第一步:初始化项目
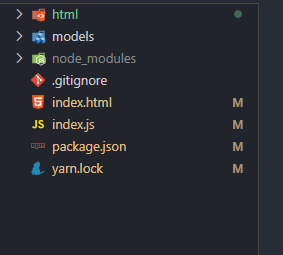
第二步:建立与本地数据库的连接
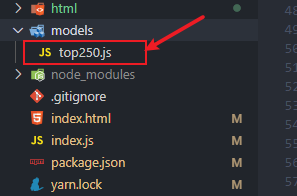
top250.js:
const mongoose = require("mongoose");
//1.连接本地数据库
mongoose.connect( 'mongodb://127.0.0.1:27017/movies', {useNewUrlParser: true});
//2.在本地定义一个Schema和远程数据库的字段映射
var Top250Schema = new mongoose.Schema({
name:String,
rating:Number
});
// 3.创建数据模型,操作数据库
var Top250Model = mongoose.model("top250",Top250Schema); //是我们获取的数据库里Top250的那张表
module.exports = Top250Model;
第三步:创建服务器路由
Tips:post需要使用到中间件koa-bodyparser,还需要注意使用跨域中间件koa2-cors。
const koa = require("koa");
const router = require("koa-router")();
const Top250Model = require("./models/top250");
const cors = require("koa2-cors");
const bodyParser = require("koa-bodyparser");
const app = new koa();
//获取数据库列表数据
router.get("/top250",async ctx=>{
var data = await Top250Model.find({})
ctx.body = data
})
app.use(cors());
app.use(bodyParser());
app.use(router.routes());
app.listen(8080);
第四步:前端获取路由数据
<div class="" id="app">
<table class="table table-hover">
<thead>
<tr>
<th>编号</th>
<th>电影名</th>
<th>评分</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="item in movies" :key="item.name">
<td>{{item._id}}</td>
<td>{{item.name}}</td>
<td>{{item.rating}}</td>
<td>
<button type="button" class="btn btn-danger" @click="doDelete(item._id)">delete</button>
<button type="button" class="btn btn-warning" @click="doUpdate()">update</button>
</td>
</tr>
</tbody>
</table>
</div>
<script>
new Vue({
el:"#app",
data:{
movies:[]
},
mounted() {
this.request();
},
methods: {
//请求数据
request(){
$.ajax({
type: "get",
url: "http://localhost:8080/top250",
dataType: 'json'
}).then(res=>{
this.movies = res;
});
},
},
})
</script>