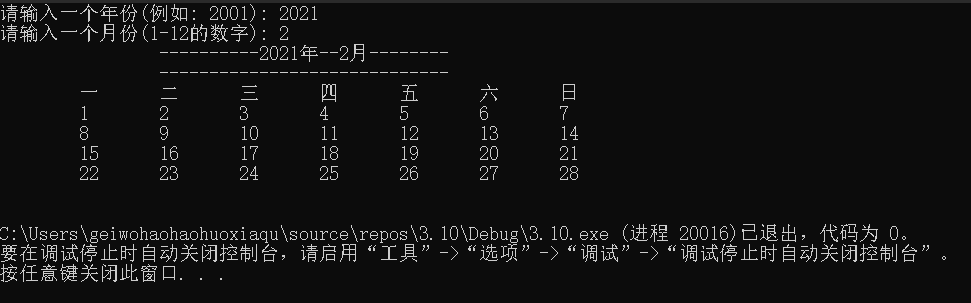
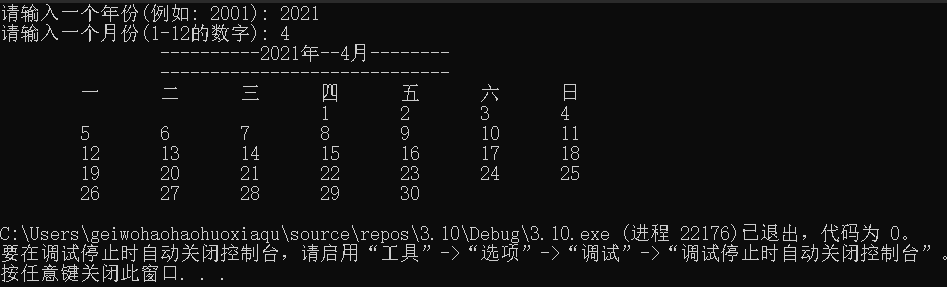
#include <stdio.h>
#include<stdbool.h>
//声明所有用到的函数
void printMonth(int, int);
void printMonthTitle(int, int);
void printMonthBody(int, int);
int getStartDay(int, int);
int getTotalNumberOfDays(int, int);
int getNumberOfDaysInMonth(int, int);
bool isLeapYear(int);
int main() {
int year, month;
printf("请输入一个年份(例如: 2001): ");
scanf_s("%d", &year);
fflush(stdin);
printf("请输入一个月份(1-12的数字): ");
scanf_s("%d", &month);
fflush(stdin);
// 调用printMonth函数打印相应年份和月份的日历
printMonth(year, month);
return 0;
}
/** 打印相应年份和月份的日历 */
void printMonth(int year, int month) {
// 打印日历的开头
printMonthTitle(year, month);
// 打印日历内容
printMonthBody(year, month);
}
/** 打印月份的开头*/
void printMonthTitle(int year, int month) {
// 填写代码打印月份的开头
printf("\t\t----------%d年--%d月--------",year,month);
printf("\n");
printf("\t\t-----------------------------");
printf("\n");
printf("\t一\t二\t三\t四\t五\t六\t日");
printf("\n");
}
/** 打印日历内容 */
void printMonthBody(int year, int month) {
// 调用getStartDay函数获得每月第一天是星期几
int xqj;
int ts;
int x,j;
j = 1;
xqj = getStartDay(year, month);
// 调用getNumberOfDaysInMonth函数获得某个月有几天?
ts = getNumberOfDaysInMonth(year, month);
// 在当月的第一天前面加上若干空格
for (x = 1; x < xqj; x++)
printf("\t ");
for (int i = 0; i < 5; i++)
{
for (x; x <= 7; x++) {
if (j <= ts) {
printf("\t%d", j++);
}
}
printf("\n");
x = 1;
}
}
/** 获得某月第一天是星期几 */
int getStartDay(int year, int month) {
const int START_DAY_FOR_JAN_1_1800 = 3;
// 获得从 1800年1月1日 至 year年month月1日之间的天数
int xq;
xq = getTotalNumberOfDays(year, month);
// 返回year年month月1日是星期几
xq += 3 ;
return xq%7;
}
/** 获得从 1800年1月1日 至 year年month月1日之间的天数 */
int getTotalNumberOfDays(int year, int month) {
// 获得从 1800年1月1日 至 year年month月1日之间的天数
int tgtn;
int ksy;
ksy = 1800;
tgtn = 0;
for (ksy; ksy < year; ksy++) {
if (isLeapYear(ksy)) {
tgtn += 366;
}
else
tgtn += 365;
}
// 计算输入年份的1月1日至输入月份的第一天之间的日期数
for (month - 1; month - 1 > 0; month--) {
tgtn += getNumberOfDaysInMonth(year, month-1);
}
return tgtn;
}
/** 获得每个月的天数 */
int getNumberOfDaysInMonth(int year, int month) {
if (month == 1|| month==3 || month==5 || month==7 || month==8 || month==10 || month==12)
return 31;
if (month == 2)
{
if (isLeapYear(year))
{
return 29;
}
else
return 28;
}
if (month == 4 || month == 6 || month == 9 || month == 11)
return 30;
}
/** 判断是否是闰年 */
bool isLeapYear(int year) {
if (year % 100 == 0) {
if ((year % 400 == 0))
return 1;
else
return 0;
}
else {
if ((year % 4 == 0))
return 1;
else
return 0;
}
}