//EmpReader.java
package edu.hue.jk.io;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.sql.Date;
import java.util.Collection;
import java.util.HashMap;
import edu.hue.jk.bean.Emp;
public class EmpReader {
public HashMap<Integer, Emp> read(File inFile) {
HashMap<Integer, Emp> empMap = new HashMap<>();
BufferedReader reader = null;
String line;
try {
reader = new BufferedReader(new FileReader(inFile));
reader.readLine(); //跳过第一行
String[] items;
Emp emp;
while ((line = reader.readLine()) != null) {
//使用item=line.split("\t");将读入的数据行按照制表符切割成一组字符串数组
items = line.split("\t");
Integer empno = Integer.parseInt(items[0]);
String ename = items[1];
String job = items[2];
Integer mgr = items[3].equals("") ? null : Integer.parseInt(items[3]);
Date hiredate = Date.valueOf(items[4]);
Double sal = items[5].equals("") ? null : Double.parseDouble(items[5]);
Double comm = items[6].equals("") ? null : Double.parseDouble(items[6]);
Integer deptno = items[7].equals("") ? null : Integer.parseInt(items[7]);
emp = new Emp(empno, ename, job, mgr, hiredate, sal, comm, deptno);
empMap.put(empno, emp);
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
return empMap;
}
public static void main(String[] args) {
File inFile = new File("etc/emp.txt");
EmpReader reader = new EmpReader();
HashMap<Integer, Emp> empMap = reader.read(inFile);
Collection<Emp> empSet = empMap.values();
for (Emp e : empSet) {
System.out.println(e);
}
}
}
//DeptReader.java
package edu.hue.jk.io;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.Collection;
import java.util.HashMap;
import edu.hue.jk.bean.Dept;
public class DeptReader {
public HashMap<Integer, Dept> read(File inFile) {
HashMap<Integer, Dept> deptMap = new HashMap<>();
BufferedReader reader = null;
String line;
try {
reader = new BufferedReader(new FileReader(inFile));
reader.readLine();
String[] items;
Dept dept;
while ((line = reader.readLine()) != null) {
items = line.split("\t");
Integer deptno = Integer.parseInt(items[0]);
String dname = items[1];
String loc = items[2];
dept = new Dept(deptno, dname, loc);
deptMap.put(deptno, dept);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return deptMap;
}
public static void main(String[] args) {
File inFile = new File("etc/dept.txt");
DeptReader reader = new DeptReader();
HashMap<Integer, Dept> deptMap = reader.read(inFile);
Collection<Dept> deptSet = deptMap.values();
for (Dept e : deptSet) {
System.out.println(e);
}
}
}
//MainApp.java
package edu.hue.jk.dao;
import java.io.File;
import java.sql.Date;
import java.util.HashMap;
import edu.hue.jk.bean.Dept;
import edu.hue.jk.bean.Emp;
import edu.hue.jk.io.DeptReader;
import edu.hue.jk.io.EmpReader;
public class MainApp {
public static void main(String[] args) {
File empinFile = new File("etc/emp.txt");
EmpReader empreader = new EmpReader();
HashMap<Integer, Emp> empMap = empreader.read(empinFile);
File deptinFile = new File("etc/dept.txt");
DeptReader deptreader = new DeptReader();
HashMap<Integer, Dept> deptMap = deptreader.read(deptinFile);
System.out.println("DEPT_NAME\tEMPLPYEE");
for (Emp e : empMap.values()) {
if (e.getComm()==null &&
(e.getSal()<2000 ||
e.getHiredate().compareTo(Date.valueOf("1981-05-01"))>=0 &&
e.getHiredate().compareTo(Date.valueOf("1981-11-30"))<=0)) {
Integer deptno = e.getDeptno();
Dept dept = deptMap.get(deptno);
System.out.println(dept.getDname() + "\t" + e.getEname());
}
}
}
}
//EmpDeptVO.java
package edu.hue.jk.dao;
public class EmpDeptVO implements Comparable<EmpDeptVO> {
private String deptt;
private Integer count;
public EmpDeptVO(String deptt, Integer count) {
super();
this.deptt = deptt;
this.count = count;
}
public String getDeptt() {
return deptt;
}
public void setDeptt(String deptt) {
this.deptt = deptt;
}
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
@Override
public String toString() {
return "EmpDeptVO [deptt=" + deptt + ", count=" + count + "]";
}
@Override
public int compareTo(EmpDeptVO o) {
// this为当前对象,o为目标对象,比较两者count,实现降序排列
return (this.getCount()-o.getCount()) * -1;
}
}
//MainApp2.java
package edu.hue.jk.dao;
import java.io.File;
import java.sql.Date;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import edu.hue.jk.bean.Dept;
import edu.hue.jk.bean.Emp;
import edu.hue.jk.io.DeptReader;
import edu.hue.jk.io.EmpReader;
public class MainApp2 {
public static void main(String[] args) {
File empinFile = new File("etc/emp.txt");
EmpReader empreader = new EmpReader();
HashMap<Integer, Emp> empMap = empreader.read(empinFile);
File deptinFile = new File("etc/dept.txt");
DeptReader deptreader = new DeptReader();
HashMap<Integer, Dept> deptMap = deptreader.read(deptinFile);
HashMap<String, List<Emp>> data = new HashMap<>();
for (Emp emp : empMap.values()) {
if (emp.getComm()==null && (emp.getSal()<2000 || emp.getHiredate().compareTo(Date.valueOf("1981-05-01"))>=0 && emp.getHiredate().compareTo(Date.valueOf("1981-11-30"))<=0)) {
Integer deptno = emp.getDeptno();
Dept dept = deptMap.get(deptno);
if (data.containsKey(dept.getDname())) {
List<Emp> empList = data.get(dept.getDname());
empList.add(emp);
} else {
List<Emp> empList = new ArrayList<Emp>();
empList.add(emp);
data.put(dept.getDname(), empList);
}
}
}
List<EmpDeptVO> voList = new ArrayList<EmpDeptVO>();
for (String Dept : data.keySet()) {
voList.add(new EmpDeptVO(Dept, data.get(Dept).size()));
}
Object[] outList = voList.toArray();
Arrays.sort(outList);
System.out.println("DEPT_NAME" + "\t" + "total");
for (Object vo : outList) {
System.out.println(((EmpDeptVO)vo).getDeptt() + "\t" + ((EmpDeptVO)vo).getCount());
}
}
}
//emp.txt
EMPNO ENAME JOB MGR HIREDATE SAL COMM DEPTNO
7369 SMITH CLERK 7902 1980-12-17 800 20
7499 ALLEN SALESMAN 7698 1981-02-20 1600 300 30
7521 WARD SALESMAN 7698 1981-02-22 1250 500 30
7566 JONES MANAGER 7839 1981-04-02 2975 20
7654 MARTIN SALESMAN 7698 1981-09-28 1250 1400 30
7698 BLAKE MANAGER 7839 1981-05-01 2850 30
7782 CLARK MANAGER 7839 1981-06-09 2450 10
7839 KING PRESIDENT 1981-11-17 5000 10
7844 TURNER SALESMAN 7698 1981-09-08 1500 0 30
7900 JAMES CLERK 7698 1981-12-03 950 30
7902 FORD ANALYST 7566 1981-12-03 3000 20
7934 MILLER CLERK 7782 1982-01-23 1300 10
//DEPT.txt
DEPTNO DNAME LOC
10 ACCOUNTING NEW YORK
20 RESEARCH DALLAS
30 SALES CHICAGO
40 OPERATIONS BOSTON
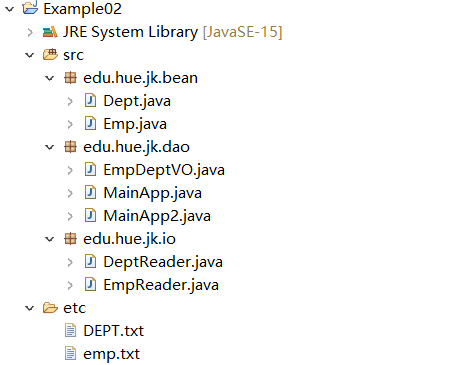