采取命令行调用最终的编译器可执行程序pascal2c.exe。
1.1 测试用例介绍
1.1.1 hello world
PASCAL-S源程序
program test(input,output);
begin
writeln('h','e','l','l','o',' ','w','o','r','l','d','!');
end.
生成的C程序 ```cpp //Head files
include
include
//Overall constant definiton
//Overall variable definition
//Subprogram declaration void test();
//Main function int main() { test(); return 0; }
//Subprogram definition void test() { printf(“%c%c%c%c%c%c%c%c%c%c%c%c\n”, ‘h’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ‘, ‘w’, ‘o’, ‘r’, ‘l’, ‘d’, ‘!’); }
<a name="aX4ih"></a>
### 1.1.2 最大公因数
- PASCAL-S源程序
```pascal
program example(input,output);
var n,i,x,y:integer;
function gcd(a,b:integer):integer;
begin
if b=0 then gcd:=a
else gcd:=gcd(b,a mod b);
end;
begin
read(n);
for i:= 1 to n do
begin
read(x,y);
writeln(gcd(x,y))
end;
end.
//Overall constant definiton
//Overall variable definition int n; int i; int x; int y;
//Subprogram declaration void example(); int gcd(int a, int b);
//Main function int main() { example(); return 0; }
//Subprogram definition void example() { scanf(“%d”, &n); for(i = 1; i <= n; i++) { scanf(“%d%d”, &x, &y); printf(“%d\n”, gcd(x, y)); } }
int gcd(int a, int b) { if(b == 0) return (a); else return (gcd(b, a % b)); }
<a name="HpW1o"></a>
### 1.1.3 快速排序
- PASCAL-S源程序
```pascal
program quicksort(input,output);
var
n,i:integer;
a:array[0..100000] of integer;
procedure kp(l,r:integer);
var
i,j,mid:integer;
begin
if l>=r then exit;
i:=l;j:=r;mid:=a[(l+r) div 2];
repeat
begin
while a[i]<mid do i:=i+1;
while a[j]>mid do j:=j-1;
if i<=j then
begin
a[0]:=a[i];a[i]:=a[j];a[j]:=a[0];
i:=i+1;j:=j-1;
end
end
until i>j;
kp(l,j);
kp(i,r)
end;
begin
read(n);
for i:=1 to n do
read(a[i]);
kp(1,n);
for i:=1 to n do
write(a[i],' ');
end.
//Overall constant definiton
//Overall variable definition int n; int i; int a[100001];
//Subprogram declaration void quicksort(); void kp(int l, int r);
//Main function int main() { quicksort(); return 0; }
//Subprogram definition void quicksort() { scanf(“%d”, &n); for(i = 1; i <= n; i++) scanf(“%d”, &a[i]); kp(1, n); for(i = 1; i <= n; i++) printf(“%d%c”, a[i], ‘ ‘); }
void kp(int l, int r) { int i; int j; int mid; if(l >= r) return; i = l; j = r; mid = a[(l + r) / 2]; do { while(a[i] < mid) i = i + 1; while(a[j] > mid) j = j - 1; if(i <= j) { a[0] = a[i]; a[i] = a[j]; a[j] = a[0]; i = i + 1; j = j - 1; } } while(!(i > j)); kp(l, j); kp(i, r); }
<a name="MX0l2"></a>
### 1.1.4 语义错误PASCAL-S源程序
```pascal
program read(write,output);//主程序名和库程序同名
const m=10;
exit=m;//exit是库函数
var a,x,y:integer;
b:real;
c:char;
d:boolean;
e:array[1..5] of integer;
function fun1:integer;//报函数没有返回语句的警告
begin
v:=a;//v未定义
end;
function fun2:integer;
begin
fun2:=1;
fun2:=e[6];//数组下标越界
fun2;//函数不能作为一条单独的语句
a:=fun2[1];//错把函数名当做数组
end;
procedure pro1(a:integer;b:real);
var c:real;
d:integer;
begin
c:=a+b;//integer可以隐式转换为real
d:=a+b;//real不能隐式转换为integer
end;
procedure pro2(var a:real;b:integer);
begin
exit(a+b);//过程没有返回值
end;
begin
a:=1;
m:=a;//常量赋值语句右值不为常量
b:=2;
c:=3;//赋值语句左右类型不匹配
d:=a>b;//d为false
if a then b:=b+1;//if条件表达式不为boolean
repeat a:=a+c until not d;//a:=a+c语句左右类型不匹配
for b:=10 to 1 do e(a,a);//循环变量不可以是real;错把数组名当做函数
while a<10 do a:=a+1;
x:=pro1(x,y);//pro1为过程,没有返回值
x:=1;
y:=2;
pro1(x,y);//传值参数支持integer到real的隐式转换
pro2(x,y);//pro2的第一个参数为传引用,integer无法隐式转换为real
pro1(x+y);//pro1有两个参数
pro1(x+y,x+y);//传值参数支持复合表达式
pro2(x+y);//pro2有两个参数
pro2(x+y,x+y);//pro2第一个参数为引用参数,只能是变量或者数组元素,不能是复杂表达式
end.
1.1.5 词法+语法错误PASCAL-S源程序
主程序参数列表缺少右括号,出现非法字符#10次
program test(input,output;
#
#
#
#
#
#
#
#
#
#
begin
end.
1.2 无参调用
- 测试命令
pascal2c
- 测试用例
1.1 hello world,保存在PascalProgram.pas中
- 预期结果
从默认输入文件PascalProgram.pas中读取PASCAL-S源程序,且编译无错误,输出C程序保存到CProgram.c中
- 控制台输出
- 结果分析
编译无错误,C代码成功保存到默认输出文件CProgram.c中
1.3 命令行参数测试
1.3.1 -inname 输入文件名
测试命令
pascal2c –inname gcd.pas
测试用例
1.2 最大公因数,保存在gcd.pas中
- 预期结果
从gcd.pas中读取PASCAL-S源程序,且编译无错误,生成的C代码保存到默认文件CProgram.c中
控制台输出
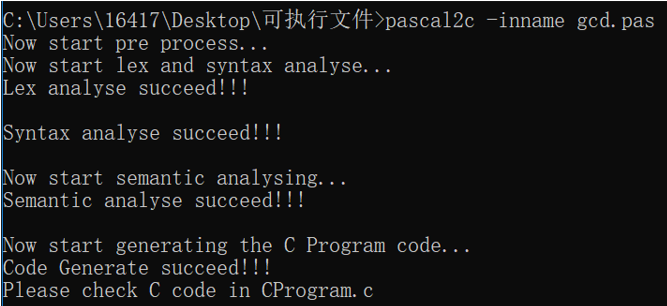<br />
结果分析
编译无错误,成功编译了来自指定输入文件gcd.pas的PASCAL-S源程序,并成功生成C代码
1.3.2 -outname 输出文件名
- 测试命令
pascal2c -inname gcd.pas -outname gcd.out
测试用例
1.2最大公因数,保存在gcd.pas中
预期结果
编译成功,从gcd.pas中读取PASCAL-S源程序,生成的C代码保存到gcd.out中
控制台输出
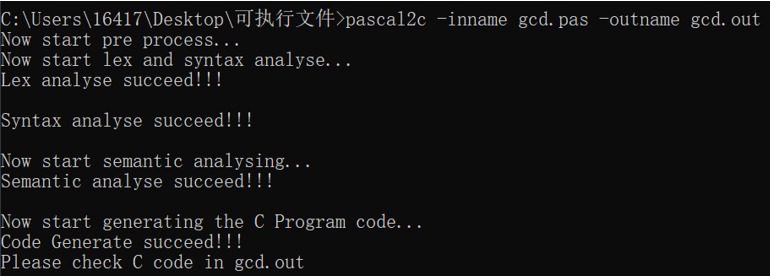
结果分析
编译成功,成功地从gcd.pas中读取PASCAL-S源程序,生成的C代码保存到了gcd.out中<br />
1.3.3 -compiler 编译器名
测试命令
pascal2c –compiler gcc
- 测试用例
1.1 hello world,保存在PascalProgram.pas中
- 预期结果
编译成功,从默认输入中读取PASCAL-S源程序,保存到默认输出中, 并调用gcc编译器编译C程序,生成可执行文件,为默认名称CProcess.exe
- 控制台输出
- 结果分析
编译成功,从默认输入中读取PASCAL-S源程序,成功生成C代码,保存到默认输出中,调用gcc编译C程序,生成的可执行文件,取名为默认名称CProcess.exe
1.3.4 -exename 可执行文件名
- 测试命令
hellpascal2c -exename helloworld
测试用例
1.1 hello world,保存在PascalProgram.pas中
预期结果
编译成功,从默认输入中读取PASCAL-S源程序,成功生成C代码,保存到默认输出中,调用gcc编译C程序,生成的可执行文件,取名为指定名称helloworld.exe
控制台输出
结果分析
编译成功,从默认输入中读取PASCAL-S源程序,成功生成C代码,保存到默认输出中,调用gcc编译C程序,生成的可执行文件,取名为指定名称helloworld.exe<br />
1.3.5 -execute 执行
测试命令
pascal2c -execute
测试用例
1.1 hello world,保存在PascalProgram.pas中
预期结果
编译成功,从默认输入中读取PASCAL-S源程序,成功生成C代码,保存到默认输出中,调用gcc编译C程序,生成的可执行文件,取名为默认名称CProcess.exe,并自动执行,输出hello world
控制台输出
结果分析
编译成功,从默认输入中读取PASCAL-S源程序,成功生成C代码,保存到默认输出中,调用gcc编译C程序,生成的可执行文件,取名为默认名称CProcess.exe,并自动执行,输出hello world<br />
1.3.6 -errorbound 错误上限
测试命令
pascal2c –errorbound 5
- 测试用例
1.4 语义错误,保存在默认输入PascalProgram.pas中
- 预期结果
达到5个语义错误后,编译器停止运行,并输出报错信息
- 控制台输出
- 结果分析
1.3.7 -developer 开发者信息
测试命令
pascal2c -developer
控制台输出
1.3.8 -version 版本信息
- 测试命令
pascal2c -version
- 控制台输出
1.3.9 -help 帮助文档
- 测试命令
pascal2c -help
- 控制台输出
1.4 综合测试
1.4.1 快速排序
- 测试命令
pascal2c -inname quicksort.pas -exename quicksort -outname quicksort.c -execute
预期结果
编译成功,从quicksort.pas中读取快速排序的PASCAL-S源程序,生成的C代码保存到quicksort.c中,编译C程序,生成可执行程序,名为quicksort.exe,自动执行可执行程序,输入样例,输出正确排序结果<br />
控制台输出及运行结果
- 结果分析
编译成功,从quicksort.pas中读取快速排序的PASCAL-S源程序,生成的C代码保存到quicksort.c中,编译C程序,生成可执行程序,名为quicksort.exe,自动执行可执行程序,输入样例
5
2 5 4 1 3
输出正确排序结果
1 2 3 4 5
1.4.2 词法+语法错误
- 测试命令
pascal2c –outname lex_and_yacc_error.c –errorbound 6
- 预期结果
报词法、语法错误,错误个数被限制在6个,未生成输出文件lex_and_yacc_error.c
- 控制台输出及运行结果
- 结果分析
报词法、语法错误,错误个数被限制在6个,未生成输出文件lex_and_yacc_error.c