原始数据类型包括
- boolean
- null
- undefined
- number
- string
- symbol //es6 新的一种数据类型
- bigint
引用数据类型
object 包括以下
- Object 普通对象
- Array 数组对象
- RegExp 正则对象
- Date 日期对象
- Math 算数对象
- Function 函数对象
数据类型检测
typeof 对于原始数据除了null (null是一个历史遗留问题 显示object) 其他都可以正确检测 对于引用数据类型(除了函数类型)都显示object
数据类型之间的转换
Javascript中类型转换只有以下三种
转换成数字
Number('abc') //NaN
Number({a:1}) // NaN
Number('123') // 123
转换成布尔值 ```javascript Boolean(0) false Boolean(1) true Boolean(‘’) false Boolean(‘123’) true
- 转换成字符串
| 原始值 | 转换目标 | 说明 |
| --- | --- | --- |
| number | boolean | 除了0 -0 NaN 其他都为true |
| string | boolean | 除了空字符串其他都为true |
| undefined null | boolean | 都是false |
| 引用类型 | boolean | 都为true |
| number | string | 5=> '5' |
| Boolean、函数、Symbol | string | 'true' |
| 数组 | string | [1,2] => '1,2' |
| 对象 | string | '[Object Object]' |
且对象类型只有null转换为Boolean为false<br />== 和 ===<br />==不像===那样严格,对于一般情况,只要值相等,就返回true,但==还涉及一些类型转换,它的转换规则如下:
- 两边的类型是否相同,相同的话就比较值的大小,例如1==2,返回false
- 判断的是否是null和undefined,是的话就返回true
- 判断的类型是否是String和Number,是的话,把String类型转换成Number,再进行比较
- 判断其中一方是否是Boolean,是的话就把Boolean转换成Number,再进行比较
- 如果其中一方为Object,且另一方为String、Number或者Symbol,会将Object转换成字符串,再进行比较
对象转原始类型,会调用内置的[ToPrimitive]函数,对于该函数而言,其逻辑如下:
- 如果Symbol.toPrimitive()方法,优先调用再返回
- 调用valueOf(),如果转换为原始类型,则返回
- 调用toString(),如果转换为原始类型,则返回
- 如果都没有返回原始类型,会报错
<br />
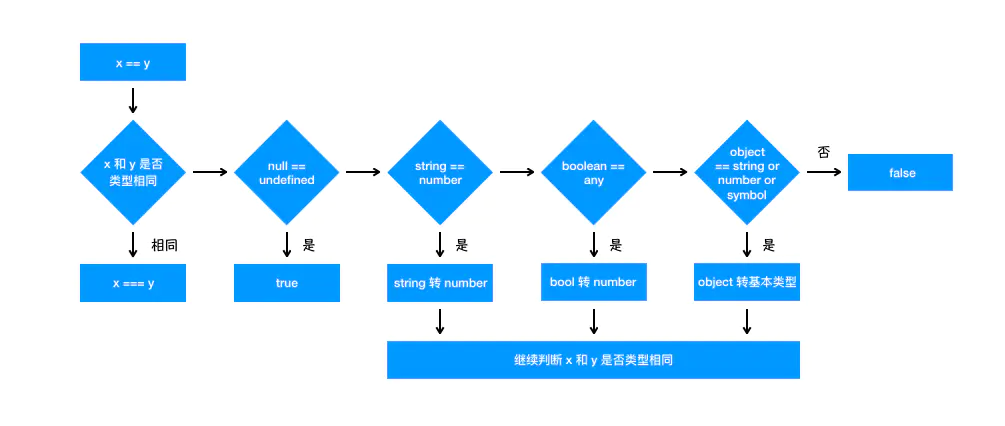
如何让if(a == 1 && a == 2)条件成立
```javascript
var a = {
value: 0,
valueOf: function() {
this.value++;
return this.value;
}
};
console.log(a == 1 && a == 2);//true
一个简单验证数据类型的函数
function isWho(x) {
// null
if (x === null) return 'null'
const primitive = ['number', 'string', 'undefined',
'symbol', 'bigint', 'boolean', 'function'
]
let type = typeof x
//原始类型以及函数
if (primitive.includes(type)) return type
//对象类型
if (Array.isArray(x)) return 'array'
if (Object.prototype.toString.call(x) === '[object Object]') return 'object'
if (x.hasOwnProperty('constructor')) return x.constructor.name
const proto = Object.getPrototypeOf(x)
if (proto) return proto.constructor.name
// 无法判断
return "can't get this type"
}
一些手写的数据类型判断
const _array = [1, 2]
// 判断是否是数组
console.log(_array instanceof Array)
console.log(Array.isArray(_array))
console.log(Object.prototype.toString.call(_array) === '[object Array]')
console.log(_array.constructor === Array)
const _object = { a: 1 }
//判断是否是对象
console.log(_object instanceof Object)
console.log(_object.constructor === Object)
console.log(Object.prototype.toString.call(_object) === '[object Object]')
const _function = function () { }
// 判断是否是function
console.log(typeof (_function) === 'function')
console.log(_function instanceof Function)
console.log(_function.constructor === Function)
console.log(Object.prototype.toString.call(_function) === '[object Function]')
const _null = null
//判断是否是null
console.log(null === null)