() --> 123
-- *** 协程的挂起 *** --
fun2 = function()
local i = 1
while true do
print(i)
i = i + 1
-- 协程的挂起函数
coroutine.yield(i)
end
end
co3 = coroutine.create(fun2)
-- 默认第一个返回值:协程是否启动成功
-- 第二个返回值为yield中的返回值
isOk, tempI = coroutine.resume(co3) --> 1
print(isOk, tempI) --> true 2
isOk, tempI = coroutine.resume(co3) --> 2
print(isOk, tempI) --> true 3
isOk, tempI = coroutine.resume(co3) --> 3
print(isOk, tempI) --> true 4
-- 这种方式的协程也有返回值,没有 协程是否启动成功 的返回值
co4 = coroutine.wrap(fun2)
print("返回值:" .. co4())
print("返回值:" .. co4())
print("返回值:" .. co4())
--[[
output:
1
返回值:2
2
返回值:3
3
返回值:4
--]]
-- ** 协程的状态 ** --
-- coroutine.status(协程对象)
--[[
dead 结束
suspended 暂停
running 进行中
--]]
print(coroutine.status(co3)) --> suspended
print(coroutine.status(co)) --> dead
-- 该函数能够得到当前正在运行的协程的线程号
coroutine.running()