package edu.mama.ls15.homework;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Homework {
/**
* 第一题:count个人抢总额为amount的拼手气红包
*
* 要求:
* 1、通过程序限制,每人最多能抢200元
* 2、通过程序限制,最多允许10人参与抢红包
* 3、红包金额单位为元,保留2位小数
* 4、打印每个人红包的金额
* 5、打印手气最佳者的序号和金额
*
* @param amount 拼手气红包总金额
* @param count 抢红包的人数
*/
public static void redEnvelope(BigDecimal amount, int count) {
//TODO 这里写下你的代码
final BigDecimal MIN = new BigDecimal("0.01");
final BigDecimal MAX = new BigDecimal("200");
if (count <= 0 || count > 10) {
throw new IllegalArgumentException("只允许1-10人参与抢红包");
}
if (amount == null
|| amount.compareTo(MIN.multiply(new BigDecimal(count))) < 0
|| amount.compareTo(MAX.multiply(new BigDecimal(count))) > 0) {
throw new IllegalArgumentException("每人最少能抢0.01元,最多200元");
}
List<BigDecimal> amounts = new ArrayList<>();
for (int i = count; i > 1; i--) {
//计算经过本次抢红包后最多允许剩下的钱数
BigDecimal maxRemind = new BigDecimal(i - 1).multiply(MAX);
//计算经过本次抢红包后最少允许剩下的钱数
BigDecimal minRemind = new BigDecimal(i - 1).multiply(MIN);
//本次抢红包金额的最小值
BigDecimal minAmount = MIN.max(amount.subtract(maxRemind));
BigDecimal maxAmount = MAX.min(amount.subtract(minRemind));
BigDecimal curAmount = maxAmount.subtract(minAmount)
.multiply(BigDecimal.valueOf(Math.random()))
.add(minAmount)
.setScale(2, RoundingMode.HALF_UP);
amounts.add(curAmount);
amount = amount.subtract(curAmount);
}
//最后一个人抢红包不需要随机,剩下多少全是他的
amounts.add(amount);
BigDecimal maxAmount = BigDecimal.ZERO;
int maxIndex = 0;
for (int i = 0; i < amounts.size(); i++) {
BigDecimal amt = amounts.get(i);
System.out.printf("第%d个人抢到:%s\n", i, amt);
if (maxAmount.compareTo(amt) < 0) {
maxAmount = amt;
maxIndex = i;
}
}
System.out.printf("第%d号为手气最佳,抢得%s\n", maxIndex, maxAmount);
}
/**
* 第二题:断点续读
* 要求:上次已经从filePath种读取了一部分内容lastRead,但并没有全部读取完成,继续读取文件中的剩余内容并返回
* 提示:查看API
*
* @param filePath 文件路径
* @param lastRead 上次读取到的内容
*/
public static String continueRead(String filePath, String lastRead) {
//TODO 这里写下你的代码
String str = "";
//使用try(resource)用法,自动关闭流
try (Reader input = new FileReader(filePath, Charset.forName("UTF-8"))) {
//跳过上次已读取的内容,从未读取的位置开始读取
input.skip(lastRead.length());
//定义数据缓存去
int bufferSize = 100;
char[] chars = new char[bufferSize];
int count = input.read(chars);
//每次读入100个字符,循环读入
while (count > 0) {
char[] contents = Arrays.copyOf(chars, count);
//将本次读到的内容拼接到字符串例
str += new String(contents);
//本次读入的数据处理完成,尝试再次读入100字符,返回实际读入字符数
count = input.read(chars);
}
} catch (IOException e) {
e.printStackTrace();
}
return str;
}
public static void main(String[] args) {
//第一题
//TODO 这里写下你的代码
try {
redEnvelope(new BigDecimal("1"), 10);
} catch (Exception e) {
System.out.println(e.getMessage());
}
//第二题
String other = continueRead("LS15/res/晖哥语录.txt", "人性无所谓善与恶");
System.out.println(other);
}
}
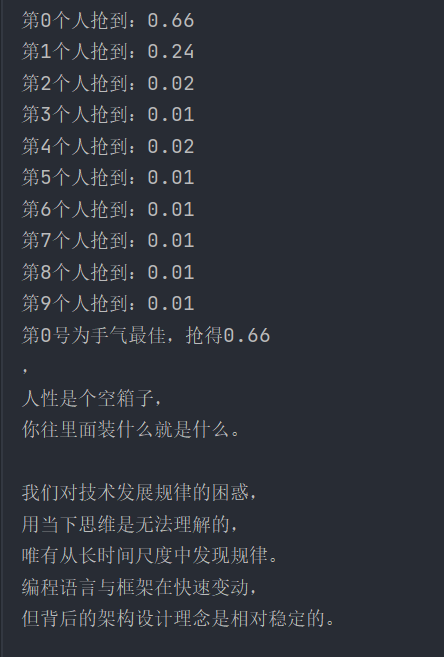