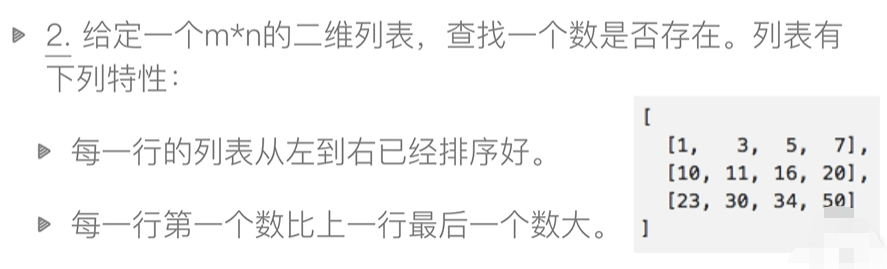
package com.algorithm.demo.searchsorts;
/**
* @Author leijs
* @date 2022/4/15
*/
public class Demo2 {
public static void main(String[] args) {
int[][] array = new int[][]{{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
System.out.println(searchMatrix(array, 6));
}
public static boolean searchMatrix(int[][] array, int target) {
int row = array.length;
if (row == 0) {
return false;
}
int column = array[0].length;
int left = 0;
int right = row * column - 1;
while (left < right) {
int mid = (left + right) / 2;
// mid = 7
// 1 2 3 4
// 5 6 7 8
// i是第几行 index / colunm
// j是第几列 index %colunm
int i = mid / column;
int j = mid % column;
if (array[i][j] == target) {
return true;
}
if (array[i][j] < target) {
left = mid + 1;
}
if (array[i][j] > target) {
right = mid - 1;
}
}
return false;
}
}