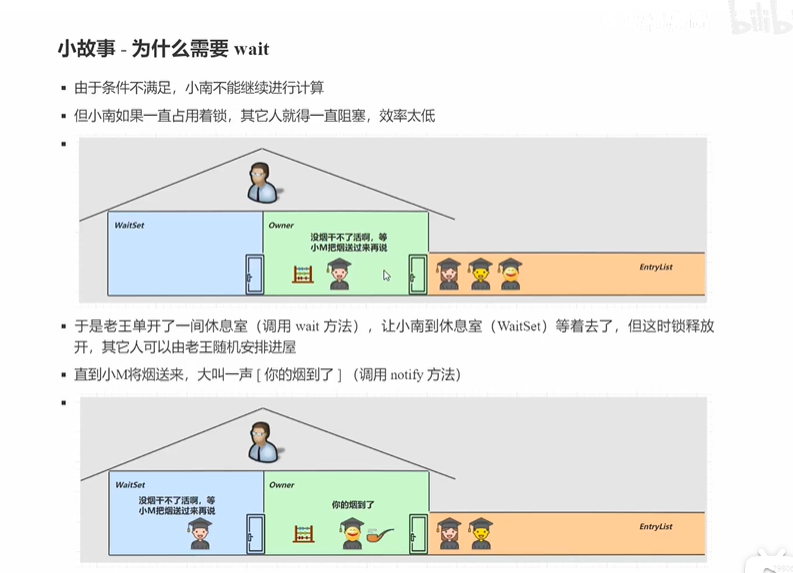
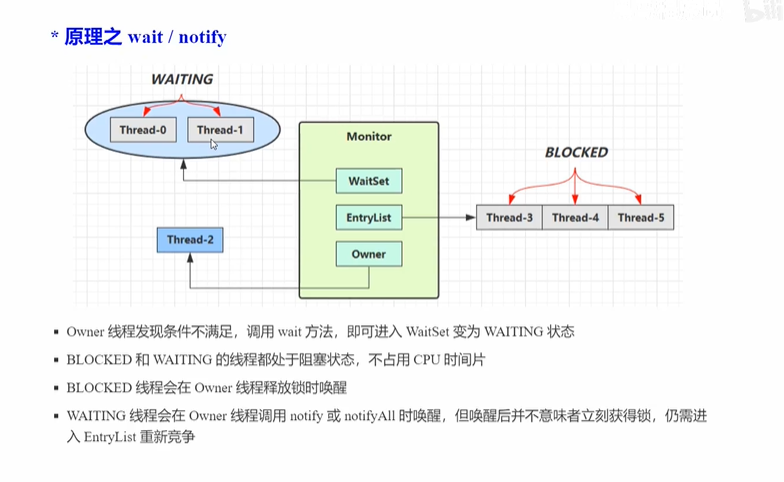
@Slf4j(topic = "c.TestWaitNotify")
public class TestWaitNotify {
static final Object lock = new Object();
public static void main(String[] args) throws InterruptedException {
new Thread(()->{
log.info("执行。。。。");
synchronized (lock){
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"t1").start();
new Thread(()->{
log.info("执行。。。");
synchronized (lock){
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"t2").start();
TimeUnit.SECONDS.sleep(2);
log.info("main thread 唤醒其他线程");
synchronized (lock){
lock.notify();// 唤醒t1和t2线程中其中一个
// lock.notifyAll();// 唤醒所有synchronized wait 的线程
}
}
}
@Slf4j
public class TestCorrectPostureStep1 {
static final Object room = new Object();
static boolean hasCigarette = false;
static boolean hasTakeout = false;
public static void main(String[] args) {
new Thread(()->{
synchronized (room){
if (!hasCigarette){
try {
log.info("没有烟,我要等一会才能工作");
room.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
if (hasCigarette){
log.debug("有烟,可以干活了");
}
}
},"t1").start();
for (int i = 0;i<5;i++){
new Thread(()->{
synchronized (room){
try {
Thread.sleep(2_000);
} catch (InterruptedException e) {
e.printStackTrace();
}
log.debug("开始干活了");
}
},"其他人"+i).start();
}
try {
Thread.sleep(1_000);
} catch (InterruptedException e) {
e.printStackTrace();
}
new Thread(()->{
synchronized (room) {
hasCigarette = true;
room.notify();
}
},"松烟的").start();
}
}
虚假唤醒
package org.example.jvm;
import lombok.extern.slf4j.Slf4j;
import java.util.concurrent.TimeUnit;
/**
* @author huskyui
*/
@Slf4j
public class TestCorrectPostureStep3 {
final static Object lock = new Object();
static boolean hasCigarette = false;
static boolean hasTakeout = false;
public static void main(String[] args) throws InterruptedException {
new Thread(()->{
synchronized (lock){
log.info("有外卖吗 {}",hasTakeout);
try{
lock.wait();
}catch (Exception e){
e.printStackTrace();
}
log.info("有外卖没 {}",hasTakeout);
if (hasTakeout){
log.debug("可以干活了。有外卖了");
}else{
log.info("没干成活。。。");
}
}
},"小女").start();
new Thread(()->{
synchronized (lock){
log.info("有烟吗 {}",hasCigarette);
try{
lock.wait();
}catch (Exception e){
e.printStackTrace();
}
log.info("有烟没 {}",hasCigarette);
if (hasCigarette){
log.debug("可以干活了。有烟了");
}else{
log.info("没干成活。。。");
}
}
},"小南").start();
TimeUnit.SECONDS.sleep(2);
new Thread(()->{
synchronized (lock){
hasCigarette = true;
lock.notifyAll();
}
}).start();
}
public final void dos(){
}
}
使用while,可以防止虚假唤醒
package org.example.jvm;
import lombok.extern.slf4j.Slf4j;
import java.util.concurrent.TimeUnit;
/**
* @author huskyui
*/
@Slf4j
public class TestCorrectPostureStep3 {
final static Object lock = new Object();
static boolean hasCigarette = false;
static boolean hasTakeout = false;
public static void main(String[] args) throws InterruptedException {
new Thread(() -> {
synchronized (lock) {
log.info("有外卖吗 {}", hasTakeout);
while (!hasTakeout) {
log.info("没有外卖先歇会");
try {
lock.wait();
} catch (Exception e) {
e.printStackTrace();
}
}
log.info("有外卖没 {}", hasTakeout);
if (hasTakeout) {
log.debug("可以干活了。有外卖了");
} else {
log.info("没干成活。。。");
}
}
}, "小女").start();
new Thread(() -> {
synchronized (lock) {
log.info("有烟吗 {}", hasCigarette);
while (!hasCigarette) {
log.info("没烟先歇会");
try {
lock.wait();
} catch (Exception e) {
e.printStackTrace();
}
}
log.info("有烟没 {}", hasCigarette);
if (hasCigarette) {
log.debug("可以干活了。有烟了");
} else {
log.info("没干成活。。。");
}
}
}, "小南").start();
TimeUnit.SECONDS.sleep(2);
new Thread(() -> {
synchronized (lock) {
hasCigarette = true;
lock.notifyAll();
}
}).start();
}
}
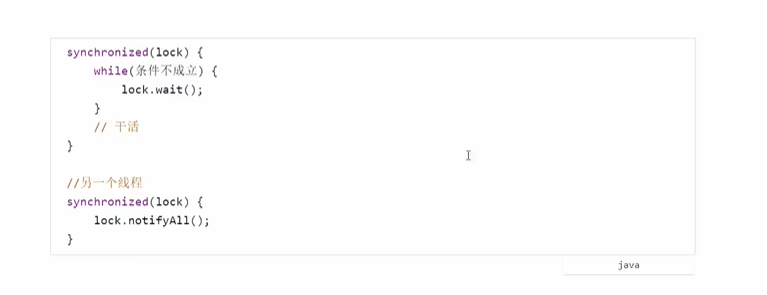